in c++ In the preceding exercises, you saw how one can use stepwise refinement to solve the problem of translating Wiki markup to HTML. Now turn the pseudocode into code. Complete the convert_to_HTML and next_symbol functions..............
in c++ In the preceding exercises, you saw how one can use stepwise refinement to solve the problem of translating Wiki markup to HTML. Now turn the pseudocode into code. Complete the convert_to_HTML and next_symbol functions..............
#include <iostream>
#include <string>
using namespace std;
// Functions defined below
string convert_to_HTML(string message);
int next_symbol(string message, string symbols, int start);
string replace_at(string str, int position, string replacement);
string tag_for_symbol(string symbol);
string replace_escapes(string str);
/**
Converts a message with Wiki markup to HTML.
@param message the message with markup
@return the message with Wiki notation converted to HTML
*/
string convert_to_HTML(string message)
{
string result = message;
/* Your code goes here */
return replace_escapes(result);
}
/**
Finds the next unescaped symbol.
@param message a message with Wiki markup
@param symbols the symbols to search for
@param start the starting position for the search
@return the position of the next markup symbol at or after start
*/
int next_symbol(string message, string symbols, int start)
{
/* Your code goes here */
}
int main()
{
string message;
getline(cin, message);
cout << convert_to_HTML(message) << endl;
return 0;
}
/**
Replaces a character of a string at a given position.
@param str the string where the replacement takes place
@param position the position of the character to be replaced
@param replacement the replacement string, or the original string
if position was not valid.
*/
string replace_at(string str, int position, string replacement)
{
if (0 <= position && position < str.length())
{
return str.substr(0, position) + replacement +
str.substr(position + 1);
}
else
{
return str;
}
}
/**
Gets the HTML tag for a markup symbol.
@param symbol the markup symbol
@return the corresponding HTML tag, or "" if none found
*/
string tag_for_symbol(string symbol)
{
if (symbol == "!") { return "em"; }
else if (symbol == "*") { return "strong"; }
else if (symbol == "`") { return "code"; }
else if (symbol == "^") { return "super"; }
else if (symbol == "_") { return "sub"; }
else { return ""; }
}
/**
Removes escape sequences from a string.
@param str the string to process.
@return the string with all \\ characters removed.
*/
string replace_escapes(string str)
{
string result;
for (int i = 0; i < str.length(); i++)
{
string ch = str.substr(i, 1);
if (ch != "\\") { result = result + ch; }
}
return result;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

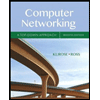
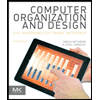
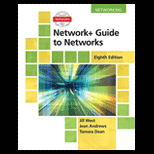
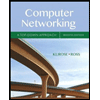
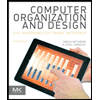
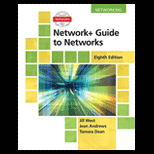
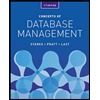
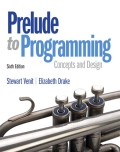
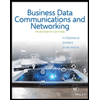