hello. This is my code: #include #include #include typedef struct HuffmanNode { int character; float frequency; struct HuffmanNode* left; struct HuffmanNode* right; } HuffmanNode; typedef struct HeapNode { HuffmanNode* node; struct HeapNode* next; } HeapNode; HuffmanNode* create_huffman_node(int character, float frequency) { HuffmanNode* node = (HuffmanNode*)malloc(sizeof(HuffmanNode)); node->character = character; node->frequency = frequency; node->left = NULL; node->right = NULL; return node; } HeapNode* create_heap_node(HuffmanNode* node) { HeapNode* heap_node = (HeapNode*)malloc(sizeof(HeapNode)); heap_node->node = node; heap_node->next = NULL; return heap_node; } HeapNode* insert_into_heap(HeapNode* heap, HuffmanNode* node) { HeapNode* heap_node = create_heap_node(node); if (heap == NULL) { return heap_node; } // Compare nodes based on probability and character value if (heap_node->node->frequency < heap->node->frequency || (heap_node->node->frequency == heap->node->frequency && heap_node->node->character < heap->node->character)) { heap_node->next = heap; return heap_node; } HeapNode* current = heap; while (current->next != NULL && (current->next->node->frequency < heap_node->node->frequency || (current->next->node->frequency == heap_node->node->frequency && current->next->node->character < heap_node->node->character))) { current = current->next; } heap_node->next = current->next; current->next = heap_node; return heap; } HuffmanNode* build_huffman_tree(float* count) { HuffmanNode* nodes[128] = {NULL}; // Array to store nodes based on ASCII value for (int i = 0; i < 128; i++) { if (count[i] > 0) { HuffmanNode* node = create_huffman_node(i, count[i]); nodes[i] = node; } } HeapNode* heap = NULL; // Insert nodes into the heap for (int i = 0; i < 128; i++) { if (nodes[i] != NULL) { heap = insert_into_heap(heap, nodes[i]); } } while (heap->next != NULL) { HuffmanNode* node1 = heap->node; heap = heap->next; HuffmanNode* node2 = heap->node; heap = heap->next; HuffmanNode* new_node = create_huffman_node(-1, node1->frequency + node2->frequency); new_node->left = node1; new_node->right = node2; heap = insert_into_heap(heap, new_node); } return heap->node; } void print_huffman_codes(HuffmanNode* node, int* code, int code_length) { if (node == NULL) { return; } code[code_length] = 0; print_huffman_codes(node->left, code, code_length + 1); if (node->character != -1) { printf("| %-5d | %.5f | ", node->character, node->frequency / 100); for (int i = 0; i < code_length; i++) { printf("%d", code[i]); } printf(" |\n"); } code[code_length] = 1; print_huffman_codes(node->right, code, code_length + 1); } void huffman_encoding(const char* filename) { float count[128] = {0}; FILE* file = fopen(filename, "r"); if (file == NULL) { printf("File not found.\n"); return; } int c; while ((c = fgetc(file)) != EOF) { if (c >= 0 && c < 128) { count[c]++; } } fclose(file); HuffmanNode* root = build_huffman_tree(count); printf("| ASCII | Percent | Code |\n"); printf("|-------|---------|------|\n"); int code[128] = {0}; print_huffman_codes(root, code, 0); free(root); } int main() { char filename[256]; printf("Enter File Name to read: "); scanf("%s", filename); huffman_encoding(filename); return 0; } It is not in the same format as the expected output and I don't know how to fix it. please help
hello. This is my code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct HuffmanNode {
int character;
float frequency;
struct HuffmanNode* left;
struct HuffmanNode* right;
} HuffmanNode;
typedef struct HeapNode {
HuffmanNode* node;
struct HeapNode* next;
} HeapNode;
HuffmanNode* create_huffman_node(int character, float frequency) {
HuffmanNode* node = (HuffmanNode*)malloc(sizeof(HuffmanNode));
node->character = character;
node->frequency = frequency;
node->left = NULL;
node->right = NULL;
return node;
}
HeapNode* create_heap_node(HuffmanNode* node) {
HeapNode* heap_node = (HeapNode*)malloc(sizeof(HeapNode));
heap_node->node = node;
heap_node->next = NULL;
return heap_node;
}
HeapNode* insert_into_heap(HeapNode* heap, HuffmanNode* node) {
HeapNode* heap_node = create_heap_node(node);
if (heap == NULL) {
return heap_node;
}
// Compare nodes based on probability and character value
if (heap_node->node->frequency < heap->node->frequency ||
(heap_node->node->frequency == heap->node->frequency && heap_node->node->character < heap->node->character)) {
heap_node->next = heap;
return heap_node;
}
HeapNode* current = heap;
while (current->next != NULL && (current->next->node->frequency < heap_node->node->frequency ||
(current->next->node->frequency == heap_node->node->frequency &&
current->next->node->character < heap_node->node->character))) {
current = current->next;
}
heap_node->next = current->next;
current->next = heap_node;
return heap;
}
HuffmanNode* build_huffman_tree(float* count) {
HuffmanNode* nodes[128] = {NULL}; // Array to store nodes based on ASCII value
for (int i = 0; i < 128; i++) {
if (count[i] > 0) {
HuffmanNode* node = create_huffman_node(i, count[i]);
nodes[i] = node;
}
}
HeapNode* heap = NULL;
// Insert nodes into the heap
for (int i = 0; i < 128; i++) {
if (nodes[i] != NULL) {
heap = insert_into_heap(heap, nodes[i]);
}
}
while (heap->next != NULL) {
HuffmanNode* node1 = heap->node;
heap = heap->next;
HuffmanNode* node2 = heap->node;
heap = heap->next;
HuffmanNode* new_node = create_huffman_node(-1, node1->frequency + node2->frequency);
new_node->left = node1;
new_node->right = node2;
heap = insert_into_heap(heap, new_node);
}
return heap->node;
}
void print_huffman_codes(HuffmanNode* node, int* code, int code_length) {
if (node == NULL) {
return;
}
code[code_length] = 0;
print_huffman_codes(node->left, code, code_length + 1);
if (node->character != -1) {
printf("| %-5d | %.5f | ", node->character, node->frequency / 100);
for (int i = 0; i < code_length; i++) {
printf("%d", code[i]);
}
printf(" |\n");
}
code[code_length] = 1;
print_huffman_codes(node->right, code, code_length + 1);
}
void huffman_encoding(const char* filename) {
float count[128] = {0};
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("File not found.\n");
return;
}
int c;
while ((c = fgetc(file)) != EOF) {
if (c >= 0 && c < 128) {
count[c]++;
}
}
fclose(file);
HuffmanNode* root = build_huffman_tree(count);
printf("| ASCII | Percent | Code |\n");
printf("|-------|---------|------|\n");
int code[128] = {0};
print_huffman_codes(root, code, 0);
free(root);
}
int main() {
char filename[256];
printf("Enter File Name to read: ");
scanf("%s", filename);
huffman_encoding(filename);
return 0;
}
It is not in the same format as the expected output and I don't know how to fix it. please help


Step by step
Solved in 3 steps

hello. i tried it out and it still didn't work
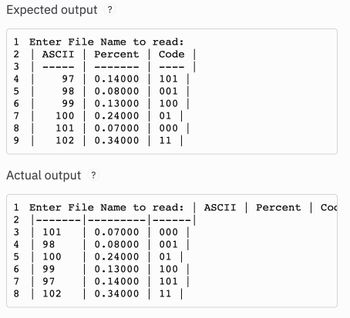
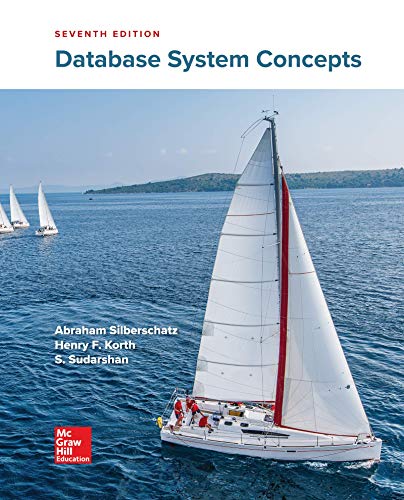
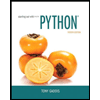
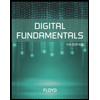
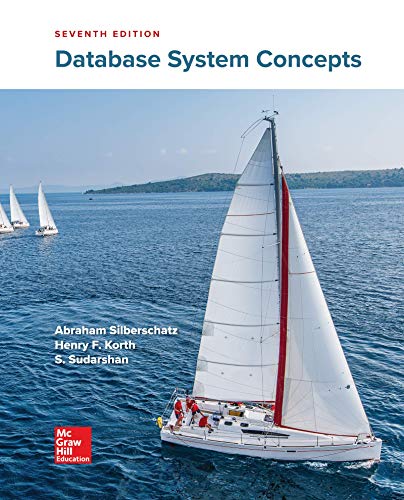
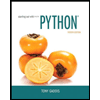
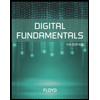
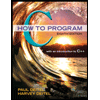
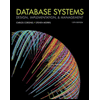
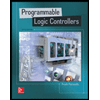