1 a. is the amount the same, yes or no, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } b. public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here } } c. is the balance the same? yes or no, why? class CheckingAct { . . . . private int balance; public void processCheck( int amount ) { int charge; if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // change the local copy of the value in "amount" amount = 0 ; } } public class CheckingTester { public static void main ( String[] args ) { CheckingAct act; int check = 5000; act = new CheckingAct( "123-345-99", "Your Name", 100000 ); System.out.println( "check:" + check ); // prints "5000" // call processCheck with a copy of the value 5000 act.processCheck( check ); System.out.println( "check:" + check ); // prints "5000" --- "check" was not changed } }
1
a. is the amount the same, yes or no, why?
public class CheckingAct
{
private String actNum;
private String nameOnAct;
private int balance;
. . . .
public void processDeposit( int amount )
{
balance = balance + amount ;
}
// modified toString() method
public String toString()
{
return "Account: " + actNum + "\tName: " + nameOnAct +
"\tBalance: " + amount ;
}
}
b.
public class CheckingAct
{
private String actNum;
private String nameOnAct;
private int balance;
. . . .
public void processDeposit( int amount )
{ // scope of amount starts here
balance = balance + amount ;
// scope of amount ends here
}
public void processCheck( int amount )
{ // scope of amount starts here
int charge;
incrementUse();
if ( balance < 100000 )
charge = 15;
else
charge = 0;
balance = balance - amount - charge ;
// scope of amount ends here
}
}
c. is the balance the same? yes or no, why?
class CheckingAct
{
. . . .
private int balance;
public void processCheck( int amount )
{
int charge;
if ( balance < 100000 )
charge = 15;
else
charge = 0;
balance = balance - amount - charge ;
// change the local copy of the value in "amount"
amount = 0 ;
}
}
public class CheckingTester
{
public static void main ( String[] args )
{
CheckingAct act;
int check = 5000;
act = new CheckingAct( "123-345-99", "Your Name", 100000 );
System.out.println( "check:" + check ); // prints "5000"
// call processCheck with a copy of the value 5000
act.processCheck( check );
System.out.println( "check:" + check ); // prints "5000" --- "check" was not changed
}
}
d. is the amount and the balance the same? yes or no, why?
class CheckingAct
{
. . . .
private int balance;
public void processCheck( int amount )
{
int amount;
incrementUse();
if ( balance < 100000 )
amount = 15; // which amount ???
else
amount = 0; // which amount ???
balance = balance - amount ; // which amount ???
}
}
e. balance the same, y, n, why?
class CheckingAct
{
. . . .
private int balance;
. . . .
public void processDeposit( int amount )
{
int balance = 0; // New declaration of balance.
balance = balance + amount ; // This uses the local variable, balance.
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

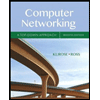
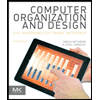
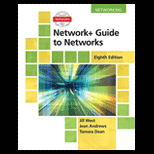
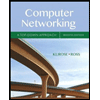
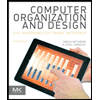
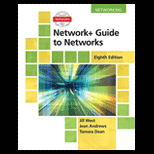
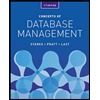
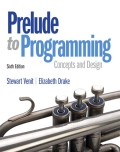
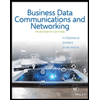