import java.util.Scanner; class InventoryNode { private String item; private int numberOfItems; private InventoryNode nextNodeRef; // Reference to the next node public InventoryNode() { item = ""; numberOfItems = 0; nextNodeRef = null; } public InventoryNode(String itemInit, int numberOfItemsInit) { this.item = itemInit; this.numberOfItems = numberOfItemsInit; this.nextNodeRef = null; } public InventoryNode(String itemInit, int numberOfItemsInit, InventoryNode nextLoc) { this.item = itemInit; this.numberOfItems = numberOfItemsInit; this.nextNodeRef = nextLoc; } // Getter for item public String getItem() { return item; } // Getter for numberOfItems public int getNumberOfItems() { return numberOfItems; } // Getter for nextNodeRef public InventoryNode getNext() { return this.nextNodeRef; } // Setter for nextNodeRef public void setNext(InventoryNode nextNode) { this.nextNodeRef = nextNode; } // Insert a node at the front of the linked list (after the dummy head node) public void insertAtFront(InventoryNode head, InventoryNode newNode) { newNode.setNext(head.getNext()); head.setNext(newNode); } // Print node data public void printNodeData() { System.out.print(this.numberOfItems + " " + this.item + " "); } } import java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode = currNode.getNext(); } } }
import java.util.Scanner;
class InventoryNode {
private String item;
private int numberOfItems;
private InventoryNode nextNodeRef; // Reference to the next node
public InventoryNode() {
item = "";
numberOfItems = 0;
nextNodeRef = null;
}
public InventoryNode(String itemInit, int numberOfItemsInit) {
this.item = itemInit;
this.numberOfItems = numberOfItemsInit;
this.nextNodeRef = null;
}
public InventoryNode(String itemInit, int numberOfItemsInit, InventoryNode nextLoc) {
this.item = itemInit;
this.numberOfItems = numberOfItemsInit;
this.nextNodeRef = nextLoc;
}
// Getter for item
public String getItem() {
return item;
}
// Getter for numberOfItems
public int getNumberOfItems() {
return numberOfItems;
}
// Getter for nextNodeRef
public InventoryNode getNext() {
return this.nextNodeRef;
}
// Setter for nextNodeRef
public void setNext(InventoryNode nextNode) {
this.nextNodeRef = nextNode;
}
// Insert a node at the front of the linked list (after the dummy head node)
public void insertAtFront(InventoryNode head, InventoryNode newNode) {
newNode.setNext(head.getNext());
head.setNext(newNode);
}
// Print node data
public void printNodeData() {
System.out.print(this.numberOfItems + " " + this.item + " ");
}
}
import java.util.Scanner;
public class Inventory {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
InventoryNode headNode;
InventoryNode currNode;
InventoryNode lastNode;
String item;
int numberOfItems;
int i;
// Front of nodes list
headNode = new InventoryNode();
lastNode = headNode;
int input = scnr.nextInt();
for(i = 0; i < input; i++ ) {
item = scnr.next();
numberOfItems = scnr.nextInt();
currNode = new InventoryNode(item, numberOfItems);
currNode.insertAtFront(headNode, currNode);
lastNode = currNode;
}
// Print linked list
currNode = headNode.getNext();
while (currNode != null) {
currNode.printNodeData();
currNode = currNode.getNext();
}
}
}


Step by step
Solved in 3 steps with 1 images

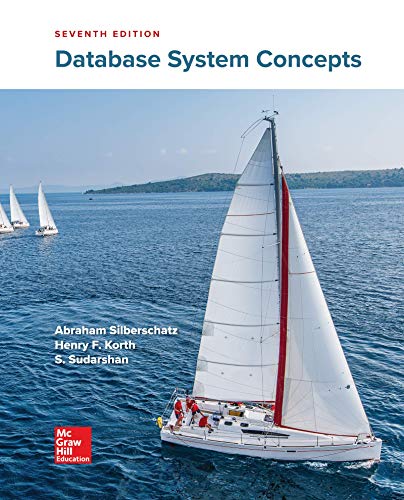
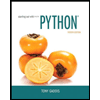
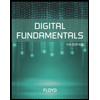
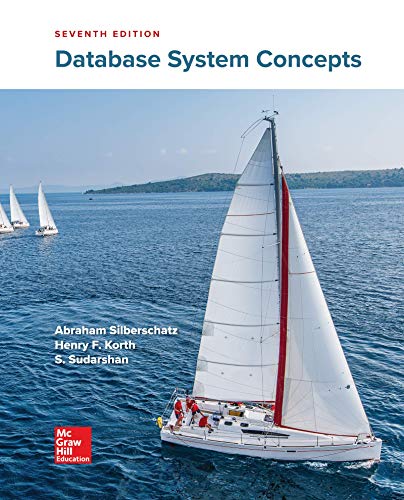
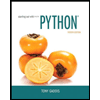
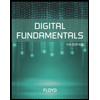
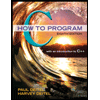
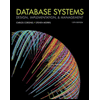
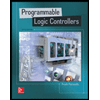