I am trying to program a hangman game in C++ using Dev-C++, complete with an ASCII-based main menu. This is the code that I have so far: //What is this code and what is it doing? #include // What do each of these do? #include #include using namespace std; //What is this code doing? int NUM_TRY = 7; int checkGuess (char, string, string); void menu(); string message = "Play!"; //What is this code doing? int main(int argc, char *argv[]) { string name; char letter; string animal; string animals[] = { "owl", "bear", "ocelot", "whale", "zebra", "armadillo", "chimpanzee", "alligator", "seahorse", "feline", "bovine", "horse", "canine" }; //What is this code doing? srand(time(NULL)); int n=rand()% 12; animal=animals[n]; string hide_m(animal.length(),'X'); //What is this code and what is it doing? while (NUM_TRY!=0) { menu(); cout << "\n\n\t\t\t\t" << hide_m; cout << "\n\n\t\t\t\tGuess a letter: "; cin >> letter; if (checkGuess(letter, animal, hide_m)==0) { message = "Incorrect letter."; NUM_TRY = NUM_TRY - 1; } else { message = "NICE! You guessed a letter"; } if (animal==hide_m) { message = "Congratulations! You got it!"; menu(); cout << "\n\t\t\t\tThe animal is : " << animal << endl; break; } } if(NUM_TRY == 0) { message = "No!! You've been hanged."; menu(); cout << "\n\t\t\t\tThe animal was : " << animal << endl; } cin.ignore(); cin.get(); return 0; } //What is this code and what is it doing? int checkGuess (char guess, string secretanimal, string &guessanimal) { int i; int matches=0; int len=secretanimal.length(); for (i = 0; i < len; i++) { if (guess == guessanimal[i]) return 0; if (guess == secretanimal[i]) { guessanimal[i] = guess; matches++; } } return matches; } menu{( //What is this code and what is it doing? system("color 05"); //change the color! system("cls"); cout << "\t\t\t\t*\t*"; cout<<"\t\t\t\t**\t**"; cout<<"\t\t\t\t***\t***"; cout<<"\t\t\t\t****\t****"; cout<<"\t\t\t\t*****\t*****"; cout<<"\t\t\t\t******\t******"; cout<<"\t\t\t\t*******\t*******"; cout<<"\t\t\t\t*******\t*******"; cout<<"\t\t\t\t******\t******"; cout<<"\t\t\t\t*****\t*****"; cout<<"\t\t\t\t****\t****"; cout<<"\t\t\t\t***\t***"; cout<<"\t\t\t\t**\t**"; cout<<"\t\t\t\t*\t*"; //What is this code and what is it doing? cout<<"\t\t@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@"; cout << "\n\t\t\t\tHangman Game!"; //Feel free to change the wording cout << "\n\t\tYou have " << NUM_TRY << " tries to try and guess the animal."; cout << "\n\n\t\t\t\t" + message; cout<<"\n\t\t@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@\n"; )} I cannot get the code to compile because of ONE error. Some help would be greatly appreciated.
I am trying to program a hangman game in C++ using Dev-C++, complete with an ASCII-based main menu. This is the code that I have so far:
//What is this code and what is it doing?
#include <cstdlib> // What do each of these do?
#include <ctime>
#include <iostream>
using namespace std;
//What is this code doing?
int NUM_TRY = 7;
int checkGuess (char, string, string);
void menu();
string message = "Play!";
//What is this code doing?
int main(int argc, char *argv[])
{
string name;
char letter;
string animal;
string animals[] =
{
"owl",
"bear",
"ocelot",
"whale",
"zebra",
"armadillo",
"chimpanzee",
"alligator",
"seahorse",
"feline",
"bovine",
"horse",
"canine"
};
//What is this code doing?
srand(time(NULL));
int n=rand()% 12;
animal=animals[n];
string hide_m(animal.length(),'X');
//What is this code and what is it doing?
