1) compareTo method must be negative not positive 2) the max value must be $24.09 but its showing "The Max is $4.80"
I have the following code for Money.java
public class Money {
private int dollar;
private int cent;
final static int MIN_CENT_VALUE = 0;
final static int MAX_CENT_VALUE = 99;
public Money() {
this.dollar = 0;
this.cent = 0;
}
public Money(int dollar, int cent) {
this.dollar = dollar;
if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) {
this.cent = cent;
} else {
cent = 0;
}
}
public int getDollar() {
return this.dollar;
}
public void setDollar(int dol) {
this.dollar = dol;
}
public int getCent() {
return this.cent;
}
public void setCent(int c) {
if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) {
this.cent = c;
}
}
public Money add(Money otherMoney) {
Money m = new Money();
m.dollar = this.dollar + otherMoney.dollar;
m.cent = this.cent + otherMoney.cent;
if (m.cent >= 100) {
m.dollar++;
m.cent -= 100;
}
return m;
}
public Money subtract(Money otherMoney) {
Money m = new Money();
m.dollar = this.dollar - otherMoney.dollar;
m.cent = this.cent - otherMoney.cent;
if (m.dollar < 0 || m.cent < 0) {
return null;
}
return m;
}
public boolean equals(Money otherMoney) {
return this == otherMoney;
}
public int compareTo(Money otherMoney) {
Money m = new Money();
if (this.dollar< otherMoney.dollar){
m = otherMoney.subtract(this);
return m.dollar*100+m.cent;
}
else if (this.dollar>otherMoney.dollar){
m = this.subtract(otherMoney);
return m.dollar*100+m.cent;
}
else{
return 0;
}
}
public String toString() {
if (this.cent<10){
return "$" + this.dollar + ".0" + this.cent;
}
return "$" + this.dollar + "." + this.cent;
}
public static Money max(Money[] m) {
int max = m[0].dollar+m[0].cent;
int index = 0;
for (int i = 0; i < m.length; i++) {
if (max < m[i].dollar+((m[i].cent)/100)) {
max = m[i].dollar+((m[i].cent)/100);
index = i;
}
}
return m[index];
}
public static void selectionSort(Money arr[]) {
int n = arr.length;
for (int i = 0; i < n -1; i++) {
int min = i;
for (int j = i + 1; j < n; j++)
if (arr[j].dollar+((arr[j].cent)/100.0) < arr[min].dollar+((arr[min].cent)/100.0))
min = j;
// Swap the found minimum element with the first element
Money temp = new Money();
temp = arr[min];
arr[min] = arr[i];
arr[i] = temp;
}
}
public static void printList(Money[] m, int i) // This method takes an array of object as parameter
{
for (Money mm : m){
System.out.print(mm + " ");
}
System.out.println();
}
}
_________________________________________
for use with the following unchangleable driver program TestMoney
import java.util.*;
public class TestMoney
{
public static void main(String[] args)
{
Random rnd = new Random(10);
Money m1 = new Money(5,45);
Money m2 = new Money(10,95);
m1.setCent(101);
Money sum = m1.add(m2);
System.out.println(m1+" + "+m2+" is "+sum);
Money diff = m1.subtract(m2);
System.out.println(m1+" - "+m2+" is "+diff);
diff = m2.subtract(m1);
System.out.println(m2+" - "+m1+" is "+diff);
System.out.println("equals method result: "+m1.equals(m2));
System.out.println("compareTo method result: "+m1.compareTo(m2));
Money[] list = new Money[15];
// Generate 15 random Money objects
for(int i=0;i<list.length;++i)
{
list[i] = new Money((int)(1+rnd.nextInt(30)),rnd.nextInt(100));
}
// print the list of Money objects, 10 per line
System.out.println("Unsorted list");
Money.printList(list,10);
// Find the largest Money object
System.out.println("The Max is "+Money.max(list));
// Sort and print
System.out.println("Sorted list");
Money.selectionSort(list);
Money.printList(list,10);
}
}
______________________________________
it's having 2 issues still
1) compareTo method must be negative not positive
2) the max value must be $24.09 but its showing "The Max is $4.80"


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

How can I correct spacing issue Thank you
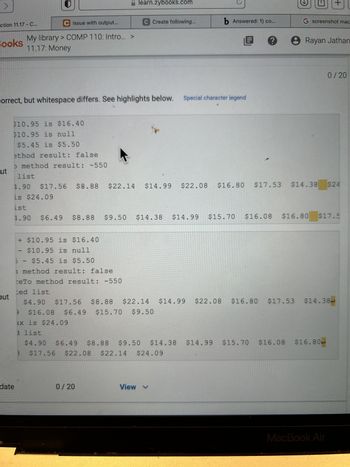
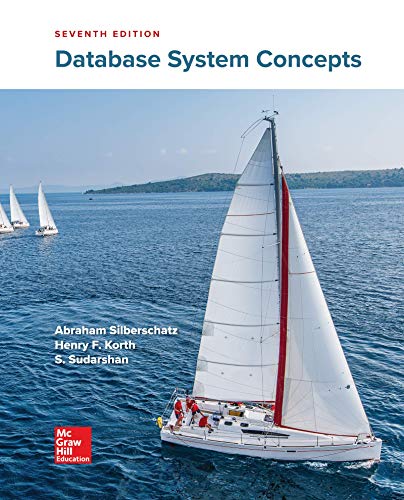
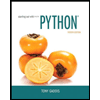
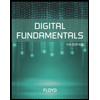
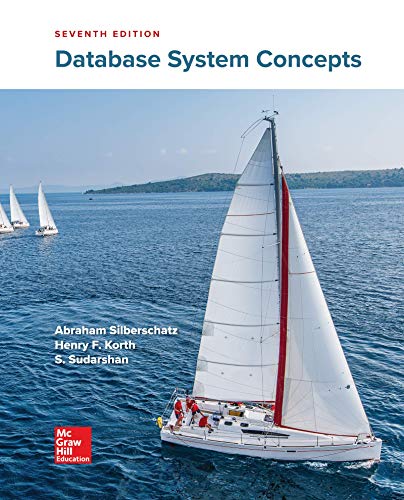
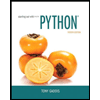
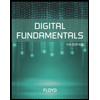
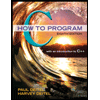
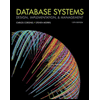
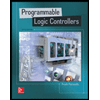