Write a C++ program in Microsoft Visual Studio 2019 and using functions only with the following features. The program reads paragraph(s) from the file and stores it in a string. Then the program counts the occurrence of each word in the paragraph(s) and stores all words with their number of occurrences. If that word has appeared more than one time in the whole string, it should store the word only once along with its total number of occurrences. The output described above (in part 3) must be stored in a new file. The program must be written in user-defined functions. Use only these libraries (iostream, string.h, ctype, fstream, algorithm,conio.h, math.h) Sample input: is the is and the is and the and is and only that is Sample output: is 5 the 3 and 4 only 1 that 1 ______________________________________________________________________________________________________________OR_____________________________________________ You can convert this code into user-defined functions (Code must be written in functions) #include #include #include #include #include using namespace std; int main() { fstream f; int i=0,b[50],j,x=0; string word,a[50]; f.open("input.txt"); while(f)//reading data from the input file { f>>word; transform(word.begin(),word.end(),word.begin(),::tolower);//to convert the word into lower case if(word[word.size()-1]=='.' || word[word.size()-1]==',' || word[word.size()-1]=='?' || word[word.size()-1]=='!') word[word.size()-1]='\0';//checking for punctuations and removing int c=0; for(j=0;j<50;j++) { transform(a[j].begin(),a[j].end(),a[j].begin(),::tolower);//conersion into lower case if(word==a[j])//checking if the word already exists { c=1; b[j]+=1; x=j; break; } } if(c==0)//if the word is not present in the array we store the value { a[i]=word;//to store the word b[i++]=1;//to store its frequency } } b[x]-=1;//reducing the extra frequency count for the last occuring word ofstream f1; f1.open("output.txt"); for(int j=0;j
Write a C++
- The program reads paragraph(s) from the file and stores it in a string.
- Then the program counts the occurrence of each word in the paragraph(s) and stores all words with their number of occurrences.
- If that word has appeared more than one time in the whole string, it should store the word only once along with its total number of occurrences.
- The output described above (in part 3) must be stored in a new file.
- The program must be written in user-defined functions.
- Use only these libraries (iostream, string.h, ctype, fstream,
algorithm ,conio.h, math.h)
Sample input:
is the is and the is and the and is and only that is
Sample output:
is 5
the 3
and 4
only 1
that 1
______________________________________________________________________________________________________________OR_____________________________________________
You can convert this code into user-defined functions (Code must be written in functions)
#include <iostream>
#include<fstream>
#include<string.h>
#include<ctype.h>
#include<algorithm>
using namespace std;
int main()
{
fstream f;
int i=0,b[50],j,x=0;
string word,a[50];
f.open("input.txt");
while(f)//reading data from the input file
{
f>>word;
transform(word.begin(),word.end(),word.begin(),::tolower);//to convert the word into lower case
if(word[word.size()-1]=='.' || word[word.size()-1]==',' || word[word.size()-1]=='?' || word[word.size()-1]=='!')
word[word.size()-1]='\0';//checking for punctuations and removing
int c=0;
for(j=0;j<50;j++)
{
transform(a[j].begin(),a[j].end(),a[j].begin(),::tolower);//conersion into lower case
if(word==a[j])//checking if the word already exists
{
c=1;
b[j]+=1;
x=j;
break;
}
}
if(c==0)//if the word is not present in the array we store the value
{
a[i]=word;//to store the word
b[i++]=1;//to store its frequency
}
}
b[x]-=1;//reducing the extra frequency count for the last occuring word
ofstream f1;
f1.open("output.txt");
for(int j=0;j<i-1;j++)//writing the output into the output.txt file
{
f1<<a[j]<<" "<<b[j]<<"\n";
}
f.close();
f1.close();
return 0;
}

Step by step
Solved in 3 steps with 2 images

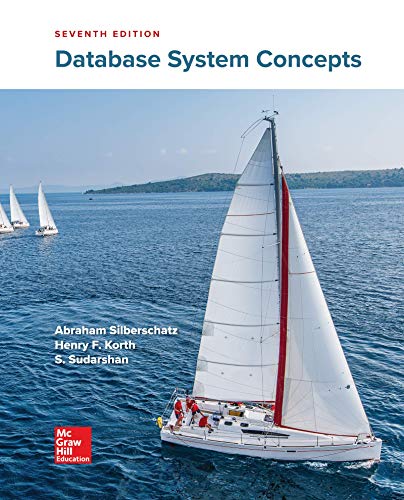
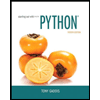
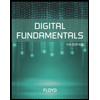
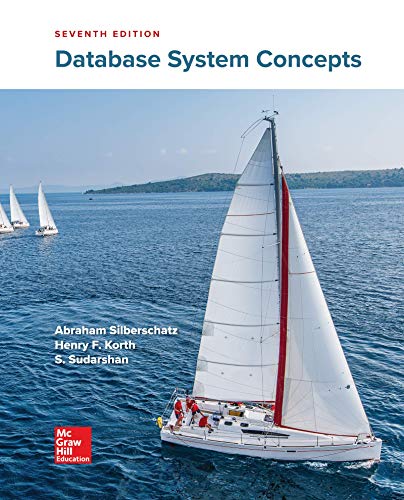
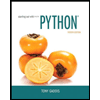
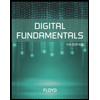
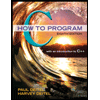
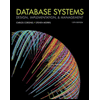
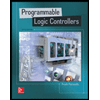