while (NUM_TRY!=0)
{
menu();
cout << "\n\n\t\t\t\t" << hide_m;
cout << "\n\n\t\t\t\tGuess a letter: ";
cin >> letter;
if (checkGuess(letter, animal, hide_m)==0)
{
message = "Incorrect letter.";
NUM_TRY = NUM_TRY - 1;
}
else
{
message = "NICE! You guessed a letter";
}
if (animal==hide_m)
{
message = "Congratulations! You got it!";
menu();
cout << "\n\t\t\t\tThe animal is : " << animal << endl;
break;
}
}
if(NUM_TRY == 0)
{
message = "No!! You've been hanged.";
menu();
cout << "\n\t\t\t\tThe animal was : " << animal << endl;
}
cin.ignore();
cin.get();
return 0;
}
//What is this code and what is it doing?
int checkGuess (char guess, string secretanimal, string &guessanimal)
{
int i;
int matches=0;
int len=secretanimal.length();
for (i = 0; i < len; i++)
{
if (guess == guessanimal[i])
return 0;
if (guess == secretanimal[i])
{
guessanimal[i] = guess;
matches++;
}
}
return matches;
}
menu{(
//What is this code and what is it doing?
system("color 05"); //change the color!
system("cls");
cout << "\t\t\t\t*\t*";
cout<<"\t\t\t\t**\t**";
cout<<"\t\t\t\t***\t***";
cout<<"\t\t\t\t****\t****";
cout<<"\t\t\t\t*****\t*****";
cout<<"\t\t\t\t******\t******";
cout<<"\t\t\t\t*******\t*******";
cout<<"\t\t\t\t*******\t*******";
cout<<"\t\t\t\t******\t******";
cout<<"\t\t\t\t*****\t*****";
cout<<"\t\t\t\t****\t****";
cout<<"\t\t\t\t***\t***";
cout<<"\t\t\t\t**\t**";
cout<<"\t\t\t\t*\t*";
//What is this code and what is it doing?
cout<<"\t\t@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@";
cout << "\n\t\t\t\tHangman Game!"; //Feel free to change the wording
cout << "\n\t\tYou have " << NUM_TRY << " tries to try and guess the animal.";
cout << "\n\n\t\t\t\t" + message;
cout<<"\n\t\t@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@\n";
)}
I cannot get the code to compile because of ONE error. Some help would be greatly appreciated.

Step by step
Solved in 4 steps with 4 images

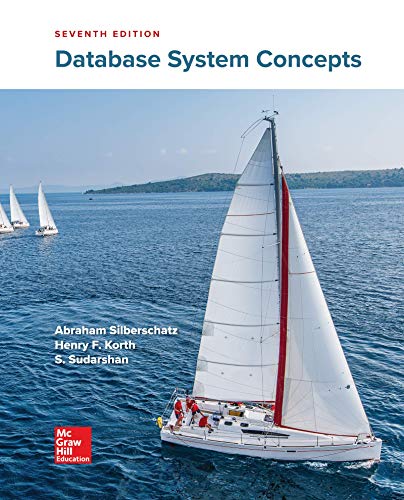
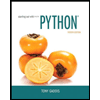
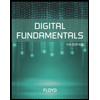
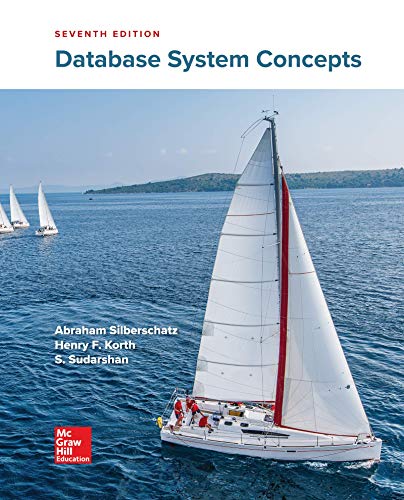
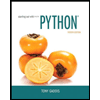
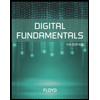
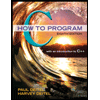
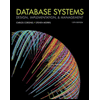
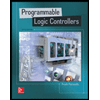