C++ Programming Redesign your class myArray using class templates so that the class can be used in any application that requires arrays to process data. #include #include "myArray.h" using namespace std; int main() { myArray list1(5); myArray list2(5); int i; cout << "list1 : "; for (i = 0; i < 5; i++) cout << list1[i] << " "; cout << endl; cout << "Enter 5 integers: "; for (i = 0; i < 5; i++) cin >> list1[i]; cout << endl; cout << "After filling list1: "; for (i = 0; i < 5; i++) cout << list1[i] << " "; cout << endl; list2 = list1; cout << "list2 : "; for (i = 0; i < 5; i++) cout << list2[i] << " "; cout << endl; cout << "Enter 3 elements: "; for (i = 0; i < 3; i++) cin >> list1[i]; cout << endl; cout << "First three elements of list1: "; for (i = 0; i < 3; i++) cout << list1[i] << " "; cout << endl; myArray list3(-2, 6); cout << "list3: "; for (i = -2; i < 6; i++) cout << list3[i] << " "; cout << endl; list3[-2] = 7; list3[4] = 8; list3[0] = 54; list3[2] = list3[4] + list3[-2]; cout << "list3: "; for (i = -2; i < 6; i++) cout << list3[i] << " "; cout << endl; system("pause"); return 0; } Source code for myArray.cpp: #include "myArray.h" #include #include "assert.h" using namespace std; //constructor for object that takes in two parameters myArray::myArray(int indexS, int indexE) { length = indexE - indexS; //set the length of the array indexStart = indexS; //set the starting array location indexEnd = indexE - 1; //set the ending array location list = new int[length]; //creating the array and setting the list to the new length of array. for (int i = indexStart; i <= indexEnd; i++) //initiallizing the elements within the array and setting each element to 0 { *(list + i) = 0; } } //constructor for the object that takes in one parameter myArray::myArray(int arrayLength) { length = arrayLength; //set the length of the array indexStart = 0; //set the starting array location indexEnd = arrayLength - 1; // set the ending array location list = new int[length]; //creating the array and setting the list to the new length of array for (int i = indexStart; i <= indexEnd; i++)//initiallizing the elements within the array and setting each element to 0 { *(list + i) = 0; } } int& myArray::operator[](int index) //overloading array index { if (index >= indexStart && index <= indexEnd) //check to see if its within bounds { int &indexLoc = *(list + index); //set memeory location to store actual value of the list index. return indexLoc; } else { cout << "Index is out of range.\n"; exit(0); } } Source code for myArray.h: #pragma once class myArray { public: myArray(int indexS, int indexE); myArray(int arrayLength); int& operator[] (const int index); private: int* list; int length; int indexStart; int indexEnd; };
C++ Programming
Redesign your class myArray using class templates so that the class can be used in any application that requires arrays to process data.
#include <iostream>
#include "myArray.h"
using namespace std;
int main()
{
myArray list1(5);
myArray list2(5);
int i;
cout << "list1 : ";
for (i = 0; i < 5; i++)
cout << list1[i] << " ";
cout << endl;
cout << "Enter 5 integers: ";
for (i = 0; i < 5; i++)
cin >> list1[i];
cout << endl;
cout << "After filling list1: ";
for (i = 0; i < 5; i++)
cout << list1[i] << " ";
cout << endl;
list2 = list1;
cout << "list2 : ";
for (i = 0; i < 5; i++)
cout << list2[i] << " ";
cout << endl;
cout << "Enter 3 elements: ";
for (i = 0; i < 3; i++)
cin >> list1[i];
cout << endl;
cout << "First three elements of list1: ";
for (i = 0; i < 3; i++)
cout << list1[i] << " ";
cout << endl;
myArray list3(-2, 6);
cout << "list3: ";
for (i = -2; i < 6; i++)
cout << list3[i] << " ";
cout << endl;
list3[-2] = 7;
list3[4] = 8;
list3[0] = 54;
list3[2] = list3[4] + list3[-2];
cout << "list3: ";
for (i = -2; i < 6; i++)
cout << list3[i] << " ";
cout << endl;
system("pause");
return 0;
}
Source code for myArray.cpp:
#include "myArray.h"
#include <iostream>
#include "assert.h"
using namespace std;
//constructor for object that takes in two parameters
myArray::myArray(int indexS, int indexE)
{
length = indexE - indexS; //set the length of the array
indexStart = indexS; //set the starting array location
indexEnd = indexE - 1; //set the ending array location
list = new int[length]; //creating the array and setting the list to the new length of array.
for (int i = indexStart; i <= indexEnd; i++) //initiallizing the elements within the array and setting each element to 0
{
*(list + i) = 0;
}
}
//constructor for the object that takes in one parameter
myArray::myArray(int arrayLength)
{
length = arrayLength; //set the length of the array
indexStart = 0; //set the starting array location
indexEnd = arrayLength - 1; // set the ending array location
list = new int[length]; //creating the array and setting the list to the new length of array
for (int i = indexStart; i <= indexEnd; i++)//initiallizing the elements within the array and setting each element to 0
{
*(list + i) = 0;
}
}
int& myArray::operator[](int index) //overloading array index
{
if (index >= indexStart && index <= indexEnd) //check to see if its within bounds
{
int &indexLoc = *(list + index); //set memeory location to store actual value of the list index.
return indexLoc;
}
else
{
cout << "Index is out of range.\n";
exit(0);
}
}
Source code for myArray.h:
#pragma once
class myArray
{
public:
myArray(int indexS, int indexE);
myArray(int arrayLength);
int& operator[] (const int index);
private:
int* list;
int length;
int indexStart;
int indexEnd;
};

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

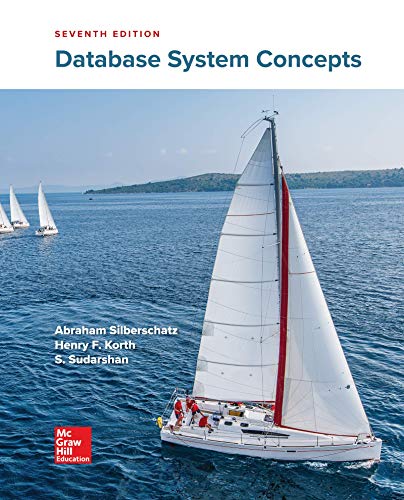
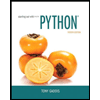
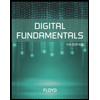
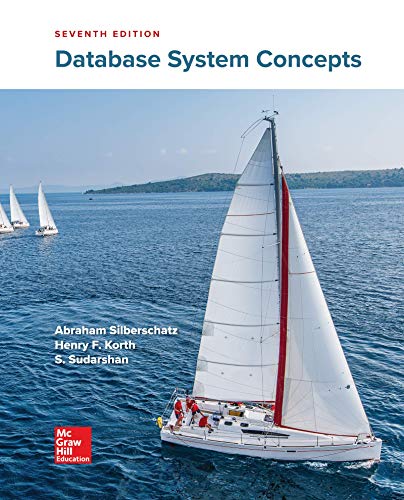
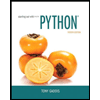
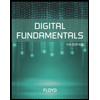
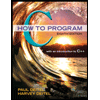
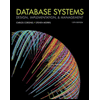
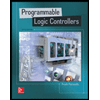