Please help me how can I write the code here #include #include // Function to repeat a string by a given integer std::string multiply(const std::string& str, int num) { std::string result; for (int i = 0; i < num; ++i) { result += str; } return result; } // Function to multiply two integers int multiply(int num1, int num2) { return num1 * num2; } // Function to multiply a double and an integer double multiply(double num1, int num2) { return num1 * num2; } // Function template to handle multiple data types template T multiply_type(T num1, int num2) { return num1 * num2; } int main() { int choice; std::cout << "Choose a test case (1-5): "; std::cin >> choice; switch (choice) { case 1: { int num1, num2; std::cout << "Enter two integers: "; std::cin >> num1 >> num2; int result = multiply(num1, num2); std::cout << "Output: " << result << std::endl; break; } case 2: { int num1, num2; std::cout << "Enter two integers: "; std::cin >> num1 >> num2; int result = multiply(num1, num2); std::cout << "Output: " << result << std::endl; break; } case 3: { double num1; int num2; std::cout << "Enter a double and an integer: "; std::cin >> num1 >> num2; double result = multiply(num1, num2); std::cout << "Output: " << result << std::endl; break; } case 4: { std::string str; int num; std::cout << "Enter a string and an integer: "; std::cin >> str >> num; std::string result = multiply(str, num); std::cout << "Output: " << result << std::endl; break; } case 5: { std::string str; int num; std::cout << "Enter a string and an integer: "; std::cin >> str >> num; std::string result = multiply(str, num); std::cout << "Output: " << result << std::endl; break; } default: std::cout << "Invalid choice!" << std::endl; } return 0; }
Please help me
how can I write the code here
#include <iostream>
#include <string>
// Function to repeat a string by a given integer
std::string multiply(const std::string& str, int num) {
std::string result;
for (int i = 0; i < num; ++i) {
result += str;
}
return result;
}
// Function to multiply two integers
int multiply(int num1, int num2) {
return num1 * num2;
}
// Function to multiply a double and an integer
double multiply(double num1, int num2) {
return num1 * num2;
}
// Function template to handle multiple data types
template <typename T>
T multiply_type(T num1, int num2) {
return num1 * num2;
}
int main() {
int choice;
std::cout << "Choose a test case (1-5): ";
std::cin >> choice;
switch (choice) {
case 1: {
int num1, num2;
std::cout << "Enter two integers: ";
std::cin >> num1 >> num2;
int result = multiply(num1, num2);
std::cout << "Output: " << result << std::endl;
break;
}
case 2: {
int num1, num2;
std::cout << "Enter two integers: ";
std::cin >> num1 >> num2;
int result = multiply(num1, num2);
std::cout << "Output: " << result << std::endl;
break;
}
case 3: {
double num1;
int num2;
std::cout << "Enter a double and an integer: ";
std::cin >> num1 >> num2;
double result = multiply(num1, num2);
std::cout << "Output: " << result << std::endl;
break;
}
case 4: {
std::string str;
int num;
std::cout << "Enter a string and an integer: ";
std::cin >> str >> num;
std::string result = multiply(str, num);
std::cout << "Output: " << result << std::endl;
break;
}
case 5: {
std::string str;
int num;
std::cout << "Enter a string and an integer: ";
std::cin >> str >> num;
std::string result = multiply(str, num);
std::cout << "Output: " << result << std::endl;
break;
}
default:
std::cout << "Invalid choice!" << std::endl;
}
return 0;
}
![## Understanding Function Implementation in C++
In this tutorial, we will delve into creating and implementing functions in C++. Specifically, we will focus on the `multiply` function and how to handle different data types. Below, you will find example code in multiple files to illustrate this concept.
### 1. **multiply.cpp**
This file contains the implementation of the multiply functions. Your task is to replace the placeholders (denoted by `...`) with the actual code.
```cpp
// Replace ... with your code. Hit enter key to get more vertical space
// IMPLEMENT
#include "multiply.h"
std::string multiply(std::string string1, int number){
...
}
int multiply(int number1, int number2){
...
}
double multiply(double number1, int number2){
...
}
```
### 2. **multiply.h**
The header file declares the `multiply` functions. Here, we use templates to allow our functions to handle different data types generically.
```cpp
// IMPLEMENT
#ifndef MULTIPLY_H
#define MULTIPLY_H
#include <string>
std::string multiply(std::string string1, int number);
int multiply(int number1, int number2);
double multiply(double number1, int number2);
// In templates, we can't separate the declaration from definition; We have to write it in a single location.
template<class T>
T multiply(T entity1, int number){
// Write your code below
// You may need to initialize your return value like,
// T return_value = T();
...
}
#endif // MULTIPLY_H
```
### 3. **multiply_driver.cpp**
In this file, we review and test our `multiply` functions. Replace the placeholders with your own test cases to validate the function implementation.
```cpp
// REVIEW
#include <iostream>
#include "multiply.h"
// Replace ... with your code. Hit enter key to get more vertical space
int main(int argc, char *argv[]){
// Testing multiply
std::cout << multiply(10, 10) << std::endl;
/* By exactly following the pattern above, test your function below for rest of the test cases
in the order they are given */
std::cout << "<Testing template function to prove the ability to write generic (type independent) code " << std::endl;
std::cout << multiply<int>(10, 10) << std::endl;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F69991f76-d6e5-4f32-9883-cbbc5a2c7e26%2F143464bf-f082-4039-9540-88dabff35615%2Fuz6jax_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

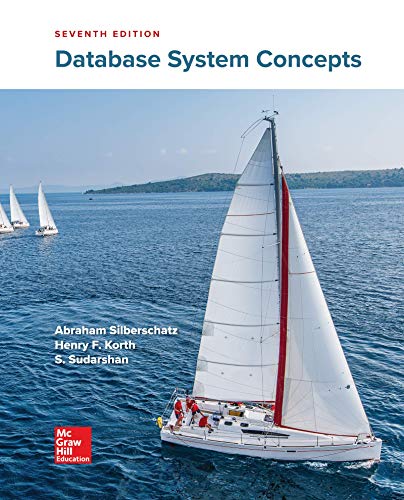
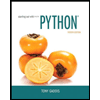
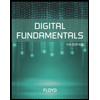
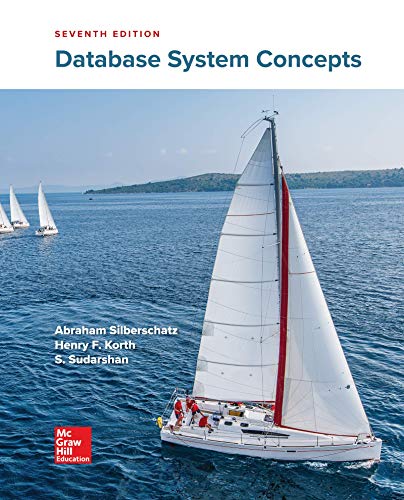
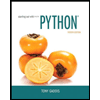
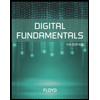
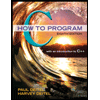
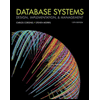
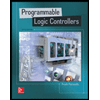