Create a flowchart for this program in c++, #include #include // for vectors #include #include // math for function like pow ,sin, log #include using std::vector; using namespace std; int main() { vector x, y;//vector x for x and y for y float x_tmp = -2.5; // initial value of x float my_function(float x); while (x_tmp <= 2.5) // the last value of x { x.push_back(x_tmp); y.push_back(my_function(x_tmp)); // calculate function's value for given x x_tmp += 1;// add step } cout << "my name's khaled , my variant is 21 ," << " my function is y = 0.05 * x^3 + 6sin(3x) + 4 " << endl; cout << "x\t"; for (auto x_tmp1 : x) cout << '\t' << x_tmp1;//printing x values with tops cout << endl; cout << "y\t"; for (auto y_tmp1 : y) cout << '\t' << y_tmp1;//printing y values with tops cout << endl; cout << "max value of function y for the given range is " << *max_element(y.begin(), y.end()) << endl; //calculate max and min using algorithm cout << "min value is " << *min_element(y.begin(), y.end()) << endl; float min_diff, value_spec = -2; cout << "Value of function y specified " << value_spec << endl; int i = 0, j = 0; // variables for indexes min_diff = abs(y[0] - value_spec); for (auto y_tmp1 : y) { if (abs(y_tmp1 - value_spec) <= min_diff)// we calculate absolute value of difference bettwen y and cerr { min_diff = abs(y_tmp1 - value_spec);// we caculate difference i = j;// we assign index for min value } ++j; } cout << "closet value of function y is : " << y[i] << " for x = " << x[i] << endl; // dont forget to include numeric cout << "Average value of function y for the given range is : " << accumulate(y.begin(), y.end(), 0, std::plus()) / float(y.size()) << endl; cout << "y change its sign" << endl; int num_changes = 0; for (int k = 1; k < y.size(); k++) { if ((y[k - 1] < 0 && y[k]>0) || (y[k - 1] > 0 && y[k] < 0)) { ++num_changes; cout << "change " << num_changes << endl; cout << "--------------" << endl; cout << "x =" << x[k - 1] << ", y =" << y[k - 1] << endl; cout << "x =" << x[k] << ", y =" << y[k] << endl; } } cout << "total number of time y changes its sign is " << num_changes; } float my_function(float x) { return 0.05 * pow(x, 3) + sin(3 * x) + 4; }
Create a flowchart for this program in c++,
#include <iostream>
#include <vector> // for
#include <
#include <cmath> // math for function like pow ,sin, log
#include <numeric>
using std::vector;
using namespace std;
int main()
{
vector <float> x, y;//vector x for x and y for y
float x_tmp = -2.5; // initial value of x
float my_function(float x);
while (x_tmp <= 2.5) // the last value of x
{
x.push_back(x_tmp);
y.push_back(my_function(x_tmp)); // calculate function's value for given x
x_tmp += 1;// add step
}
cout << "my name's khaled , my variant is 21 ," << " my function is y = 0.05 * x^3 + 6sin(3x) + 4 " << endl;
cout << "x\t";
for (auto x_tmp1 : x)
cout << '\t' << x_tmp1;//printing x values with tops
cout << endl;
cout << "y\t";
for (auto y_tmp1 : y)
cout << '\t' << y_tmp1;//printing y values with tops
cout << endl;
cout << "max value of function y for the given range is " << *max_element(y.begin(), y.end()) << endl; //calculate max and min using algorithm
cout << "min value is " << *min_element(y.begin(), y.end()) << endl;
float min_diff, value_spec = -2;
cout << "Value of function y specified " << value_spec << endl;
int i = 0, j = 0; // variables for indexes
min_diff = abs(y[0] - value_spec);
for (auto y_tmp1 : y)
{
if (abs(y_tmp1 - value_spec) <= min_diff)// we calculate absolute value of difference bettwen y and cerr
{
min_diff = abs(y_tmp1 - value_spec);// we caculate difference
i = j;// we assign index for min value
}
++j;
}
cout << "closet value of function y is : " << y[i] << " for x = " << x[i] << endl;
// dont forget to include numeric
cout << "Average value of function y for the given range is : " << accumulate(y.begin(), y.end(), 0, std::plus<float>()) / float(y.size()) << endl;
cout << "y change its sign" << endl;
int num_changes = 0;
for (int k = 1; k < y.size(); k++)
{
if ((y[k - 1] < 0 && y[k]>0) || (y[k - 1] > 0 && y[k] < 0))
{
++num_changes;
cout << "change " << num_changes << endl;
cout << "--------------" << endl;
cout << "x =" << x[k - 1] << ", y =" << y[k - 1] << endl;
cout << "x =" << x[k] << ", y =" << y[k] << endl;
}
}
cout << "total number of time y changes its sign is " << num_changes;
}
float my_function(float x)
{
return 0.05 * pow(x, 3) + sin(3 * x) + 4;
}

Step by step
Solved in 2 steps with 2 images

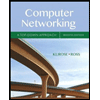
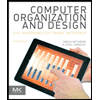
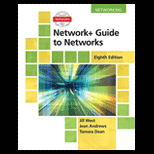
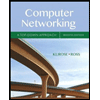
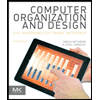
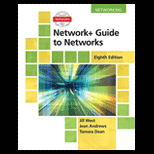
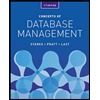
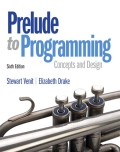
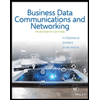