import edu.princeton.cs.algs4.StdOut; public class LinkedIntListR { // helper linked list node class private class Node { private int item; private Node next; public Node(int item, Node next) { this.item = item; this.next = next; } } private Node first; // first node of the list public LinkedIntListR() { first = null; } public LinkedIntListR(int[] data) { for (int i = data.length - 1; i >= 0; i--) first = new Node(data[i], first); } public void addFirst(int data) { first = new Node(data, first); } public void printList() { if (first == null) { StdOut.println("[]"); } else if (first.next == null) { StdOut.println("[" + first.item + "]"); } else { StdOut.print("[" + first.item); for(Node ptr = first.next; ptr != null; ptr = ptr.next) StdOut.print(", " + ptr.item); StdOut.println("]"); } } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; LinkedIntListR other = (LinkedIntListR) obj; Node thisPtr = first; Node otherPtr = other.first; while(thisPtr != null && otherPtr != null) { if (thisPtr.item != otherPtr.item) return false; thisPtr = thisPtr.next; otherPtr = otherPtr.next; } if (thisPtr != null || otherPtr != null) return false; return true; } /********************************** HOMEWORK **********************************/ /** * Computes the sum of all the ints in the list. * * @return the sum of all the ints in the list */ public int sum() { // TODO throw new RuntimeException("Not implemented"); } /** * Returns the index of the first occurrence of the specified int in this list, * or -1 if this list does not contain the int. * * @param i int to search for * @return the index of the first occurrence of the specified int in this list, * or -1 if this list does not contain the int */ public int indexOf(int i) { // TODO throw new RuntimeException("Not implemented"); // HINT:The empty list is not the only base case. //If the first item in the list is the one you are looking for //you should also stop because you have found it. } /** * Constructs a string representing the list. The ints in the list appear in a * comma separated list contained inside square brackets. * * @return a string represeting the list. */ public String toString() { // TODO throw new RuntimeException("Not implemented"); // HINT:Have the private method just return comma separted list //and have the public method put the [ and ] around the private //method's answer. //Also, you probably need a base case for a list of size 1 as well //The empty list can be handled in the public method if you want. } /** * Constructs a new {@code LinkedIntList} consisting of just the even number * from this list appearing in the same order as they occur in this list. * * @return a new list that looks like this list be with all the odds removed. */ public LinkedIntListR evens() { // TODO throw new RuntimeException("Not implemented"); // HINT:Have the private method return a list via the first node. //Have the public method create an empty list and then set //first in that empty list to the list returned by the helper } /** * Constructs a new int list where each entry in the new list is the sum of the * corresponding entries in this list and the argument list. * * @arg otherList the other list to be added to this list * @return a new list where each entry in the new list is the sum of the * corresponding entries in this list and the argument list * @throws IllegalArgumentException if this list and the other list have have * different lengths */ public LinkedIntListR listAddition(LinkedIntListR otherList) { // TODO throw new RuntimeException("Not implemented"); // HINT:Have the private method return a list via the first node. //Have the public method create an empty list and then set //first in that empty list to the list returned by the helper } }
import edu.princeton.cs.algs4.StdOut;
public class LinkedIntListR {
// helper linked list node class
private class Node {
private int item;
private Node next;
public Node(int item, Node next) {
this.item = item;
this.next = next;
}
}
private Node first; // first node of the list
public LinkedIntListR() {
first = null;
}
public LinkedIntListR(int[] data) {
for (int i = data.length - 1; i >= 0; i--)
first = new Node(data[i], first);
}
public void addFirst(int data) {
first = new Node(data, first);
}
public void printList() {
if (first == null) {
StdOut.println("[]");
} else if (first.next == null) {
StdOut.println("[" + first.item + "]");
} else {
StdOut.print("[" + first.item);
for(Node ptr = first.next; ptr != null; ptr = ptr.next)
StdOut.print(", " + ptr.item);
StdOut.println("]");
}
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
LinkedIntListR other = (LinkedIntListR) obj;
Node thisPtr = first;
Node otherPtr = other.first;
while(thisPtr != null && otherPtr != null) {
if (thisPtr.item != otherPtr.item)
return false;
thisPtr = thisPtr.next;
otherPtr = otherPtr.next;
}
if (thisPtr != null || otherPtr != null)
return false;
return true;
}
/********************************** HOMEWORK **********************************/
/**
* Computes the sum of all the ints in the list.
