Python Programming only please no java Create a PollMachine class that can be used for a simple poll for users. Your program must perform the following actions: Method to clear the machine state Method to agree/Disagree to 3 options of your choice for example (I enjoy being a student Agree(A)/Disagree(D)) Get tallies of all agrees and disagrees for each answer Keep asking for users to enter answers until the user ends the program. Print the following to the console: Display the results after the poll has ended. Make sure to use a try-except block and include the finally block. Describe the data you print. Do not just print numbers to the screen explain what each number represents.
Python
Create a PollMachine class that can be used for a simple poll for users.
Your program must perform the following actions:
- Method to clear the machine state
- Method to agree/Disagree to 3 options of your choice for example (I enjoy being a student Agree(A)/Disagree(D))
- Get tallies of all agrees and disagrees for each answer
Keep asking for users to enter answers until the user ends the program.
Print the following to the console:
-
Display the results after the poll has ended.
-
Make sure to use a try-except block and include the finally block.
Describe the data you print. Do not just print numbers to the screen explain what each number represents.

Code:-
import sys
def userInput(talliesYes, talliesNo):
print('Enter your name')
user = input()
try:
print('you like a student! Press Y for yes and N for no')
var = input()
if var == 'Y':
talliesYes += 1
elif var == 'N':
talliesNo += 1
else:
raise Exception('Invalid the Input!!')
print('you enjoy the courses you have taken so far! Press Y for yes and N for no')
var = input()
if var == 'Y':
talliesYes += 1
elif var == 'N':
talliesNo += 1
else:
raise Exception('Invalid Input!!')
print(' you undertaken any part-time jobs! Press Y for yes and N for no')
var = input()
if var == 'Y':
talliesYes += 1
elif var == 'N':
talliesNo += 1
else:
raise Exception('Invalid Input!!')
print(user +', you\'ve reached the end of the poll. Press Q to quit')
var = input()
if var == 'Q':
sys.exit()
else:
raise Exception('Invalid Input!!')
except:
print('Something went wrong during polling!!')
finally:
print('The poll for user '+user+' are: No. of affirmatives - '+str(talliesYes)+ ' No. of negations - '+ str(talliesNo))
userInput(0,0)
import os
def clearState():
command = 'clear'
if os.name in ('nt', 'dos'):
command = 'cls'
os.system(command)
clearState()
Step by step
Solved in 2 steps with 3 images

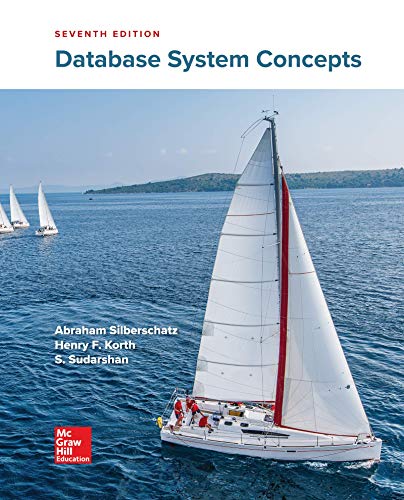
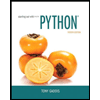
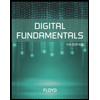
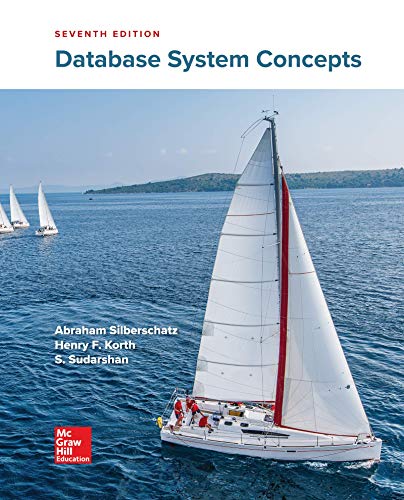
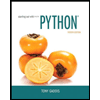
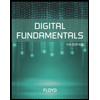
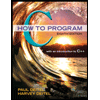
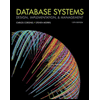
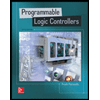