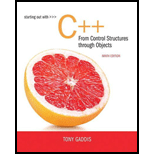
A)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
B)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
C)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
D)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
- Largest/Smallest Array Values Write a program that lets the user enter 10 values into an array. The program should then display the largest and smallest values stored in the array. Do question 7(above) again this time using 5 functions. void getValues(int [], int); void displayValues(const int[], int); int largest(const int[],int); int smallest(const int[],int); void displayLargestSmallest(int,int);arrow_forwardbe recor #include #include minutes #include limit on int func(int, int, int, int); main(){ srand(time(NULL)); int a, b, c, fNum; printf("Choose three different numbers between 0-39:"); scanf ("%d%d%d", &a, &b, &c); fNum = func (a, b, c, 25); printf("\nThe result: %d", fNum); } int func (int ul, int u2, int u3, int iter){ srand (time (NULL)); int n1=0, i=0, count=0; for (;iarrow_forwardC++ Coding Write a program that asks a teacher to enter the student's name and the grades received on her quarterly exams. The datatype for the student's name could be a string and the four grades should be entered in an array of data types int. Test scores are on the base of 100. REMINDER: sum and cap CANNOT be integers. The main() functions describe the average function with the following title line: double avg( int grades[], int cap ) The program prints the student's name, her four grades, and her average grades The main() function also uses the following print function: void print( int grades[], int cap);arrow_forwardThe following code will display 150: { int a[] = { 10,20,30,40,50 }; int sum = 0; for (int i = 0; i <= 4;i++ ) sum += a[--i]; cout << sum; Select one: True Falsearrow_forwardYou are given the following two dimensional arrays of people and their birth months: String [][] people = {{June, May, March, June, May, March, February, June, May, January, May, March}, {John, Mary, Peter, Bob, Sam, Dan, Judith, Gary, Tiff, Suzy, Jim, Jane}} Create an enum type for the the first six months of the year Randomly select a month from this enumarrow_forwardConsider a two-by-three integer array t. a) Write a statement that declares and creates t.b) How many rows does t have? c) How many columns does t have? d) How many elements does t have? e) Write access expressions for all the elements in row 1 of t. f) Write access expressions for all the elements in column 2 of t. g) Write a single statement that sets the element of t in row 0 and column 1 to zero. h) Write a series of statements that initializes each element of t to zero. Do not use a repetition statement. i) Write a nested for statement that initializes each element of t to zero.j) Write a nested for statement that inputs the values for the elements of t from the user. k) Write a series of statements that determines and displays the smallest value in t. l) Write a printf statement that displays the elements of the first row of t. Do not use repetition. m) Write a statement that totals the elements of the third column of t. Do not use repetition. n) Write a series of…arrow_forwardC#/Csharp program Tyarrow_forwardC++ Coding Write a program that asks a teacher to enter the student's and the grades received on her quarterly exams. The datatype for the student's name could be a string and the four grades should be entered in an array of data types int. Test scores are on the base of 100. The main() functions describe the average function with the following title line: double avg( int grades[] , int cap ): The program prints the student's name, her four grades, and her average grades. The main() function also uses the following print function: void print( int grades[], int cap);arrow_forward1) Arrays have a built in toString method that returns all of the elements in the array as one String with “\n” insertedbetween each element.arrow_forwardWhen traversing an array, which of the following statements does not need to keep track of the individual array subscripts: Do...Loop, For...Next, or For Each...Next?arrow_forwardDisplay Array values Write a program that lets the user enter ten values into an array. Then pass the array, the size of the array, and the number, num to a function called displayArrayValues(). The function should then display all the numbers in the array larger than n. Write a program using C++.arrow_forward+ || * 00 < CO Final Discount Price A shopkeeper has a sale to complete and has arranged the items being sold in an array. Starting from the left, the shopkeeper sells each item at its full price minus the price of the first lower or equal priced item to its right. If there is no item to the right that costs less than or equal to the current item's price, the current item is sold at full price. For example, assume there are items priced [2, 3, 1, 2, 4, 2] • The items 0 and 1 are each discounted by 1 unit, the first equal or lower price to the right • Item 2, priced 1 unit sells at full price because there are no equal or lower priced items to the right • The next item, item 3 at 2 units, is discounted 2 units, as would the item 4 at 4 units • The final item 5 at 2 units must be purchased at full price because there are no lower prices to the right The total cost is 1+2+1+0+2+2=8units. The full price items are at indices [2, 5] using Obased indexing. You have to print the sum of the…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
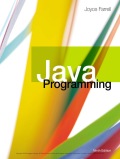