Create a class named Package with data fields for weight in ounces, shipping method, and shipping cost. The shipping method is a character: A for air, T for truck, or M for mail. The Package class contains a constructor that requires arguments for weight and shipping method. The constructor calls a calculateCost() method that determines the shipping cost, based on the following table: Weight (oz.) Air ($) Truck ($) Mail ($) 1 to 8 9 to 16 17 and over 2.00 3.00 4.50 1.50 2.35 3.25 .50 1.50 2.15 The package class also contains a display() method that displays the values in all four fields. Create a subclass named InsuredPackage that adds an insurance cost to the shipping cost, based on the following table: Shipping Cost Before Insurance ($) Additional Cost ($) 0 to 1.00 1.01 to 3.00 3.01 and over 2.45 3.95 5.55 Write an application named UsePackage that instantiates at least three objects of each type (Package and InsuredPackage) using a variety of weights and shipping method codes. Display the results for each Package and InsuredPackage. Save the files as Package.java, InsuredPackage.java, and UsePackage.java
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a class named Package with data fields for weight in ounces, shipping method, and shipping cost. The shipping method is a character: A for air, T for truck, or M for mail. The Package class contains a constructor that requires arguments for weight and shipping method. The constructor calls a calculateCost() method that determines the shipping cost, based on the following table:
Weight (oz.) | Air ($) | Truck ($) | Mail ($) |
1 to 8 9 to 16 17 and over |
2.00 3.00 4.50 |
1.50 2.35 3.25 |
.50 1.50 2.15 |
The package class also contains a display() method that displays the values in all four fields. Create a subclass named InsuredPackage that adds an insurance cost to the shipping cost, based on the following table:
Shipping Cost Before Insurance ($) | Additional Cost ($) |
0 to 1.00 1.01 to 3.00 3.01 and over |
2.45 3.95 5.55 |
Write an application named UsePackage that instantiates at least three objects of each type (Package and InsuredPackage) using a variety of weights and shipping method codes. Display the results for each Package and InsuredPackage. Save the files as Package.java, InsuredPackage.java, and UsePackage.java.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

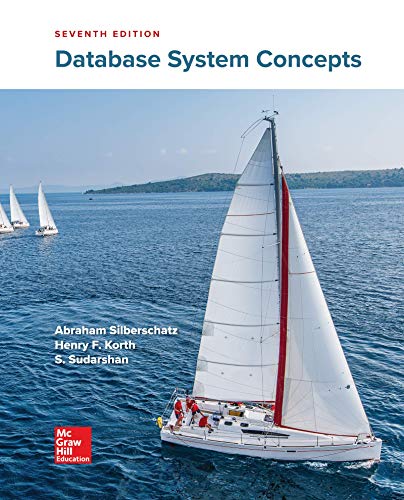
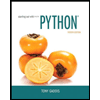
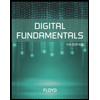
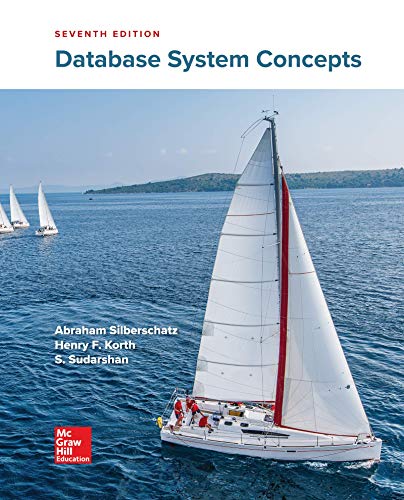
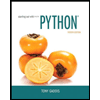
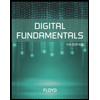
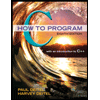
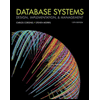
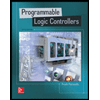