I have the following code: class Money { private int dollar; private int cent; final static int MIN_CENT_VALUE=0; final static int MAX_CENT_VALUE=99; public Money(){ this.dollar=0; this.cent=0; } public Money(int dollar,int cent){ this.dollar=dollar; if(cent>=MIN_CENT_VALUE&¢<=MAX_CENT_VALUE){ this.cent=cent; }else{ cent=0; } } public int getDollar(){ return this.dollar; } public void setDollar(int dol){ this.dollar=dol; } public int getCent(){ return this.cent; } public void setCent(int c){ if(c>=MIN_CENT_VALUE&&c<=MAX_CENT_VALUE){ this.cent=c; } } public Money add(Money otherMoney){ this.dollar+=otherMoney.dollar; this.cent+=otherMoney.cent; return this; } public Money subtract(Money otherMoney){ this.dollar-=otherMoney.dollar; this.cent-=otherMoney.cent; if(this.dollar<0||this.cent<0){ return null; } return this; } public boolean equals(Money otherMoney){ return this==otherMoney; } public int compareTo(Money otherMoney){ if(this.dollar m){ for(int i=0;i
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I have the following code:
class Money {
private int dollar;
private int cent;
final static int MIN_CENT_VALUE=0;
final static int MAX_CENT_VALUE=99;
public Money(){
this.dollar=0;
this.cent=0;
}
public Money(int dollar,int cent){
this.dollar=dollar;
if(cent>=MIN_CENT_VALUE&¢<=MAX_CENT_VALUE){
this.cent=cent;
}else{
cent=0;
}
}
public int getDollar(){
return this.dollar;
}
public void setDollar(int dol){
this.dollar=dol;
}
public int getCent(){
return this.cent;
}
public void setCent(int c){
if(c>=MIN_CENT_VALUE&&c<=MAX_CENT_VALUE){
this.cent=c;
}
}
public Money add(Money otherMoney){
this.dollar+=otherMoney.dollar;
this.cent+=otherMoney.cent;
return this;
}
public Money subtract(Money otherMoney){
this.dollar-=otherMoney.dollar;
this.cent-=otherMoney.cent;
if(this.dollar<0||this.cent<0){
return null;
}
return this;
}
public boolean equals(Money otherMoney){
return this==otherMoney;
}
public int compareTo(Money otherMoney){
if(this.dollar<otherMoney.dollar){
return 1;
}else if(this.dollar==otherMoney.dollar){
return 0;
}else{
return -1;
}
}
public static void printList(Money[] list,int n)
{
for(int i=0;i<n;i++)
{
System.out.println(list[i].dollar+" "+list[i].cent);
}
}
public String toString(){
return "Dollar is "+this.dollar+" and cents is "+this.cent;
}
public static Money max(Money[] m,int n){
Money res= new Money();
res.dollar=0;
res.cent=0;
for(int i=0;i<n;i++){
if(res.dollar<m[i].dollar){
res=m[i];
}
}
return res;
}
public void publicList(ArrayList<Money> m){
for(int i=0;i<m.size();i++){
System.out.print(m.get(i)+" ");
}
}
public static void selectionSort(Money[] m,int n)
{
// Loop to increase the boundary of the sorted array
for (int i = 0; i < n-1; i++)
{
int min_element = i;
for (int j = i+1; j < n; j++)
if (m[j].dollar < m[min_element].dollar)
min_element = j;
else if((m[j].dollar==m[min_element].dollar) && (m[j].cent< m[min_element].cent))
min_element=j;
/* Swap the smallest element from the unsorted array with the last element of the sorted array */
Money temp = new Money();
temp=m[min_element];
m[min_element] = m[i];
m[i] = temp;
}
}
}
_________________________________________
for use with this driver program, testMoney.java
import java.util.*;
public class TestMoney
{
public static void main(String[] args)
{
Random rnd = new Random(10);
Money m1 = new Money(5,45);
Money m2 = new Money(10,95);
m1.setCent(101);
Money sum = m1.add(m2);
System.out.println(m1+" + "+m2+" is "+sum);
Money diff = m1.subtract(m2);
System.out.println(m1+" - "+m2+" is "+diff);
diff = m2.subtract(m1);
System.out.println(m2+" - "+m1+" is "+diff);
System.out.println("equals method result: "+m1.equals(m2));
System.out.println("compareTo method result: "+m1.compareTo(m2));
Money[] list = new Money[15];
// Generate 15 random Money objects
for(int i=0;i<list.length;++i)
{
list[i] = new Money((int)(1+rnd.nextInt(30)),rnd.nextInt(100));
}
// print the list of Money objects, 10 per line
System.out.println("Unsorted list");
Money.printList(list,10);
// Find the largest Money object
System.out.println("The Max is "+Money.max(list));
// Sort and print
System.out.println("Sorted list");
Money.selectionSort(list);
Money.printList(list,10);
}
}
But I keep getting the following error messages:
![### Error Output Explanation
The code provided fails to compile due to several errors and a warning. Below is a detailed breakdown of these issues:
#### Errors:
1. **Error in Money.java at line 95:**
- **Message:** `cannot find symbol`
- **Problem:** The code is trying to use a class `ArrayList` which is not recognized.
