in c# i need to Create a BankAccount class with the following properties: Account Number (string) Account Holder Name (string) Balance (double) Account Type (enum: Savings, Current) Create the following methods in the BankAccount class: Deposit(double amount): Method to deposit money into the account Withdraw(double amount): Method to withdraw money from the account CheckBalance(): Method to check the current balance of the account I am getting a error in pic my code is // Define an enumeration type for bank account types public enum AccountType { Savings, Current } // Define a class for bank accounts public class BankAccount { // Properties for the account number, account holder name, balance and account type public string AccountNumber { get; set; } public string AccountHolderName { get; set; } public double Balance { get; set; } public AccountType AccountType { get; set; } // Method to deposit money into the account public void Deposit(double amount) { Balance += amount; } // Method to withdraw money from the account // Returns true if the withdrawal was successful, false otherwise public bool Withdraw(double amount) { if (Balance >= amount) { Balance -= amount; return true; } return false; } // Method to check the balance of the account // Returns the balance amount public double CheckBalance() { return Balance; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in c# i need to
Create a BankAccount class with the following properties:
Account Number (string)
Account Holder Name (string)
Balance (double)
Account Type (enum: Savings, Current)
Create the following methods in the BankAccount class:
Deposit(double amount): Method to deposit money into the account
Withdraw(double amount): Method to withdraw money from the account
CheckBalance(): Method to check the current balance of the account
I am getting a error in pic
my code is
// Define an enumeration type for bank account types
public enum AccountType
{
Savings,
Current
}
// Define a class for bank accounts
public class BankAccount
{
// Properties for the account number, account holder name, balance and account type
public string AccountNumber { get; set; }
public string AccountHolderName { get; set; }
public double Balance { get; set; }
public AccountType AccountType { get; set; }
// Method to deposit money into the account
public void Deposit(double amount)
{
Balance += amount;
}
// Method to withdraw money from the account
// Returns true if the withdrawal was successful, false otherwise
public bool Withdraw(double amount)
{
if (Balance >= amount)
{
Balance -= amount;
return true;
}
return false;
}
// Method to check the balance of the account
// Returns the balance amount
public double CheckBalance()
{
return Balance;
}
}
![Error: Specified file could not be compiled.
e:\stef C# homework\New folder\BankAccount.cs(0,0): error CS5001: Program does not contain a static 'Main' method suitable for an entry point [C:\Users\stefa\AppData\Local\Temp\
csscript.core\cache\-306824470\.build\BankAccount.cs\BankAccount.csproj]
e:\stef C# homework\New folder\BankAccount.cs(0,0): error CS5001: Program does not contain a static 'Main' method suitable for an entry point [C:\Users\stefa\AppData\Local\Temp\
csscript.core\cache\-306824470\.build\BankAccount.cs\BankAccount.csproj]
PS E:\stef C# homework\New folder>](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda2a136f-d248-470a-adf7-afa3bf81602c%2Fda556cc2-da63-47a1-a467-7713aac14013%2Fyyhzuwn_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

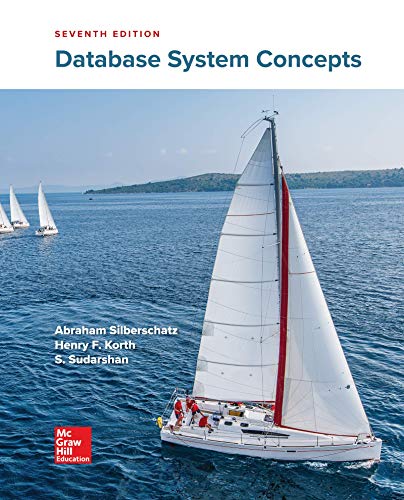
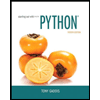
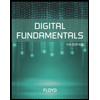
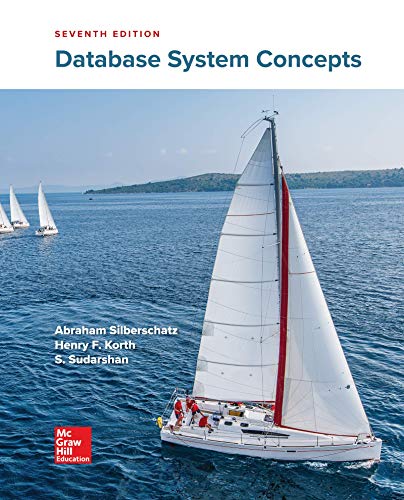
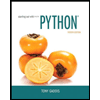
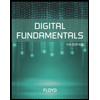
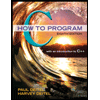
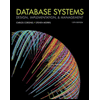
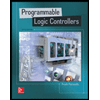