This is a python programming question Python Code: class Node: def __init__(self, initial_data): self.data = initial_data self.next = None class LinkedList: def __init__(self):
This is a python programming question
Python Code:
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def prepend(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head = new_node
def insert_after(self, current_node, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
elif current_node is self.tail:
self.tail.next = new_node
self.tail = new_node
else:
new_node.next = current_node.next
current_node.next = new_node
def remove_after(self, current_node):
if (current_node == None) and (self.head != None):
succeeding_node = self.head.next
self.head = succeeding_node
if succeeding_node == None: # Remove last item
self.tail = None
elif current_node.next != None:
succeeding_node = current_node.next.next
current_node.next = succeeding_node
if succeeding_node == None: # Remove tail
self.tail = current_node
def display(self):
node = self.head
if (node == None):
print('Empty!')
while node != None:
print(node.data, end=' ')
node = node.next
def remove_smallest_node(self):
#*** Your code goes here!***
# NOTE: this function will print out the information about the smallest node
# before removing it
def Generate_List_of_LinkedLists(n):
LLL = []
#*** Your code goes here!***
# NOTE: this function will read the input from the user for each of the lists and
# generate the list
return LLL
if __name__ == '__main__':
#*** Your code goes here!***
****Note****
This is how the output should be, given example input.
Example -
For Input
2 1 3 5 7 -1 3 4 7 2 9 -1 1
Expected Output
linked list [ 0 ]:
1 3 5 7
linked list [1]:
3 4 7 2 9
the smallest node is:
2
List 1 after removing smallest node:
3 4 7 9
![In this lab you are asked to complete the provided code so that it generates a list of singly-linked-lists (note: this will be a python list, where
each element of the list is a singly linked list). Your code should do the following:
• For the linked list in the index position supplied by the user, your code should find the node with the smallest value and delete it
• Your code should display "Empty!", if there is no nodes left in the linked list after removing the smallest node!
• Finally, it should print the modified linked list
For example, when the input is:
2
1
7
• To generate a list of singly linked lists, your code should accept the number of linked lists as an input
• Then, for each linked list, it should accept integers as nodes till the user enters -1
• It should next display all the linked lists in the lists entered by the user
• Next, it should accept a number - this is an index position for the list (a linked list)
3
7
1
The output should be:
linked list [ 0 ]:
1 3 5 7
linked list [ 1 ]:
34729
the smallest node is:
2
List 1 after removing the smallest node:
3479
Note Make sure to check for the following possible user's mistakes
• if the user inputs a negative number as the number of linked lists in the list, your code should display: "Wrong input! try again:"; then,
let the user to input another number
• Same for the Empty linked lists](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F45d19586-ebc3-4f1a-8702-47e93c6193b1%2F7a1161d8-312f-4638-a78d-efd6dde33588%2Fzdl0oi_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

I'm getting the errors in this image for the below code:
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def prepend(self, new_node):
if self.head is None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head = new_node
def insert_after(self, current_node, new_node):
if self.head is None:
self.head = new_node
self.tail = new_node
elif current_node is self.tail:
self.tail.next = new_node
self.tail = new_node
else:
new_node.next = current_node.next
current_node.next = new_node
def remove_after(self, current_node):
if (current_node is None) and (self.head is not None):
succeeding_node = self.head.next
self.head = succeeding_node
if succeeding_node is None: # Remove last item
self.tail = None
elif current_node.next is not None:
succeeding_node = current_node.next.next
current_node.next = succeeding_node
if succeeding_node is None: # Remove tail
self.tail = current_node
def display(self):
node = self.head
if node == None:
print('Empty!')
