// Simulates a simple car with operations to drive and check the odometer. public class SimpleCar { // Number of miles driven private int miles; public SimpleCar(){ miles = 0; } public void drive(int dist){ miles = miles + dist; }
// Simulates a simple car with operations to drive and check the odometer. public class SimpleCar { // Number of miles driven private int miles; public SimpleCar(){ miles = 0; } public void drive(int dist){ miles = miles + dist; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
// Simulates a simple car with operations to drive and check the odometer.
public class SimpleCar {
// Number of miles driven
private int miles;
public SimpleCar(){
miles = 0;
}
public void drive(int dist){
miles = miles + dist;
}
public void reverse(int dist){
miles = miles - dist;
}
public int getOdometer(){
return miles;
}
public void honkHorn(){
System.out.println("beep beep");
}
public void report(){
System.out.println("Car has driven: " + miles + " miles");
}
}
![**LAB ACTIVITY**
**26.5.1: LAB: Simple car**
**Current file: LabProgram.java**
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
/* Type your code here. */
}
}
```
### Explanation
This Java program snippet sets up the starting framework for a lab activity titled "Simple car." The program uses `java.util.Scanner` to handle input from the user.
- **Line 1**: Imports the `Scanner` class from the `java.util` package, allowing the program to read input.
- **Line 3**: Declares a public class named `LabProgram`.
- **Line 4**: Defines the `main` method, which serves as the entry point for the Java application. It accepts a string array `args` as an argument.
- **Line 5**: Initializes a `Scanner` object named `scnr` to read from the standard input stream (`System.in`).
- **Lines 7-8**: Comments provide a placeholder for where additional code should be typed.
This basic structure is ideal for building programs that require user input.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2Ff1b50906-fa1a-47a9-815e-1f4e3d1ccb27%2F8lbfym_processed.png&w=3840&q=75)
Transcribed Image Text:**LAB ACTIVITY**
**26.5.1: LAB: Simple car**
**Current file: LabProgram.java**
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
/* Type your code here. */
}
}
```
### Explanation
This Java program snippet sets up the starting framework for a lab activity titled "Simple car." The program uses `java.util.Scanner` to handle input from the user.
- **Line 1**: Imports the `Scanner` class from the `java.util` package, allowing the program to read input.
- **Line 3**: Declares a public class named `LabProgram`.
- **Line 4**: Defines the `main` method, which serves as the entry point for the Java application. It accepts a string array `args` as an argument.
- **Line 5**: Initializes a `Scanner` object named `scnr` to read from the standard input stream (`System.in`).
- **Lines 7-8**: Comments provide a placeholder for where additional code should be typed.
This basic structure is ideal for building programs that require user input.

Transcribed Image Text:Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations:
- Drives input number of miles forward
- Drives input number of miles in reverse
- Honks the horn
- Reports car status
The `SimpleCar` class is found in the file `SimpleCar.java`.
Ex: If the input is:
```
100 4
```
the output is:
```
beep beep
Car has driven: 96 miles
```
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
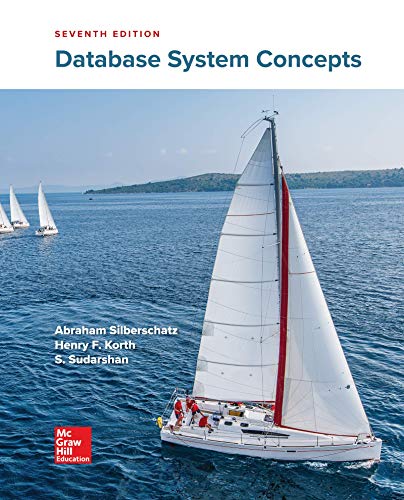
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
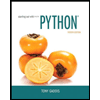
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
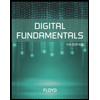
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
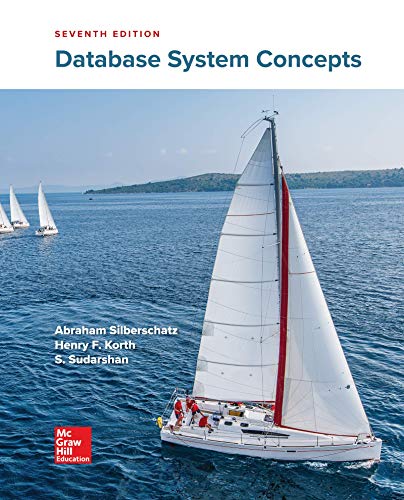
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
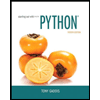
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
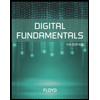
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
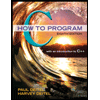
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
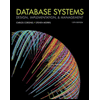
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
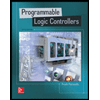
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education