*
* @return the sum of all the ints in the list
*/
public int sum() { // TODO
throw new RuntimeException("Not implemented");
}
/**
* Returns the index of the first occurrence of the specified int in this list,
* or -1 if this list does not contain the int.
*
* @param i int to search for
* @return the index of the first occurrence of the specified int in this list,
* or -1 if this list does not contain the int
*/
public int indexOf(int i) { // TODO
throw new RuntimeException("Not implemented");
// HINT:The empty list is not the only base case.
//If the first item in the list is the one you are looking for
//you should also stop because you have found it.
}
/**
* Constructs a string representing the list. The ints in the list appear in a
* comma separated list contained inside square brackets.
*
* @return a string represeting the list.
*/
public String toString() { // TODO
throw new RuntimeException("Not implemented");
// HINT:Have the private method just return comma separted list
//and have the public method put the [ and ] around the private
//method's answer.
//Also, you probably need a base case for a list of size 1 as well
//The empty list can be handled in the public method if you want.
}
/**
* Constructs a new {@code LinkedIntList} consisting of just the even number
* from this list appearing in the same order as they occur in this list.
*
* @return a new list that looks like this list be with all the odds removed.
*/
public LinkedIntListR evens() { // TODO
throw new RuntimeException("Not implemented");
// HINT:Have the private method return a list via the first node.
//Have the public method create an empty list and then set
//first in that empty list to the list returned by the helper
}
/**
* Constructs a new int list where each entry in the new list is the sum of the
* corresponding entries in this list and the argument list.
*
* @arg otherList the other list to be added to this list
* @return a new list where each entry in the new list is the sum of the
* corresponding entries in this list and the argument list
* @throws IllegalArgumentException if this list and the other list have have
* different lengths
*/
public LinkedIntListR listAddition(LinkedIntListR otherList) { // TODO
throw new RuntimeException("Not implemented");
// HINT:Have the private method return a list via the first node.
//Have the public method create an empty list and then set
//first in that empty list to the list returned by the helper
}
}
![thisPtr = thisPtr.next;
otherPtr = otherPtr. next;
}
if (thisPtr != null || otherPtr != null)
return false;
return true;
}
/*******
****** HOMEWORK ********
*********/
/**
* Computes the sum of all the ints in the list.
* @return the sum of all the ints in the list
*/
public int sum() { // TODO
throw new RuntimeException ("Not implemented");
}
/**
* Returns the index of the first occurrence of the specified int in this list,
* or -1 if this list does not contain the int.
*
* @param i int to search for
* @return the index of the first occurrence of the specified int in this list,
or -1 if this list does not contain the int
*/
public int index0f (int i) { // TODO
throw new RuntimeException("Not implemented");
// HINT:
//
//
}
The empty list is not the only base case.
If the first item in the list is the one you are looking for
you should also stop because you have found it.
/**
* Constructs a string representing the list. The ints in the list appear in a
* comma separated list contained inside square brackets.
* @return a string represeting the list.
*/
public String toString() { // TODO
throw new RuntimeException("Not implemented");
// HINT:
//
Have the private method just return comma separted list
and have the public method put the [ and ] around the private
method's answer.
Also, you probably need a base case for a list of size 1 as well
The empty list can be handled in the public method if you want.
}
/**
* Constructs a new {@code LinkedIntList} consisting of just the even number
* from this list appearing in the same order as they occur in this list.
* @return a new list that looks like this list be with all the odds removed.
*/](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2426aaa0-cd69-40ca-b1a2-ff638b3c6350%2F23dd8adb-73f3-4feb-9ff7-a0565ec81370%2F6nrhs7p_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

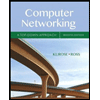
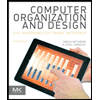
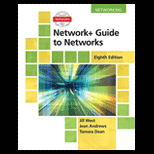
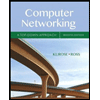
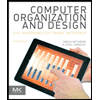
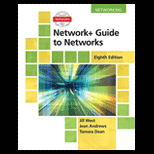
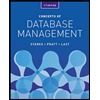
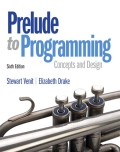
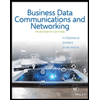