in C# i need to Create a program named PaintingDemo that instantiates an array of eight Room objects and demonstrates the Room methods. The Room constructor requires parameters for length, width, and height fields (all of type int); use a variety of values when constructing the objects. The Room class also contains the following fields: Area - The wall area of the Room (as an int) Gallons - The number of gallons of paint needed to paint the room (as an int) Both of these values are computed by calling private methods. Include read-only properties to get a Room’s values. A room is assumed to have four walls, and you do not need to allow for windows and doors, and you do not need to allow for painting the ceiling. A room requires one gallon of paint for every 350 square feet (plus an extra gallon for any square feet greater than 350). In other words, a 12 x 3 x 10 room with 9-foot ceilings has 396 square feet of wall space, and so requires two gallons of paint. the errors are Room class defined and returns its area 0 out of 1 checks passed. Compilation failed: 6 error(s), 0 warnings NtTestd1b16202.cs(12,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference? NtTestd1b16202.cs(13,26): error CS0841: A local variable `room1' cannot be used before it is declared NtTestd1b16202.cs(15,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference? NtTestd1b16202.cs(16,26): error CS0841: A local variable `room2' cannot be used before it is declared NtTestd1b16202.cs(18,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference? NtTestd1b16202.cs(19,26): error CS0841: A local variable `room3' cannot be used before it is declared Test Contents [TestFixture] public class RoomClassTest { [Test] public void RoomTest() { Room room1 = new Room(8,8,8); Assert.AreEqual(256, room1.Area); Room room2 = new Room(18,13,10); Assert.AreEqual(620, room2.Area); Room room3 = new Room(22,15,11); Assert.AreEqual(814, room3.Area); } } The Room class calculates and returns the number of gallons required to paint the room 0 out of 1 checks passed. Unit TestIncomplete The Room class can be initialized and returns the required number of gallons to paint the room Build Status Build Failed Build Output Compilation failed: 6 error(s), 0 warnings NtTest667e54f5.cs(12,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference? NtTest667e54f5.cs(13,24): error CS0841: A local variable `room1' cannot be used before it is declared NtTest667e54f5.cs(15,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference? NtTest667e54f5.cs(16,24): error CS0841: A local variable `room2' cannot be used before it is declared NtTest667e54f5.cs(18,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference? NtTest667e54f5.cs(19,24): error CS0841: A local variable `room3' cannot be used before it is declared Test Contents [TestFixture] public class RoomClassTest { [Test] public void RoomTest() { Room room1 = new Room(8,8,8); Assert.AreEqual(1, room1.Gallons); Room room2 = new Room(18,13,10); Assert.AreEqual(2, room2.Gallons); Room room3 = new Room(22,15,11); Assert.AreEqual(3, room3.Gallons); } } my code so far is using static System.Console; class PaintintDemo { static void Main() { // Write your main here } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in C# i need to
Create a program named PaintingDemo that instantiates an array of eight Room objects and demonstrates the Room methods.
The Room constructor requires parameters for length, width, and height fields (all of type int); use a variety of values when constructing the objects.
The Room class also contains the following fields:
- Area - The wall area of the Room (as an int)
- Gallons - The number of gallons of paint needed to paint the room (as an int)
Both of these values are computed by calling private methods. Include read-only properties to get a Room’s values.
A room is assumed to have four walls, and you do not need to allow for windows and doors, and you do not need to allow for painting the ceiling. A room requires one gallon of paint for every 350 square feet (plus an extra gallon for any square feet greater than 350). In other words, a 12 x 3 x 10 room with 9-foot ceilings has 396 square feet of wall space, and so requires two gallons of paint.
the errors are
Room class defined and returns its area
0 out of 1 checks passed.
Compilation failed: 6 error(s), 0 warnings
NtTestd1b16202.cs(12,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference?
NtTestd1b16202.cs(13,26): error CS0841: A local variable `room1' cannot be used before it is declared
NtTestd1b16202.cs(15,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference?
NtTestd1b16202.cs(16,26): error CS0841: A local variable `room2' cannot be used before it is declared
NtTestd1b16202.cs(18,5): error CS0246: The type or namespace name `Room' could not be found. Are you missing an assembly reference?
NtTestd1b16202.cs(19,26): error CS0841: A local variable `room3' cannot be used before it is declared
Test Contents
[TestFixture]
public class RoomClassTest
{
[Test]
public void RoomTest()
{
Room room1 = new Room(8,8,8);
Assert.AreEqual(256, room1.Area);
Room room2 = new Room(18,13,10);
Assert.AreEqual(620, room2.Area);
Room room3 = new Room(22,15,11);
Assert.AreEqual(814, room3.Area);
}
}
The Room class calculates and returns the number of gallons required to paint the room
0 out of 1 checks passed.
The Room class can be initialized and returns the required number of gallons to paint the room

Algorithm steps to solve the given problem:
- Start
- Define a class 'Room' with the member variables length, width, height, area, and gallons.
- Define a constructor for the class 'Room' which takes length, width, and height as parameters.
- In the constructor, set the member variables equal to the length, width, and height parameters and call the methods Calculate Area and Calculate Gallons.
- Define the method Calculate Area which sets the area member variable equal to 2 multiplied by the height multiplied by the sum of the length and width variables.
- Define the method Calculate Gallons which sets the gallons member variable equal to the area divided by 350, and if the remainder is not 0, then add 1 to the gallons member variable.
- Define the properties Area and Gallons for the class Room which return the area and gallons member variables, respectively.
- In the main method, declare an array of Room objects of size 3.
- Create 3 Room objects and pass in the appropriate length, width, and height values to each.
- Use a for loop to loop through each Room object in the array and output its area and gallons of paint needed.
- Output the result.
- Stop.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

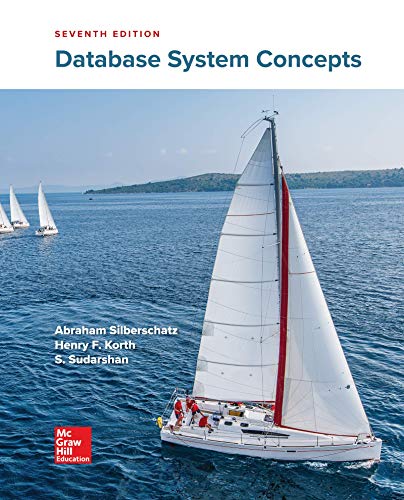
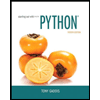
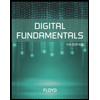
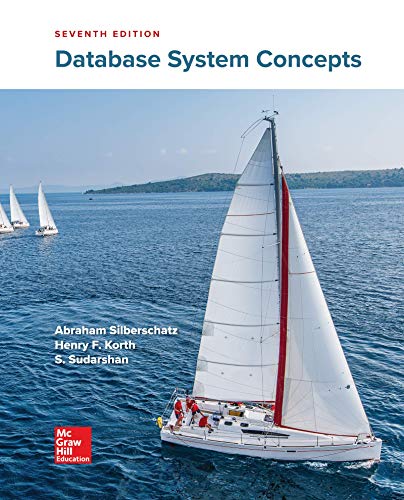
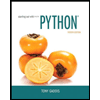
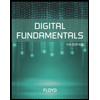
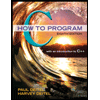
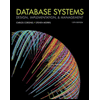
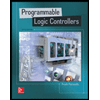