ECE526-HW#2-solutions
docx
keyboard_arrow_up
School
California State University, Northridge *
*We aren’t endorsed by this school
Course
526
Subject
Mechanical Engineering
Date
Dec 6, 2023
Type
docx
Pages
4
Uploaded by ChefSummerFlamingo33
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
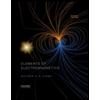
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
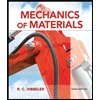
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
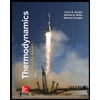
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
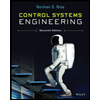
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
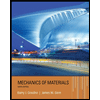
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
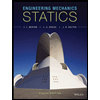
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
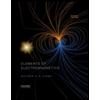
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
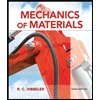
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
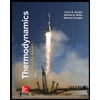
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
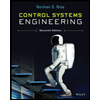
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
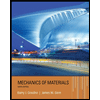
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
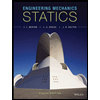
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY