HOW CAN I Addlast ,DeleteLast,AddAt,and DeleteAt.
class Node{
int data;
Node next;
public Node (int d) {
this(d,null);
}
public Node (int d,Node n) {
data=d;
next=n;
}
}
public class List {
private Node head;
public List() {
head=null;
}
public void addBegenning(int d) {
Node n= new Node(d);
if (head==null) {
head=n;
}else {
n.next=head;
head=n;
}
}
public void addEnd(int d) {
if (head==null) {
head=new Node(d);
}else {
Node tmp=head;
while(tmp.next!=null)
tmp=tmp.next;
tmp.next=new Node(d);
}
}
public String toString() {
Node tmp=head;
String ans="";
while(tmp!=null) {
ans+=tmp.data+"-->";
tmp=tmp.next;
}
return ans;
}
public void deleteBegging() {
if (head!=null) {
head=head.next;
}
}
public void deleteEnd() {
Node tmp=head;
Node prev= null;
while(tmp.next!=null) {
prev=tmp;
tmp=tmp.next;
}
prev.next=null;
}
public boolean contains(int d) {
Node tmp=head;
while (tmp!=null) {
if (tmp.data==d)return true;
tmp=tmp.next;
}
return false;
}
public void Remove(int d) {//if the d not exsist
Node tmp=head;
Node prev= null;
while (tmp.data!=d) {// tmp!=null &&
prev=tmp;
tmp=tmp.next;
}
prev.next=tmp.next;
}
public static void main(String[]args) {
List L = new List();
L.addBegenning(10);
L.addBegenning(20);
L.addBegenning(5);
L.addEnd(50);
L.addEnd(100);
L.addBegenning(1);
System.out.println(L);
L.deleteBegging();
System.out.println(L);
L.deleteEnd();
System.out.println(L);
L.contains(20);
System.out.println(L.contains(20));
System.out.println(L.contains(100));
}
}
HOW CAN I Addlast ,DeleteLast,AddAt,and DeleteAt.

Solution:--
1)The given question has required for the solution which is to be provided as the functions
of the Java language.
2)The functions given in the question are as follows:--
i)addLast
ii)deleteLast
iii)AddAt
iv)DeleteAt
3)The given above functions are required to be expressed in the code of the Java program
respectively.
4)Note:-- I have provided the required functions in Java in the following below step
respectively.
Step by step
Solved in 2 steps

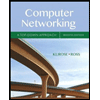
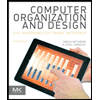
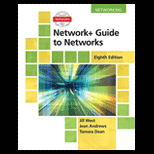
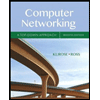
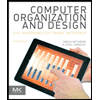
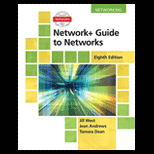
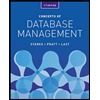
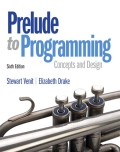
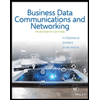