Code is complete, I just need an explanation on how this code functions. #include using namespace std; class node { public: double data; node* next; }; node* head; void addData(double val) { node *n = new node; n->data=val; n->next=NULL; if(head==NULL) { head=n; } else { node *ptr=head; while(ptr->next!=NULL) { ptr=ptr->next; } ptr->next=n; } } void addBack(double x) { node *n = new node; n->data=x; n->next=NULL; node *ptr=head; while(ptr->next!=NULL) { ptr=ptr->next; } ptr->next=n; } void display() { node *ptr=head; while(ptr!=NULL) { cout<data<<", "; ptr=ptr->next; } cout<
Code is complete, I just need an explanation on how this code functions.
#include <iostream>
using namespace std;
class node
{
public:
double data;
node* next;
};
node* head;
void addData(double val)
{
node *n = new node;
n->data=val;
n->next=NULL;
if(head==NULL)
{
head=n;
}
else
{
node *ptr=head;
while(ptr->next!=NULL)
{
ptr=ptr->next;
}
ptr->next=n;
}
}
void addBack(double x)
{
node *n = new node;
n->data=x;
n->next=NULL;
node *ptr=head;
while(ptr->next!=NULL)
{
ptr=ptr->next;
}
ptr->next=n;
}
void display()
{
node *ptr=head;
while(ptr!=NULL)
{
cout<<ptr->data<<", ";
ptr=ptr->next;
}
cout<<endl;
}
int main()
{
head=NULL;
addData(4.7);
addData(12.8);
addData(14.7);
addData(16.2);
cout<<"Linked List"<<endl;
display();
addBack(56.9);
cout<<"After adding node with data 56.9 to the back of linked list:";
display();
addBack(11.9);
cout<<"After adding node with data 11.9 to the back of linked list:";
display();
addBack(34.9);
cout<<"After adding node with data 34.9 to the back of linked list:";
display();
return 0;
}

Step by step
Solved in 2 steps

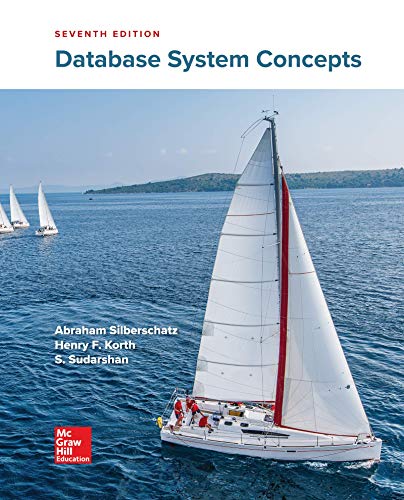
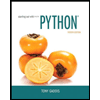
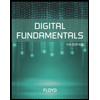
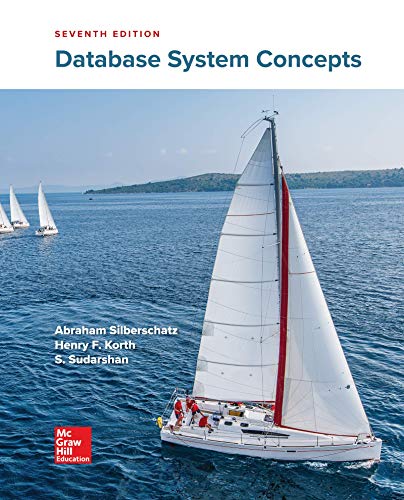
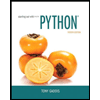
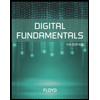
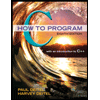
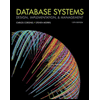
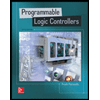