Fix this code so that it follows the following instructions. #include #include #include #include #include #include #include std::vector loadWordList(const std::string& filename) { std::vector wordList; std::ifstream file(filename); std::string line; while (std::getline(file, line)) { std::istringstream iss(line); std::string number, word; if (iss >> number >> word) { wordList.push_back(word); } } return wordList; } std::string generatePassphrase(const std::vector& wordList, int numWords) { std::string passphrase; int wordListSize = wordList.size(); for (int i = 0; i < numWords; ++i) { int index = rand() % wordListSize; passphrase += wordList[index] + " "; } return passphrase; } int main() { std::string filename = "diceware_wordlist.txt"; // Update with the correct filename int numWords; std::cout << "Diceware Passphrase Generator\n"; std::cout << "Enter the number of words for your passphrase: "; std::cin >> numWords; std::vector wordList = loadWordList(filename); // Seed the random number generator srand(static_cast(time(0))); std::string passphrase = generatePassphrase(wordList, numWords); std::cout << "Generated passphrase: " << passphrase << std::endl; return 0; } Suppose you want a six word passphrase, as we recommend for most users. You will need 6 times 5 or 30 dice rolls. Let's say they come out as: 1, 6, 6, 6, 5, 1, 5, 6, 5, 3, 5, 6, 3, 2, 2, 3, 5, 6, 1, 6, 6, 5, 2, 2, 4, 6, 4, 3, 2, and 6. Write down the results on a scrap of paper in groups of five rolls: 1 6 6 6 5 1 5 6 5 3 5 6 3 2 2 3 5 6 1 6 6 5 2 2 4 6 4 3 2 6 You then look up each group of five rolls in the Diceware word list by finding the number in the list and writing down the word next to the number: 1 6 6 6 5 cleft 1 5 6 5 3 cam 5 6 3 2 2 synod 3 5 6 1 6 lacy 6 5 2 2 4 yr 6 4 3 2 6 wok Your passphrase would then be: cleft cam synod lacy yr wok Components: ● Program will have an interface that explains its operation and is clear for users ● Program reads in the word list from a file ● Program will ask the user for the number of words for a pass phrase and generate it Suggested Possible Enhancements: · Regular password generation · Passphrase enhancements (special character inclusion, capitalization) · Passphrase strength measurement · Animation or visualization of generation · Use of colour · Add more languages
Fix this code so that it follows the following instructions.
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <
#include <cstdlib>
#include <ctime>
std::vector<std::string> loadWordList(const std::string& filename) {
std::vector<std::string> wordList;
std::ifstream file(filename);
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
std::string number, word;
if (iss >> number >> word) {
wordList.push_back(word);
}
}
return wordList;
}
std::string generatePassphrase(const std::vector<std::string>& wordList, int numWords) {
std::string passphrase;
int wordListSize = wordList.size();
for (int i = 0; i < numWords; ++i) {
int index = rand() % wordListSize;
passphrase += wordList[index] + " ";
}
return passphrase;
}
int main() {
std::string filename = "diceware_wordlist.txt"; // Update with the correct filename
int numWords;
std::cout << "Diceware Passphrase Generator\n";
std::cout << "Enter the number of words for your passphrase: ";
std::cin >> numWords;
std::vector<std::string> wordList = loadWordList(filename);
// Seed the random number generator
srand(static_cast<unsigned int>(time(0)));
std::string passphrase = generatePassphrase(wordList, numWords);
std::cout << "Generated passphrase: " << passphrase << std::endl;
return 0;
}
Suppose you want a six word passphrase, as we recommend for most users. You will need 6 times 5 or 30 dice rolls. Let's say they come out as:
1, 6, 6, 6, 5, 1, 5, 6, 5, 3, 5, 6, 3, 2, 2, 3, 5, 6,
1, 6, 6, 5, 2, 2, 4, 6, 4, 3, 2, and 6.
Write down the results on a scrap of paper in groups of five rolls:
1 6 6 6 5
1 5 6 5 3
5 6 3 2 2
3 5 6 1 6
6 5 2 2 4
6 4 3 2 6
You then look up each group of five rolls in the Diceware word list by finding the number in the list and writing down the word next to the number:
1 6 6 6 5 cleft
1 5 6 5 3 cam
5 6 3 2 2 synod
3 5 6 1 6 lacy
6 5 2 2 4 yr
6 4 3 2 6 wok
Your passphrase would then be:
cleft cam synod lacy yr wok
Components:
● Program will have an interface that explains its operation and is clear for users
● Program reads in the word list from a file
● Program will ask the user for the number of words for a pass phrase and generate it
Suggested Possible Enhancements:
· Regular password generation
· Passphrase enhancements (special character inclusion, capitalization)
· Passphrase strength measurement
· Animation or visualization of generation
· Use of colour
· Add more languages

Step by step
Solved in 3 steps

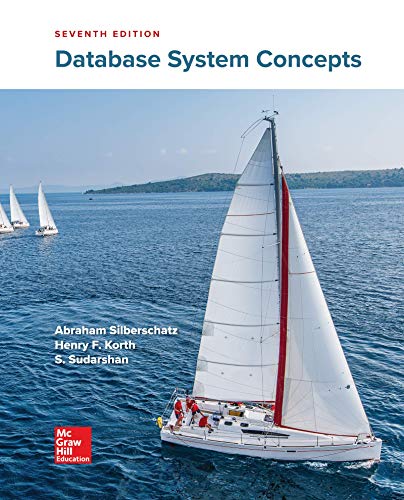
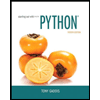
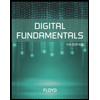
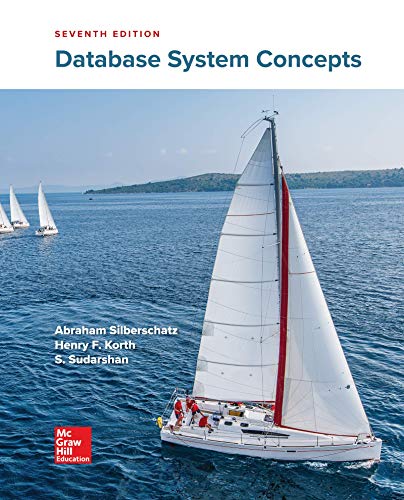
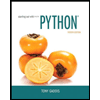
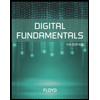
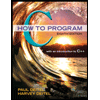
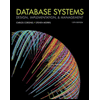
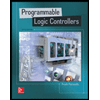