#include #include #include #include using namespace std; const int TABLE_SIZE = 128; /* * HashEntry Class Declaration */ class HashEntry { public: int key; int value; HashEntry(int key, int value) { this->key = key; this->value = value; } }; /* * HashMap Class Declaration */ class HashMap { private: HashEntry **table; public: HashMap() { table = new HashEntry * [TABLE_SIZE]; for (int i = 0; i< TABLE_SIZE; i++) { table[i] = NULL; } } /* * Hash Function */ int HashFunc(int key) { return key % TABLE_SIZE; } /* * Insert Element at a key */ void Insert(int key, int value) { int hash = HashFunc(key); while (table[hash] != NULL && table[hash]->key != key) { hash = HashFunc(hash + 1); } if (table[hash] != NULL) delete table[hash]; table[hash] = new HashEntry(key, value); } /* * Search Element at a key */ int Search(int key) { int hash = HashFunc(key); while (table[hash] != NULL && table[hash]->key != key) { hash = HashFunc(hash + 1); } if (table[hash] == NULL) return -1; else return table[hash]->value; } /* * Remove Element at a key */ void Remove(int key) { int hash = HashFunc(key); while (table[hash] != NULL) { if (table[hash]->key == key) break; hash = HashFunc(hash + 1); } if (table[hash] == NULL) { cout<<"No Element found at key "<>choice; switch(choice) { case 1: cout<<"Enter element to be inserted: "; cin>>value; cout<<"Enter key at which element to be inserted: "; cin>>key; hash.Insert(key, value); break; case 2: cout<<"Enter key of the element to be searched: "; cin>>key; if (hash.Search(key) == -1) { cout<<"No element found at key "<>key; hash.Remove(key); break; case 4: exit(1); default: cout<<"\nEnter correct option\n"; } } return 0; } Based on the code above. Update the code for Hash Tables so it will Accept an integer key and Map to String Value. ID (Key) Namee 1234 “Jan Smith” 3445 “John Do” 9762 “Jane Collins” 1981 “Bill Rodgers” 8653 “Eric Jones” 4321 “Jill Wright” “8763” “Karen Bader” Use Conventional Method Names Insert, Remove, Search: insert(key, value) insert(ID, “NAMe”) Search(“id”) → Method will return value Remove(“id”) → Method will delete key and value
#include<iostream>
#include<cstdlib>
#include<string>
#include<cstdio>
using namespace std;
const int TABLE_SIZE = 128;
/*
* HashEntry Class Declaration
*/
class HashEntry
{
public:
int key;
int value;
HashEntry(int key, int value)
{
this->key = key;
this->value = value;
}
};
/*
* HashMap Class Declaration
*/
class HashMap
{
private:
HashEntry **table;
public:
HashMap()
{
table = new HashEntry * [TABLE_SIZE];
for (int i = 0; i< TABLE_SIZE; i++)
{
table[i] = NULL;
}
}
/*
* Hash Function
*/
int HashFunc(int key)
{
return key % TABLE_SIZE;
}
/*
* Insert Element at a key
*/
void Insert(int key, int value)
{
int hash = HashFunc(key);
while (table[hash] != NULL && table[hash]->key != key)
{
hash = HashFunc(hash + 1);
}
if (table[hash] != NULL)
delete table[hash];
table[hash] = new HashEntry(key, value);
}
/*
* Search Element at a key
*/
int Search(int key)
{
int hash = HashFunc(key);
while (table[hash] != NULL && table[hash]->key != key)
{
hash = HashFunc(hash + 1);
}
if (table[hash] == NULL)
return -1;
else
return table[hash]->value;
}
/*
* Remove Element at a key
*/
void Remove(int key)
{
int hash = HashFunc(key);
while (table[hash] != NULL)
{
if (table[hash]->key == key)
break;
hash = HashFunc(hash + 1);
}
if (table[hash] == NULL)
{
cout<<"No Element found at key "<<key<<endl;
return;
}
else
{
delete table[hash];
}
cout<<"Element Deleted"<<endl;
}
~HashMap()
{
for (int i = 0; i < TABLE_SIZE; i++)
{
if (table[i] != NULL)
delete table[i];
delete[] table;
}
}
};
/*
* Main Contains Menu
*/
int main()
{
HashMap hash;
int key, value;
int choice;
while (1)
{
cout<<"\n----------------------"<<endl;
cout<<"Operations on Hash Table"<<endl;
cout<<"\n----------------------"<<endl;
cout<<"1.Insert element into the table"<<endl;
cout<<"2.Search element from the key"<<endl;
cout<<"3.Delete element at a key"<<endl;
cout<<"4.Exit"<<endl;
cout<<"Enter your choice: ";
cin>>choice;
switch(choice)
{
case 1:
cout<<"Enter element to be inserted: ";
cin>>value;
cout<<"Enter key at which element to be inserted: ";
cin>>key;
hash.Insert(key, value);
break;
case 2:
cout<<"Enter key of the element to be searched: ";
cin>>key;
if (hash.Search(key) == -1)
{
cout<<"No element found at key "<<key<<endl;
continue;
}
else
{
cout<<"Element at key "<<key<<" : ";
cout<<hash.Search(key)<<endl;
}
break;
case 3:
cout<<"Enter key of the element to be deleted: ";
cin>>key;
hash.Remove(key);
break;
case 4:
exit(1);
default:
cout<<"\nEnter correct option\n";
}
}
return 0;
}
Based on the code above.
Update the code for Hash Tables so it will Accept an integer key and Map to String Value.
ID (Key) |
Namee |
1234 |
“Jan Smith” |
3445 |
“John Do” |
9762 |
“Jane Collins” |
1981 |
“Bill Rodgers” |
8653 |
“Eric Jones” |
4321 |
“Jill Wright” |
“8763” |
“Karen Bader” |
Use Conventional Method Names Insert, Remove, Search:
insert(key, value)
insert(ID, “NAMe”)
Search(“id”) → Method will return value
Remove(“id”) → Method will delete key and value

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

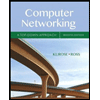
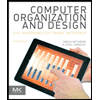
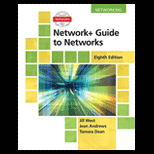
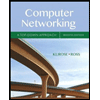
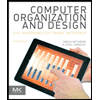
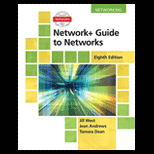
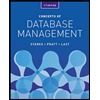
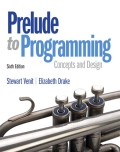
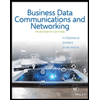