while node is not None:
print(node.data, end=' ')
node = node.next
def remove_smallest_node(self):
# *** Your code goes here!***
temp_prev = None
temp = self.head
smallest = self.head
prev = None
while temp is not None:
if temp.data < smallest.data:
smallest = temp
prev = temp_prev
temp_prev = temp
temp = temp.next
if smallest == self.head and smallest == self.tail:
self.head = self.tail = None
elif smallest == self.head:
self.head = self.head.next
elif smallest == self.tail:
self.tail = prev
prev.next = None
else:
prev.next = smallest.next
return smallest.data
def Generate_List_of_LinkedLists(n):
LLL = []
# *** Your code goes here!***
for i in range(n):
LLL.append(LinkedList())
return LLL
if __name__ == '__main__':
# *** Your code goes here!***
n = int(input())
LLL = Generate_List_of_LinkedLists(n)
for i in range(n):
x = int(input())
while x != -1:
node = Node(x)
LLL[i].append(node)
x = int(input())
for i in range(n):
print("\nlinked list [", i, "]:")
LLL[i].display()
print("")
x = int(input())
while x >= n or LLL[x].head is None:
if x >= n:
x = int(input("Wrong input! try again:"))
else:
x = int(input("Wrong input! try again:"))
smallest = LLL[x].remove_smallest_node()
print("the smallest node is:")
print(smallest)
print("List 1 after removing smallest node: ")
LLL[x].display()
![Input
Your output
Expected output
1
#
linked list [ 0 ]:
1 3 5 7
linked list [ 1 ]:
34729
the smallest node is:
2
List 1 after removing smallest node:
3479
linked list [ 0 ]:
1 3 5 7
linked list [ 1 ]:
34729
the smallest node is:
2
List 1 after removing smallest node:
3479
error 1
Output differs. See highlights below. Special character legend
Input
Your output
Expected output
-
9
0
List 1 after removing smallest node:
234
linked list [ 0 ]:
2340
Input
Wrong input! try again
Wrong input! try again
the smallest node is:
0
List after removing smallest node:
234
Your output
A
linked list [0]:
2340
Wrong input! try again: Wrong input! try again: the smallest node is:
0
Expected output
error 4
1
0
3
4
1
5
-1
0
e
error 2
linked list [0]:
03415
the smallest node is:
0
List 1 after removing smallest node:
3 4 15
linked list [ 0 ]:
0 3 4 15
the smallest node is:
0
List 0 after removing smallest node:
3 4 15
Traceback (most recent call last):
File "main.py", line 108, in <module>
while x >= n or LLL [x].head is None:
IndexError: list index out of range
Output differs. See highlights below.
Expected output
Input
Your output
Your output
Expected output
Input
1
-1
1
-1
0
0
اله
2
3
4
1
5
0
d
Wrong input! try again: Wrong input! try again: Wrong input! try again: Wrc
Wrong input! try again:
linke
[0]:4
2 3 4154
Traceback (most recent call last):
File "main.py", line 112, in <module>
x = int (input ("Wrong input! try again:"))
EOFError: EOF when reading a line
Output differs. See highlights below. Special character legend
the
14
Special character legend
smallest node is:
List 0 after removing smallest node: <
2 3 4 5
linked list [ 0 ]:
Empty!
Wrong input! try again:
linked list [ 0 ]:
1
the smallest node is:<
14
error 3
Wrong input! try again: Wrong input! try again: Wrong input! try again:
List 0 after removing smallest node: <
Empty!
error 5](https://content.bartleby.com/qna-images/question/45d19586-ebc3-4f1a-8702-47e93c6193b1/67399859-5db2-4071-81ea-f7043fdec075/iz0sjfd_thumbnail.png)
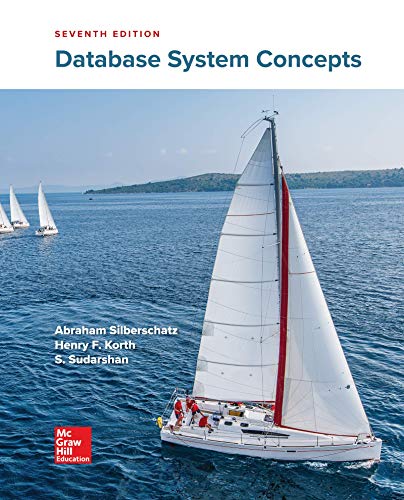
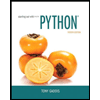
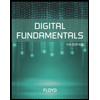
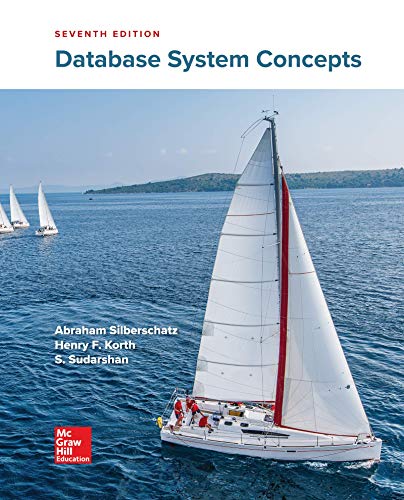
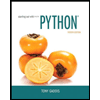
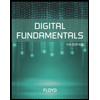
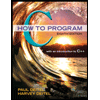
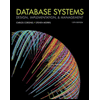
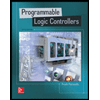