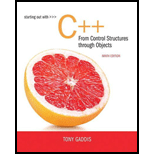
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 9, Problem 26RQE
Explanation of Solution
Program Code:
The below code is used to dynamically allocate array of 20 integers and allows user to get values for the array from the user.
// Include the necessary headers
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//defining the size of the array
const int SIZE = 20;
//pointer variable declaration
int *myptr;
//allocation of memory
myptr = new int[SIZE];
//for loop that iterates for the given size of array
for (int j = 0; j < SIZE; j++)
{
//get...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Declare an integer and an array of doubles called pointValues
The array can hold 110 doubles
Ask the user how many points they want to enter (up to 110)
Get the number from the user
Fill the array with with user input (HINT: use a loop and prompt for input)
For example if the user entered 100 you would write a loop to have the user enter the 100
pointValues one by one
In visual basic
Write a For…Next loop that will printout each value in the array from problem 4.
Ch7 - Arrays java
In a loop, ask the user to enter 10 integer values and store the values in an array. Pass the array to a method. In the method, use a loop to subtract 5 from each element and return the changed array to the main method.In the main method, use a loop to add the array values and display the result.
Chapter 9 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 9.5 - Prob. 9.1CPCh. 9.5 - Write the definition statement for a variable...Ch. 9.5 - List three uses of the symbol in C++.Ch. 9.5 - What is the output of the following code? int x =...Ch. 9.5 - Rewrite the following loop so it uses pointer...Ch. 9.5 - Prob. 9.6CPCh. 9.5 - Prob. 9.7CPCh. 9.5 - Is each of the following definitions valid or...Ch. 9.9 - Prob. 9.9CPCh. 9.9 - Give an example of the proper way to call the...
Ch. 9.9 - Complete the following program skeleton. When...Ch. 9.9 - Look at the following array definition: const int...Ch. 9.9 - Assume ip is a pointer to an int. Write a...Ch. 9.9 - Prob. 9.14CPCh. 9.9 - Prob. 9.15CPCh. 9.9 - Prob. 9.16CPCh. 9.9 - Prob. 9.17CPCh. 9 - What does the indirection operator do?Ch. 9 - Look at the following code. int x = 7; int iptr =...Ch. 9 - So far you have learned three different uses for...Ch. 9 - Prob. 4RQECh. 9 - Prob. 5RQECh. 9 - Prob. 6RQECh. 9 - What is the purpose of the new operator?Ch. 9 - What happens when a program uses the new operator...Ch. 9 - Prob. 9RQECh. 9 - Prob. 10RQECh. 9 - Prob. 11RQECh. 9 - Prob. 12RQECh. 9 - Each byte in memory is assigned a unique...Ch. 9 - The _________ operator can be used to determine a...Ch. 9 - Prob. 15RQECh. 9 - The ________ operator can be used to work with the...Ch. 9 - Array names can be used as ________, and vice...Ch. 9 - Prob. 18RQECh. 9 - The ________ operator is used to dynamically...Ch. 9 - Under older compilers, if the new operator cannot...Ch. 9 - Prob. 21RQECh. 9 - When a program is finished with a chunk of...Ch. 9 - You should only use pointers with delete that were...Ch. 9 - Prob. 24RQECh. 9 - Look at the following array definition: int...Ch. 9 - Prob. 26RQECh. 9 - Assume tempNumbers is a pointer that points to a...Ch. 9 - Look at the following function definition: void...Ch. 9 - Prob. 29RQECh. 9 - Prob. 30RQECh. 9 - Prob. 31RQECh. 9 - T F The operator is used to get the address of a...Ch. 9 - T F Pointer variables are designed to hold...Ch. 9 - T F The symbol is called the indirection...Ch. 9 - T F The operator dereferences a pointer.Ch. 9 - T F When the indirection operator is used with a...Ch. 9 - T F Array names cannot be dereferenced with the...Ch. 9 - Prob. 38RQECh. 9 - T F The address operator is not needed to assign...Ch. 9 - T F You can change the address that an array name...Ch. 9 - T F Any mathematical operation, including...Ch. 9 - T F Pointers may be compared using the relational...Ch. 9 - T F When used as function parameters, reference...Ch. 9 - T F The new operator dynamically allocates memory.Ch. 9 - T F A pointer variable that has not been...Ch. 9 - Prob. 46RQECh. 9 - T F In using a pointer with the delete operator,...Ch. 9 - Prob. 48RQECh. 9 - Prob. 49RQECh. 9 - int x, ptr = nullptr; ptr = x;Ch. 9 - Prob. 51RQECh. 9 - Prob. 52RQECh. 9 - Prob. 53RQECh. 9 - float level; int fptr = level;Ch. 9 - Prob. 55RQECh. 9 - Prob. 56RQECh. 9 - Prob. 57RQECh. 9 - Prob. 58RQECh. 9 - int pint = nullptr; pint = new int[100]; //...Ch. 9 - Prob. 60RQECh. 9 - Prob. 61RQECh. 9 - Prob. 62RQECh. 9 - Array Allocator Write a function that dynamically...Ch. 9 - Test Scores #1 Write a program that dynamically...Ch. 9 - Drop Lowest Score Modify Problem 2 above so the...Ch. 9 - Test Scores #2 Modify the program of Programming...Ch. 9 - Prob. 5PCCh. 9 - Case Study Modification #1 Modify Program 9-19...Ch. 9 - Case Study Modification #2 Modify Program 9-19...Ch. 9 - Mode Function In statistics, the mode of a set of...Ch. 9 - Median Function In statistics, when a set of...Ch. 9 - Reverse Array Write a function that accepts an int...Ch. 9 - Array Expander Write a function that accepts an...Ch. 9 - Element Shifter Write a function that accepts an...Ch. 9 - Movie Statistics Write a program that can be used...