- **Location:** The error is located in the `class Money`.
- **Potential Cause:** The `ArrayList` class might not be imported. Ensure `import java.util.ArrayList;` is included at the beginning of the file.
2. **Error in TestMoney.java at line 30:**
- **Message:** `method max in class Money cannot be applied to given types`
- **Details:** `System.out.println("The Max is " + Money.max(list));`
- **Required Type:** `Money[], int`
- **Found Type:** `Money[]`
- **Reason:** There is a mismatch in argument lists length between what the method expects and what is provided.
- **Solution:** Check the `max` method to ensure it matches its usage.
3. **Error in TestMoney.java at line 33:**
- **Message:** `method selectionSort in class Money cannot be applied to given types`
- **Details:** `Money.selectionSort(list);`
- **Required Type:** `Money[], int`
- **Found Type:** `Money[]`
- **Reason:** Similar to the previous error, there is a mismatch between expected and provided arguments.
- **Solution:** Verify the `selectionSort` method's parameters and adjust the call accordingly.
#### Warning:
1. **Warning in TestMoney.java at line 24:**
- **Message:** `[cast] redundant cast to int`
- **Code Segment:** `list[i] = new Money(((int)(1 + rnd.nextInt(30))), rnd.nextInt(100));`
- **Explanation:** The cast to `int` is unnecessary as `rnd.nextInt(30)` already returns an `int`.
- **Solution:** Remove the unnecessary cast for cleaner code.
#### Summary:
There are three main issues and one warning that need addressing:
- Ensure necessary imports (like `ArrayList`) are included.
- Check method definitions and their calls for argument mismatches.
- Remove redundant type casts.
Correcting these issues will](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e826cb-c133-47e8-aeaa-858e7b3a09da%2Fc65a444b-15d6-449e-9511-696bdb8dabe9%2Fihfjoc_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

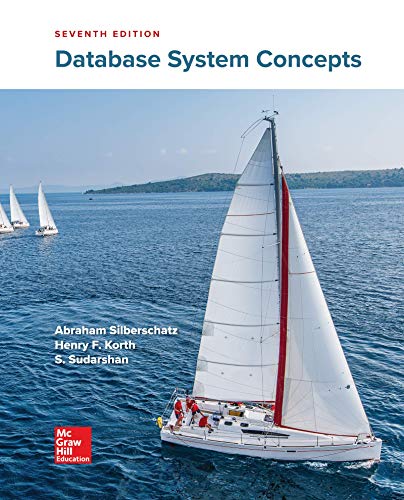
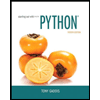
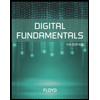
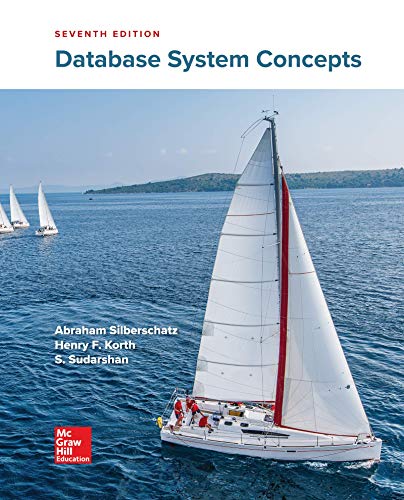
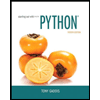
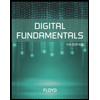
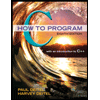
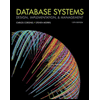
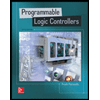