IN JAVA Look at the following class definition: public class Dog { private int age = 0; // age is given in years, the default value is 0 private String name = "Fido"; // default name is Fido Dog(String firstName, int firstAge) { name = firstName; age = firstAge; if (age < 0) { age = 0; } } public void setName(String newName) { name = newName; } public int getAge() { return age; } public String getName() { return name; } public void haveBirthdayParty() { age = age + 1; System.out.println("Happy birthday " + name + "! You are now " + age + " years old!"); } public void printInfo() { System.out.println("This dog's name is " + name + " and he's " + age + " years old!"); } } Make a post containing your answers to all of the questions below: Can you create a new dog without specifying its name? Why or why not? Which of the Dog class's methods are accessors? Which ones are mutators? Can you use the methods provided to make a dog younger? (In other words, can you set age to a lower value than it already was?) When you create a new dog, what happens if you try to set its age to a negative value? Complete the main method below by writing code underneath each comment (assume the program has access to the Dog class). public static void main(String[] args) { // Put together a new Dog object. You can give it any name you want, but the age must be 3 // Call the printInfo method to print the dog's information // Call the haveBirthdayParty method so the dog becomes 4 years old }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
IN JAVA
Look at the following class definition:
public class Dog {
private int age = 0; // age is given in years, the default value is 0
private String name = "Fido"; // default name is Fido
Dog(String firstName, int firstAge) {
name = firstName;
age = firstAge;
if (age < 0) {
age = 0;
}
}
public void setName(String newName) {
name = newName;
}
public int getAge() {
return age;
}
public String getName() {
return name;
}
public void haveBirthdayParty() {
age = age + 1;
System.out.println("Happy birthday " + name + "! You are now " + age + " years old!");
}
public void printInfo() {
System.out.println("This dog's name is " + name + " and he's " + age + " years old!");
}
}
Make a post containing your answers to all of the questions below:
- Can you create a new dog without specifying its name? Why or why not?
- Which of the Dog class's methods are accessors? Which ones are mutators?
- Can you use the methods provided to make a dog younger? (In other words, can you set age to a lower value than it already was?)
- When you create a new dog, what happens if you try to set its age to a negative value?
- Complete the main method below by writing code underneath each comment (assume the program has access to the Dog class).
public static void main(String[] args) {
// Put together a new Dog object. You can give it any name you want, but the age must be 3
// Call the printInfo method to print the dog's information
// Call the haveBirthdayParty method so the dog becomes 4 years old
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

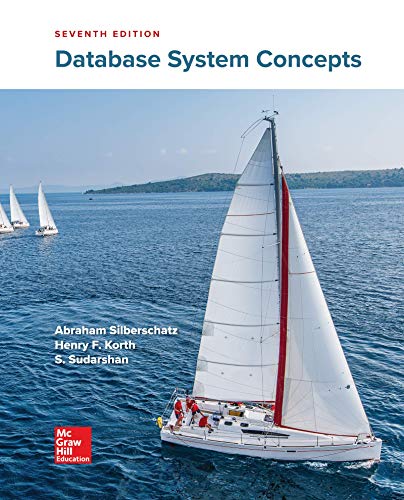
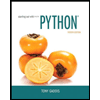
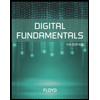
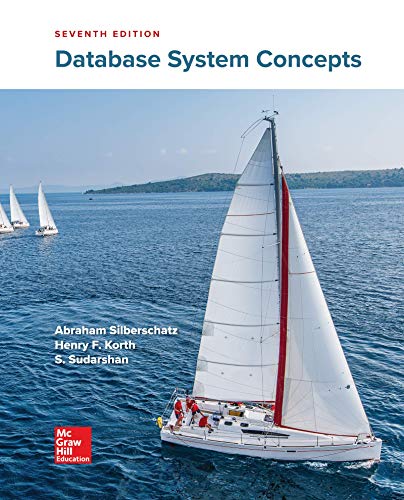
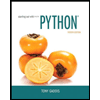
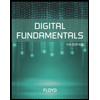
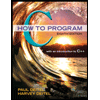
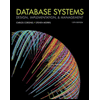
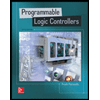