Hello, thank you C++ programming The runner file is attached as attachments(screenshot) Part 1 Work with inserting elements at the front of a vector and a deque Part 2 Work with inserting elements at the back of a vector and a deque Part 3 Work with inserting elements in the middle, and removing elements from, a vector and a deque
Hello, thank you C++ programming The runner file is attached as attachments(screenshot) Part 1 Work with inserting elements at the front of a vector and a deque Part 2 Work with inserting elements at the back of a vector and a deque Part 3 Work with inserting elements in the middle, and removing elements from, a vector and a deque
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Hello, thank you C++
The runner file is attached as attachments(screenshot)
Part 1
Work with inserting elements at the front of a
Part 2
Work with inserting elements at the back of a vector and a deque
Part 3
Work with inserting elements in the middle, and removing elements from, a vector and a deque

Transcribed Image Text:// You may not touch this code below
int main()
{
}
// Add some variability to the random number generator
srand (static_cast<unsigned int>(NULL));
// Work with the fronts of the vector and deque data structures
PartOne();
// Work with the backs of the vector and deque data structures
Part Two();
// Work with inserting and clearing the vector and deque data structures
PartThree();
// Cleanup
system("PAUSE");
return 0;

Transcribed Image Text:#include <iostream>
#include <deque>
#include <vector>
void PartOne()
{
//
// 1A
// PROPERLY SET UP VECTOR a WITH 500000 ELEMENTS IN ORDER 1, 2, 3, 4,
// PUT EACH ELEMENT IN THE FRONT AS YOU ADD THEM TO THE VECTOR
// -
std::vector<int> a;
}
//
// 1B
// PROPERLY SET UP DEQUE b WITH 500000 ELEMENTS IN ORDER 1, 2, 3, 4,
// PUT EACH ELEMENT IN THE FRONT AS YOU ADD THEM TO THE DEQUE
// - -
std::deque<int> b;
}
void Part Two ()
{
// 1C
// FULLY DESCRIBE WHICH OF THE TWO OPERATIONS IS FASTER THAN THE OTHER AND WHY
// EITHER USE A COMMENT, OR PRINT YOUR DESCRIPTION TO THE SCREEN
// UP TO 5 POINTS (MAXIMUM 10 OVERALL) EXTRA CREDIT IF YOU CAN SHOW EVIDENCE OF
// YOUR DESCRIPTION USING C++ CODE
//
11 -
// 2A
// SET UP VECTOR a WITH 500000 ELEMENTS IN ORDER 1, 2, 3, 4, ..., 500000
// PUT EACH ELEMENT IN THE BACK AS YOU ADD THEM TO THE VECTOR
// -
std::vector<int> a;
//
// 2B
// SET UP DEQUE b WITH 500000 ELEMENTS IN ORDER 1, 2, 3, 4,
// PUT EACH ELEMENT IN THE BACK AS YOU ADD THEM TO THE DEQUE
// -
std::deque<int> b;
}
void PartThree ()
{
500000
500000
// -
// 3A
500000
// 2C
// FULLY DESCRIBE WHICH OF THE TWO OPERATIONS IS FASTER THAN THE OTHER AND WHY
// EITHER USE A COMMENT, OR PRINT YOUR DESCRIPTION TO THE SCREEN
// UP TO 5 POINTS (MAXIMUM 10 OVERALL) EXTRA CREDIT IF YOU CAN SHOW EVIDENCE OF
// YOUR DESCRIPTION USING C++ CODE
// 1) INSERT RANDOMLY 5-10 VALUES OF 8 BETWEEN THE 2 AND THE 3 VALUE IN THE a VECTOR
// FOR EXAMPLE 1,2,3,4,5 BECOMES 1,2,8,8,8,8,8,8,3,4,5 (6 WAS RANDOMLY GENERATED)
// 2) EMPTY/CLEAR OUT THE VECTOR BY ONLY USING EITHER erase () OR pop_back ()
// -
std::vector<int> a { 1, 2, 3, 4, 5 };
//
// 3B
// 1) INSERT RANDOMLY 5-10 VALUES OF 8 BETWEEN THE 2 AND THE 3 VALUE IN THE b DEQUE
// FOR EXAMPLE 1,2,3,4,5 BECOMES 1,2,8,8,8,8,8,8,3,4,5 (6 WAS RANDOMLY GENERATED)
// 2) PROPERLY EMPTY/CLEAR OUT THE DEQUE
//
std::deque<int *> b { new int(1), new int(2), new int (3), new int (4), new int (5) };
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
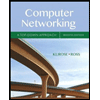
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
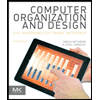
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
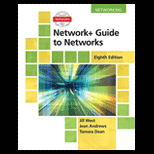
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
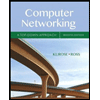
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
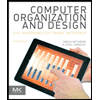
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
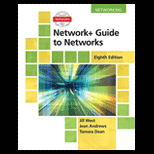
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
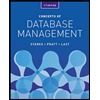
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
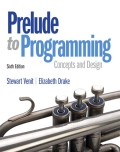
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
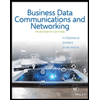
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY