Java Whats Wrong With my Code Line 20 ArrayList ? MAP Class package chapter21; import java.util.ArrayList; import java.util.TreeMap; public class Map { Employee employees1[] = { new Employee("12345", "Tom", "Baker", 200000), new Employee("56789", "Dan", "Jones", 130000), new Employee("24680", "Ann", "Scoot", 90000), new Employee("13579", "Pat", "Jones", 200000), new Employee("11111", "Bob", "Scott", 65000), new Employee("23232", "Scott", "Baker", 65000), new Employee("45454", "Amy", "Perez", 105000), new Employee("67676", "Jones", "BakwJoneser", 140000) }; // create an array list object that will hold data type = Employee ArrayList employeeList = new ArrayList <>(); // iterate the array and add the employee to the list for (Employee employee1 : employees) { //add employee1 to the employeeList employeeList.add(employee1); } System.out.println("All Employee\n"); // use and enhanced for loop to print the employees for (Employee employees : employeeList) { System.out.println(employee1); } // create a tree map with String and Empolyee Objects TreeMap employeeTreeMap = new TreeMap<>(); for(Employee employee:employeeList){ //use for lope to add the employees to the TreeSet using put() method to get the employees IDs and Name employeeTreeMap.put(employee1.getId(),employee1); } // print each employee sorted by employee id System.out.println("Employees By ID #\n"); //iterate the tree map for(String id: employeeTreeMap.keySet()){ //Print out employee names and ID with Tree Map System.out.println(employeeTreeMap.get(id)); } } } Employee Class package chapter21; import java.text.DecimalFormat; import java.text.NumberFormat; public class Employee { //Attributes (all private): private String id; private String lastName; private String firstName; private int salary; // Methods Employee (String id, String lastName, String firstName, int salary) { this.id = id; //pts to the data that is put into the constructor this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Getters for all attributes public String getId() { //creates a method to return the data return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } // toString method to display an Employee's attributes (See output of executable below) //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to format the salary to desired output. //"ID 23232:Baker, Amy, salary $100,000" // "ID 12345:Jones, Dan, salary $200,000" //Number froamt returns a currency format NumberFormat nfm = NumberFormat.getCurrencyInstance(); // String pt = ((DecimalFormat) nfm).toPattern(); // String npt = pt.replace("\u00A4", "").trim(); //create varaible to hold decimal NumberFormat nfm2 = new DecimalFormat(npt); //set to setMinimum to round up or down for miney format nfm2.setMinimumFractionDigits(0); //return //"ID + 23232 + : + Baker,+ " , " + Amy +, salary $+ 100,000" return "ID " + id + ":" + lastName + ", " + firstName + ", salary $" + nfm2.format(salary); } }
Java
Whats Wrong With my Code Line 20 ArrayList ?
MAP Class
package chapter21;
import java.util.ArrayList;
import java.util.TreeMap;
public class Map {
Employee employees1[] = {
new Employee("12345", "Tom", "Baker", 200000),
new Employee("56789", "Dan", "Jones", 130000),
new Employee("24680", "Ann", "Scoot", 90000),
new Employee("13579", "Pat", "Jones", 200000),
new Employee("11111", "Bob", "Scott", 65000),
new Employee("23232", "Scott", "Baker", 65000),
new Employee("45454", "Amy", "Perez", 105000),
new Employee("67676", "Jones", "BakwJoneser", 140000)
};
// create an array list object that will hold data type = Employee
ArrayList<Employee> employeeList = new ArrayList <>();
// iterate the array and add the employee to the list
for (Employee employee1 : employees) {
//add employee1 to the employeeList
employeeList.add(employee1);
}
System.out.println("All Employee\n");
// use and enhanced for loop to print the employees
for (Employee employees : employeeList) {
System.out.println(employee1);
}
// create a tree map with String and Empolyee Objects
TreeMap<String,Employee> employeeTreeMap = new TreeMap<>();
for(Employee employee:employeeList){
//use for lope to add the employees to the TreeSet using put() method to get the employees IDs and Name
employeeTreeMap.put(employee1.getId(),employee1);
}
// print each employee sorted by employee id
System.out.println("Employees By ID #\n");
//iterate the tree map
for(String id: employeeTreeMap.keySet()){
//Print out employee names and ID with Tree Map
System.out.println(employeeTreeMap.get(id));
}
}
}
Employee Class

Step by step
Solved in 6 steps with 3 images

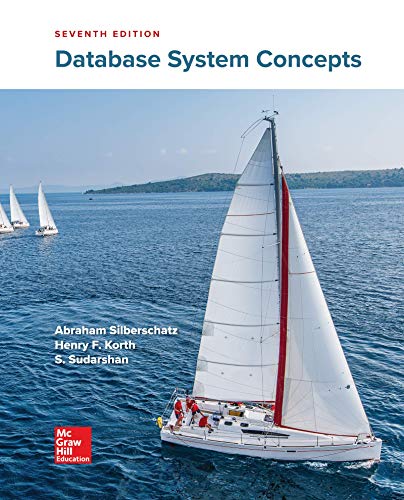
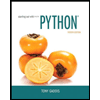
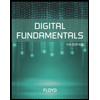
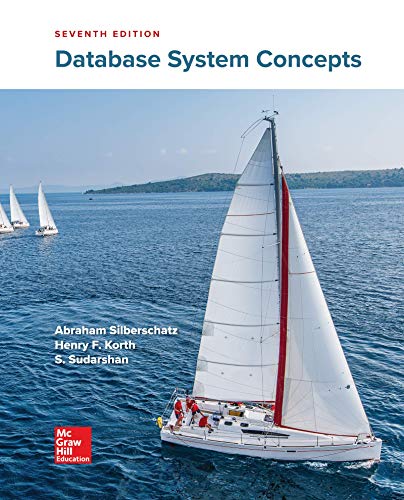
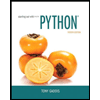
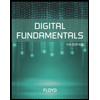
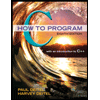
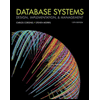
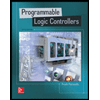