q1) provide commneted code for each line of code
mport java.util.Scanner;
public class ParkingCharges {
// function to calculate the basic charge using the asked hours
static double getBasicCharge(int hours) {
if (hours >= 7 && hours <= 8)
return 5.50;
else if (hours >= 5 && hours <= 6)
return 4.50;
else if (hours >= 2 && hours <= 4)
return 4.00;
return 3.00;
}
// function to return the amount to subtract based on local living and OAP
static double getDiscount(String isLocal, String isOAP) {
if (isOAP.equals("Yes") && isLocal.equals("Yes"))
return 2.0 + 1.0;
else if (isOAP.equals("Yes"))
return 2.0;
else if (isLocal.equals("Yes"))
return 1.0;
return 0;
}
public static void main(String[] args) {
// create a new Scanner object
Scanner sc = new Scanner(System.in);
// prompt the user to ask if they are disabled
System.out.print("Are you disabled? ");
String isDisabled = sc.nextLine();
// check if user entered 'Yes'
if (isDisabled.equals("Yes"))
System.out.println("Parking for you is free");
else {
// prompt the user for the number of hours
System.out.print("How many hous do you wish to park (1-8)? ");
int hours = sc.nextInt();
// prompt the user to ask if they have a "I live locally badge"
System.out.print("Do you have an \"I live locally badge\"? ");
sc.nextLine(); // consume the newline in the input stream
String isLocal = sc.nextLine();
// prompt the user to ask if they are an OAP
System.out.print("Are you an OAP? ");
String isOAP = sc.nextLine();
// call the getBasicCharge() method
double basicCharge = getBasicCharge(hours);
// update the amount based on the discount
double finalCharge = basicCharge - getDiscount(isLocal, isOAP);
System.out.println("The parking charge for you is " + finalCharge + " pounds.");
}
// close the Scanner object
sc.close();
}
}
q1) provide commneted code for each line of code

Step by step
Solved in 2 steps with 2 images

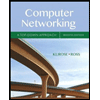
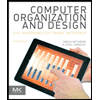
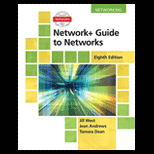
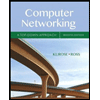
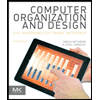
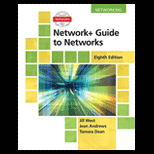
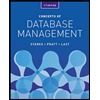
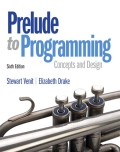
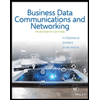