Given a GVDie object and an integer that represents the total sum desired as parameters, complete method rollTotal() in LabProgram class that returns the number of rolls needed to achieve at least the total sum. Note: For testing purposes, the GVDie object is created in the main() method using a pseudo-random number generator with a fixed seed value. The program uses a seed value of 15 during development, but when submitted, a different seed value will be used for each test case. Ex: If the GVDie object is created with a seed value of 15 and the input of the program is: 20 the output is: Number of rolls to reach at least 20: 6
Given a GVDie object and an integer that represents the total sum desired as parameters, complete method rollTotal() in LabProgram class that returns the number of rolls needed to achieve at least the total sum. Note: For testing purposes, the GVDie object is created in the main() method using a pseudo-random number generator with a fixed seed value. The program uses a seed value of 15 during development, but when submitted, a different seed value will be used for each test case. Ex: If the GVDie object is created with a seed value of 15 and the input of the program is: 20 the output is: Number of rolls to reach at least 20: 6
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
import java.util.*;
public class GVDie implements Comparable {
private int myValue;
private Random rand;
public GVDie() {
// set default values
myValue = (int) (Math.random()*6)+1;
rand = new Random();
}
public void roll (){
myValue = rand.nextInt(6) + 1;
}
public int getValue(){
return myValue;
}
// set the random number generator seed for testing
public void setSeed(int seed){
rand.setSeed(seed);
}
// allows dice to be compared if necessary
public int compareTo(Object o){
GVDie d = (GVDie) o;
return getValue() - d.getValue();
}
}

Transcribed Image Text:### Educational Content: Rolling Dice Simulation
**Objective:**
Develop a method in the `LabProgram` class to determine the number of dice rolls needed to reach a specified total sum using a GVDice object.
**Instructions:**
- You are provided with a GVDice object and an integer representing the target total sum.
- Your task is to complete the method `rollTotal()` so that it returns the number of rolls required to achieve at least the target sum.
**Important Note:**
- The GVDice object is created within the `main()` method, utilizing a pseudo-random number generator with a fixed seed value for development. This allows for consistent testing results.
- Although a seed value of 15 is set during development, it is essential to note that a different seed value will be employed for each test case upon submission.
**Example Usage:**
Suppose the GVDice object is initialized with a seed value of 15, and the program's input is:
```
20
```
**Expected Output:**
The program should output:
```
Number of rolls to reach at least 20: 6
```
This indicates it takes 6 rolls to accumulate a total sum of at least 20.
**Conclusion:**
This exercise aims to enhance your understanding of random number generation and the application of loops in achieving a specified target sum through multiple iterative processes.
![**Lab Activity: 26.10.1 - How many dice rolls?**
**Current File: LabProgram.java**
```java
import java.util.Scanner;
public class LabProgram {
public static int rollTotal(GVDie d, int total) {
/* Type your code here. */
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
GVDie die = new GVDie(); // Create a GVDie object
die.setSeed(15); // Set the GVDie object with seed value 15
int total;
int rolls;
total = scnr.nextInt();
rolls = rollTotal(die, total); // Should return the number of rolls to reach total.
System.out.println("Number of rolls to reach at least " + total + ": " + rolls);
}
}
```
---
### Explanation:
This Java program is designed to simulate dice rolls until a specified total is reached. It includes a main method for execution and a `rollTotal` method that needs to be implemented.
- **Imports**: The program imports `java.util.Scanner`, which is used to handle user input.
- **Classes and Methods**:
- `LabProgram`: This is the main class that contains the program logic.
- `rollTotal`: A static method that is intended to calculate the number of dice rolls required to reach a given total. The implementation needs to be completed.
- **Main Method**:
- Initializes a `Scanner` object to receive user input.
- Initializes a `GVDie` object to simulate a dice. `GVDie` is presumably a class provided for dice simulation in this context.
- Sets a seed of 15 for the random number generator associated with the dice, ensuring consistent results.
- Reads an integer `total` from the input, which specifies the target sum of dice rolls.