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question 1 Write a program that declares an array of 100 doubles. The program asks the user to enter a number N between 100, and then: initializes the first N elements of the array to random numbers between 1 and 100. calculates the average of these numbers and displays it. A final loop displays the elements of the array, each on a line, with an indication as to whether that number is lower or higher than the average. For example, if the numbers generated are 5, 28, 3, 8, 15, 7, 22, 6, 1, 4, then the program will print: Average = 9.9 5 28 3 8 15 7 22 6 1 4 11 11 11 -- 11 11 1- —— 11 lower than average higher than average lower than average lower than average higher than average lower than average higher than average lower than average lower than average lower than averagearrow_forwardUsing c++ write a program Program : Date Printer Write a program that reads a char array from the user containing a date in the form mm/dd/yyyy. It should print the date in the form March 12, 2014. For example Enter Date 5/14/2019 May 14, 2019 Validation Required: Month should be less than 13, day should be less than 31.arrow_forwardDate Printer Write a program that reads a char array from the user containinga date in the form mm/dd/yyyy. It should print the date in the form March 12, 2014.arrow_forward
- An array can hold as much different data types as you want. True Falsearrow_forwardpseudo code in problem solving and logic and flowchartTotal Sales (Arrays) Design a program that asks the user to enter a store’s sales for each day of the week (for one week). The amounts should be stored in an array. Use a loop to calculate the total sales for the week and display the result. Guideline: Rename the variables with your student number (e.g., amount_697) Use an array to solve the above problem Add comments with your name/student number Comment your codearrow_forwardA for loop is often used when processing the elements of an array. Given an array called scores with 10 elements, what entries would complete the for loop header shown below? for (int i = 10; i++) O first blank: 0 second blank: <= O first blank: 0 second blank: < O first blank: 1 second blank: <= first blank: 1 second blank: < O Oarrow_forward
- Problem2 Write a program that display the position of a given element in an array. You should print the index (i.e. the position) of the element. If the element appears more than one time than you should print all its positions. The size of the array should be entered by the user. If the element does not occur then you should display element not found. Sample1: Enter the size of the array: 5 Enter an array of size 5: 44 5 13 44 67 Enter the element to find: 44 44 is found at position 44 is found at position 44 occurs 2 time(s) Sample2: Enter the size of the array: 4 Enter an array of size 4: 12 150 17 20 Enter the element: 18 18 is not foundarrow_forwardThe array size declarator must be an integer expression with a value greater than or equal to zero. True Falsearrow_forwardAskForNumbers Declare an integer array locally with the size of 200. Create a program that asks the user how many numbers the have. Use your getChoice() function from before. Make sure it does not exceed 200 as the locally declared array has the size of 200 Use a for loop and ask the user to enter each value that must be stored in the array Use a second loop to display each number, and also determine the average of all values in the array After the for loop, display the average of all numbers. This program will let you enter a list of numbers into an array. It will then display all of the numbers, and finally display the average of all numbers. How many numbers would you like to enter?5 Please enter a number: 22 Please enter a number: 33 Please enter a number:44 Please enter a number: 55 Please enter a number: 66 lumber 1 is 22 lumber 2 is 33 lumber 3 is 44 lumber 4 is 55 lumber 5 is 66 The average is 44arrow_forward
- In C#, how would you compile a program that declares a 5 element double array that uses a loop to read values for each element from the user. The program should then use an additional loop to compute and output the sum of the elements in the array.arrow_forwardCreate a Console application that does the following:This program must use a single array to store integers and find the maximum value.1. Create an array and initialize it internally with the values 5, 8, 9, 4, and 2.2. Use a loop to iterate through the array and determine the maximum value.arrow_forwardLottery Number Generator Problem Use pseudocode to design a program that generates a 7-digit lottery number. This program should have an integer array with 7 elements. Then Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Finally, write another loop that displays the contents of the array.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
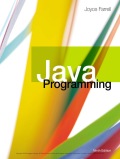
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
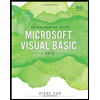
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning