Please code in C language. Please use the starter code to help you solve the deleted node and the reverse list. Here is the starter code: #include #include #include #include #include "linkedlist.h" // print an error message by an error number, and return // the function does not exit from the program // the function does not return a value void error_message(enum ErrorNumber errno) { char *messages[] = { "OK", "Memory allocaton failed.", "Deleting a node is not supported.", "The number is not on the list.", "Sorting is not supported.", "Reversing is not supported.", "Token is too long.", "A number should be specified after character d, a, or p.", "Token is not recognized.", "Invalid error number."}; if (errno < 0 || errno > ERR_END) errno = ERR_END; printf("linkedlist: %s\n", messages[errno]); } node *new_node(int v) { node *p = malloc(sizeof(node)); // Allocate memory if (p == NULL) { error_message(ERR_NOMEM); exit(-1); } // Set the value in the node. p->v = v; // you could do (*p).v p->next = NULL; return p; // return } node *prepend(node *head, node *newnode) { newnode->next = head; return newnode; } node *find_node(node *head, int v) { while (head != NULL) { if (head->v == v) return head; head = head->next; } return head; } node *find_last(node *head) { if (head != NULL) { while (head->next != NULL) head = head->next; } return head; } node *append(node *head, node *newnode) { node *p = find_last(head); newnode->next = NULL; if (p == NULL) return newnode; p->next = newnode; return head; } node *delete_list(node *head) { while (head != NULL) { node *p = head->next; free(head); head = p; } return head; } void print_list(node *head) { printf("["); while (head) { printf("%d, ", head->v); head = head->next; } printf("]\n"); } void print_node(node *p) { printf("%p: v=%-5d next=%p\n", p, p->v, p->next); } void print_list_details(node *head) { while (head) { print_node(head); head = head->next; } } // functions that have not been implemented node *delete_node(node *head, int v) { // TODO error_message(ERR_NODELETE); return head; } /* * Given a pointer to the head node of an acyclic list, change the * next links such that the nodes are linked in reverse order. * Allocating new nodes or copying values from one node to another * is not allowed, but you may use additional pointer variables. * Return value is a pointer to the new head node. */ node *reverse_list(node *head) { // TODO error_message(ERR_NOREVERSE); return head; }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Please code in C language. Please use the starter code to help you solve the deleted node and the reverse list. Here is the starter code:
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <string.h>
#include "linkedlist.h"
// print an error message by an error number, and return
// the function does not exit from the program
// the function does not return a value
void error_message(enum ErrorNumber errno) {
char *messages[] = {
"OK",
"Memory allocaton failed.",
"Deleting a node is not supported.",
"The number is not on the list.",
"Sorting is not supported.",
"Reversing is not supported.",
"Token is too long.",
"A number should be specified after character d, a, or p.",
"Token is not recognized.",
"Invalid error number."};
if (errno < 0 || errno > ERR_END)
errno = ERR_END;
printf("linkedlist: %s\n", messages[errno]);
}
node *new_node(int v) {
node *p = malloc(sizeof(node)); // Allocate memory
if (p == NULL) {
error_message(ERR_NOMEM);
exit(-1);
}
// Set the value in the node.
p->v = v; // you could do (*p).v
p->next = NULL;
return p; // return
}
node *prepend(node *head, node *newnode) {
newnode->next = head;
return newnode;
}
node *find_node(node *head, int v) {
while (head != NULL) {
if (head->v == v)
return head;
head = head->next;
}
return head;
}
node *find_last(node *head) {
if (head != NULL) {
while (head->next != NULL)
head = head->next;
}
return head;
}
node *append(node *head, node *newnode) {
node *p = find_last(head);
newnode->next = NULL;
if (p == NULL)
return newnode;
p->next = newnode;
return head;
}
node *delete_list(node *head) {
while (head != NULL) {
node *p = head->next;
free(head);
head = p;
}
return head;
}
void print_list(node *head) {
printf("[");
while (head) {
printf("%d, ", head->v);
head = head->next;
}
printf("]\n");
}
void print_node(node *p) {
printf("%p: v=%-5d next=%p\n", p, p->v, p->next);
}
void print_list_details(node *head) {
while (head) {
print_node(head);
head = head->next;
}
}
// functions that have not been implemented
node *delete_node(node *head, int v) {
// TODO
error_message(ERR_NODELETE);
return head;
}
/*
* Given a pointer to the head node of an acyclic list, change the
* next links such that the nodes are linked in reverse order.
* Allocating new nodes or copying values from one node to another
* is not allowed, but you may use additional pointer variables.
* Return value is a pointer to the new head node.
*/
node *reverse_list(node *head) {
// TODO
error_message(ERR_NOREVERSE);
return head;
}
![Part 1. Delete node. The linked list example we have studied in lecture maintains a singly linked list of
integers. The program can append and prepend integers to the list. Type help in the program to see a list
of commands. Note that if the number to be added is already on the list, the program prints the information
about the node that stores the number and does not add the number twice.
The list is displayed every time an integer is processed.
Currently, the program does not support the delete function. Complete the function delete_node () in
linkedlist.c so that the program can delete an integer from the list. The prototype of the function is:
node* delete_node (node * head, int v);
head is a pointer to the head node and v is the integer to be removed. The function returns the pointer
to the head node of the new list, which could be the same as head. If v is not on the list, the function prints
a message using the following function call: error_message (ERR_NOTFOUND).
Below is an example session using the delete feature.
$
1 2 3 4 5 6 7 8 9
./linkedlist-main
[1, ]
[1, 2, ]
[1, 2, 3, ]
[1, 2, 3, 4, ]
[1, 2, 3, 4, 5, ]
[1, 2, 3, 4, 5, 6, 1
5,
5, 6, 7, 8, ]
[1, 2, 3, 4,
[1, 2, 3, 4,
6, 7, ]
[1, 2, 3, 4, 5,
d5
[1, 2, 3, 4,
d3
[1, 2, 4, 6, 7, 8, 9, 1
d3
6, 7, 8, 9, ]
6, 7, 8, 9, ]
linkedlist: The number is not on the list.
[1, 2, 4, 6, 7, 8, 9, 1
Part 2. List reversal. In this exercise, we implement another function reverse_list() in linkedlist.
The prototype of the function is:
node reverse_list (node * head);
The function receives head as its sole argument, a pointer to the head node of the linked list. Assum
the list is acyclic. The function must change the next fields of each node so that the nodes end up linke
in reverse order. The function must return the address of the new head node (originally last in the list
You may use additional functions and local variables besides those already included in the starter code, bu
cannot change the values stored in the nodes or dynamically allocate new nodes. You can use either a loc
or recursion.
Below is an example session using the reversal feature.
$ ./linkedlist-main
1 2 3 4 5 6 7 8 9
[1, ]
[1, 2, ]
[1, 2, 3, ]
[1, 2, 3, 4, ]
[1, 2, 3, 4, 5, ]
[1, 2, 3, 4, 5, 6, ]
[1, 2, 3, 4, 5, 6, 7, 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa5602bda-7b39-4a2b-a1a8-06efc64b3411%2F216cdb42-dbbf-4c4c-984c-a23ceb245153%2Fcly3xfj_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

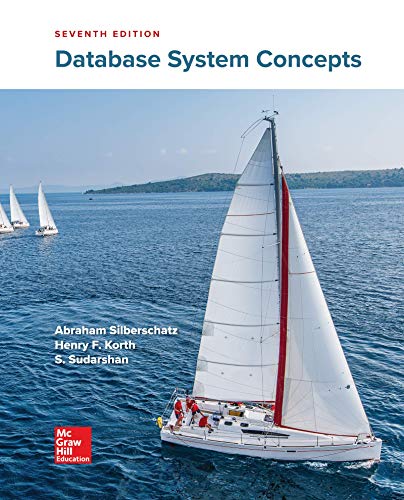
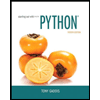
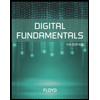
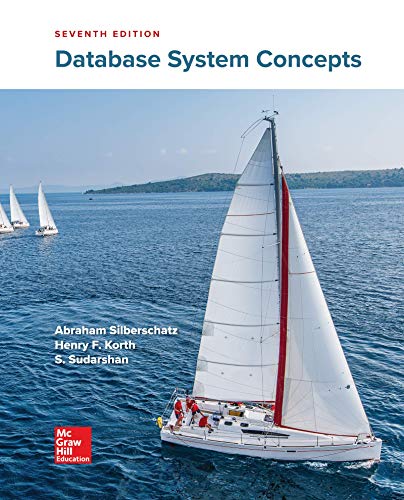
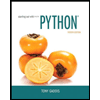
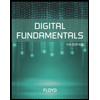
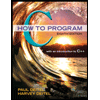
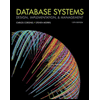
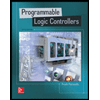