- Calls the `rollTotal` method to determine how many rolls are needed to reach at least the specified total. The result is stored in the `rolls` variable.
- Outputs the number of rolls required.
The `rollTotal` method should include logic to:
- Continuously roll the dice.
- Accumulate the sum until the specified `total` is reached or exceeded.
- Count and return the number of rolls taken.
This exercise is likely part of a learning module on loops, conditionals](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2F7fe312d9-c7f3-422d-836b-e4bbf2647ea8%2F30ad6h_processed.png&w=3840&q=75)
Transcribed Image Text:**Lab Activity: 26.10.1 - How many dice rolls?**
**Current File: LabProgram.java**
```java
import java.util.Scanner;
public class LabProgram {
public static int rollTotal(GVDie d, int total) {
/* Type your code here. */
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
GVDie die = new GVDie(); // Create a GVDie object
die.setSeed(15); // Set the GVDie object with seed value 15
int total;
int rolls;
total = scnr.nextInt();
rolls = rollTotal(die, total); // Should return the number of rolls to reach total.
System.out.println("Number of rolls to reach at least " + total + ": " + rolls);
}
}
```
---
### Explanation:
This Java program is designed to simulate dice rolls until a specified total is reached. It includes a main method for execution and a `rollTotal` method that needs to be implemented.
- **Imports**: The program imports `java.util.Scanner`, which is used to handle user input.
- **Classes and Methods**:
- `LabProgram`: This is the main class that contains the program logic.
- `rollTotal`: A static method that is intended to calculate the number of dice rolls required to reach a given total. The implementation needs to be completed.
- **Main Method**:
- Initializes a `Scanner` object to receive user input.
- Initializes a `GVDie` object to simulate a dice. `GVDie` is presumably a class provided for dice simulation in this context.
- Sets a seed of 15 for the random number generator associated with the dice, ensuring consistent results.
- Reads an integer `total` from the input, which specifies the target sum of dice rolls.
- Calls the `rollTotal` method to determine how many rolls are needed to reach at least the specified total. The result is stored in the `rolls` variable.
- Outputs the number of rolls required.
The `rollTotal` method should include logic to:
- Continuously roll the dice.
- Accumulate the sum until the specified `total` is reached or exceeded.
- Count and return the number of rolls taken.
This exercise is likely part of a learning module on loops, conditionals
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
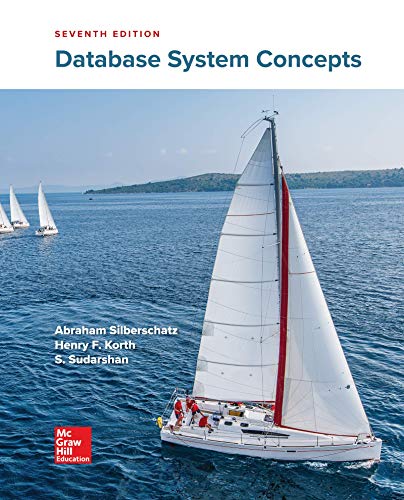
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
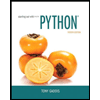
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
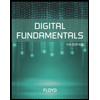
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
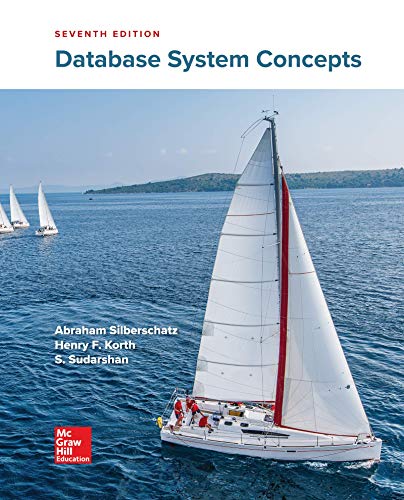
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
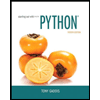
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
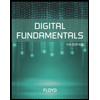
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
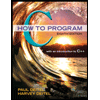
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
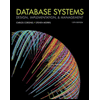
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
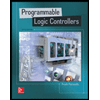
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education