C PROGRAMMING Instructions: Use the code template below to implement the length() and reverse() functions into the main() /* This program will create a string which is the reverse of an original string using pointers. * Performs string handling, manipulates pointers, passes pointers to functions. */ #include #include int length( ) { while(str1[len++] != '\0'); // Calculate length of string len--; // Remove the null character len--; // Array index start from 0 to (length -1) return ; } void reverse( ) { while (counter < len) { i = str1[counter]; str1[counter] = str1[len]; str1[len] = i; counter++; len--; } return ; } int main () { char str1[41]; // String to be Reversed printf("Enter some text (No spaces and 40 characters max): "); scanf("%40s", str2); printf("Reversed string: %s\n", str1); return 0; }
C
Instructions: Use the code template below to implement the length() and reverse() functions into the main()
/* This program will create a string which is the reverse of an original string using pointers.
* Performs string handling, manipulates pointers, passes pointers to functions.
*/
#include <stdio.h>
#include <stdlib.h>
int length( )
{
while(str1[len++] != '\0'); // Calculate length of string
len--; // Remove the null character
len--; // Array index start from 0 to (length -1)
return ;
}
void reverse( )
{
while (counter < len)
{
i = str1[counter];
str1[counter] = str1[len];
str1[len] = i;
counter++;
len--;
}
return ;
}
int main ()
{
char str1[41]; // String to be Reversed
printf("Enter some text (No spaces and 40 characters max): ");
scanf("%40s", str2);
printf("Reversed string: %s\n", str1);
return 0;
}

Step by step
Solved in 2 steps with 2 images

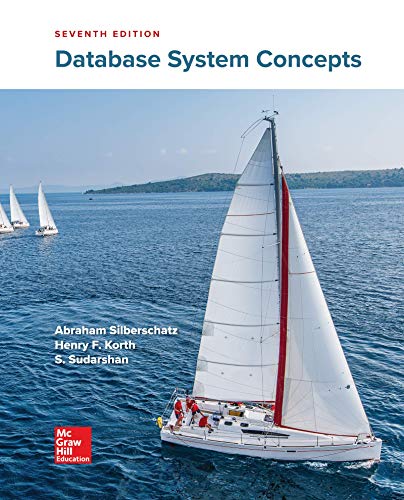
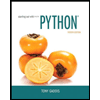
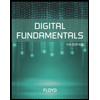
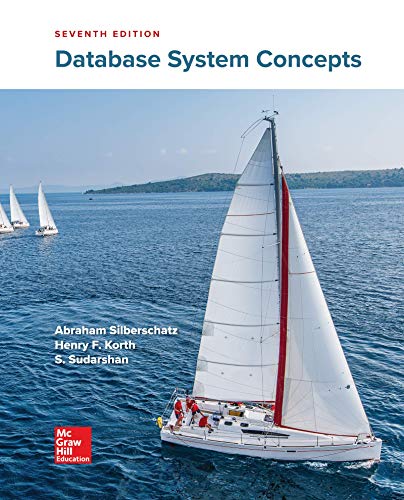
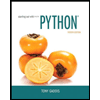
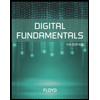
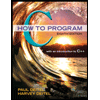
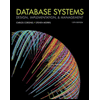
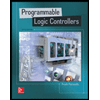