in C++ /*your C++ code starts below ... Your code needs to 1. declare a new datatype struct StructuredPhoneNumber{ std::string areaCode="000"; //3 digits std::string prefix="000"; //3 digits std::string lineNo="0000"; // 4 digits }; 2. Use the following function prototype to implement your algorithm: //converts a phone number from a 10-digit long long to {area code, prefix, lineNo} /returns a StructuredPhoneNumber object {"000", "000", "0000"} if the input number is negative or does not contain 10 digits. StructuredPhoneNumber phoneNumBreakdown(long long phoneNum_ll); 3. include three unit tests test1( ), test2(), and test3(), each of which returns true if phoneNumBreakdown( ) correctly calculates the three piceces of info For example: bool test1() { //if you wish, you can print out a message here e.g., "my first test ...\n". //call phoneNumBreakdown(8005551212) ; //check the result } 4. overload the operator== to compare whether two StructuredPhoneNumber objects contain exactly the same phone number. 5. include two unit tests to test the above overloaded operator== bool test_operator1(){ //call the overloaded operator== //check if it produces the expected result } bool test_operator2(){ //call the overloaded operator== //check if it produces the expected result } */
in C++
/*your C++ code starts below ...
Your code needs to
1. declare a new datatype
struct StructuredPhoneNumber{
std::string areaCode="000"; //3 digits
std::string prefix="000"; //3 digits
std::string lineNo="0000"; // 4 digits
};
2. Use the following function prototype to implement your
//converts a phone number from a 10-digit long long to {area code, prefix, lineNo}
/returns a StructuredPhoneNumber object {"000", "000", "0000"} if the input number is negative or does not contain 10 digits.
StructuredPhoneNumber phoneNumBreakdown(long long phoneNum_ll);
3. include three unit tests test1( ), test2(), and test3(), each of which returns true
if phoneNumBreakdown( ) correctly calculates the three piceces of info
For example:
bool test1()
{
//if you wish, you can print out a message here e.g., "my first test ...\n".
//call phoneNumBreakdown(8005551212) ;
//check the result
}
4. overload the operator== to compare whether two StructuredPhoneNumber objects
contain exactly the same phone number.
5. include two unit tests to test the above overloaded operator==
bool test_operator1(){
//call the overloaded operator==
//check if it produces the expected result
}
bool test_operator2(){
//call the overloaded operator==
//check if it produces the expected result
}
*/

Here are the main tasks asked in the question,
Declare a New Data Type:
- Create a new type of data called
StructuredPhoneNumber
that can hold three pieces of information: area code, prefix, and line number for a phone number.
- Create a new type of data called
Create a Function:
- Write a function named
phoneNumBreakdown
that takes a long number (like a phone number) as input. This function will break down the number into its parts (area code, prefix, and line number) and return them in a specific format.
- Write a function named
Write Tests:
- Create three tests (test1, test2, and test3) to check if the
phoneNumBreakdown
function works correctly. These tests will check if the function can correctly split a phone number into its parts.
- Create three tests (test1, test2, and test3) to check if the
Compare Phone Numbers:
- Make a way to compare two sets of phone number parts (area code, prefix, and line number) to see if they are the same.
Write Tests for Comparison:
- Create two more tests (test_operator1 and test_operator2) to check if the comparison between two sets of phone number parts works correctly.
Display Results:
- Finally, run all these tests and display a message indicating whether everything is working correctly or if there are any problems. If all tests pass, it will say "All tests passed!" Otherwise, it will say "Tests failed!"
Step by step
Solved in 3 steps with 1 images

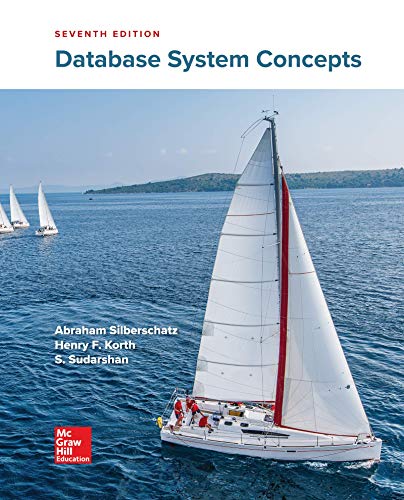
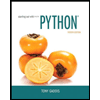
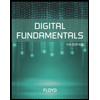
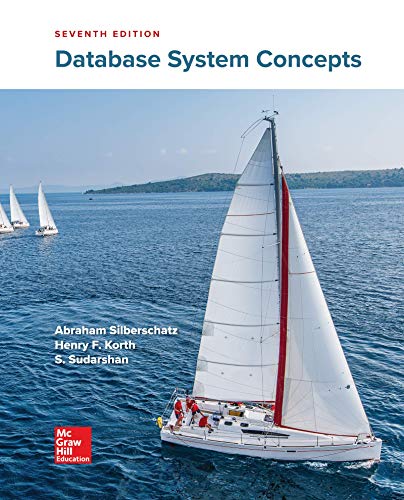
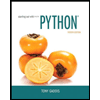
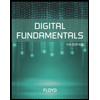
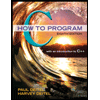
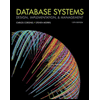
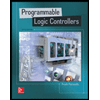