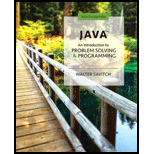
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 8.2, Problem 9STQ
Add a constructor to the class Student that sets the student’s name to a given argument string and sets the student’s number to zero. Your constructor should invoke another constructor in Student to accomplish this.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Problem Class
In this exercise, you are going to create the Problem class. The Problem class is used
to help simulate a math fact, for example:
2+5=
Your class needs to contain two constructors, one that takes String, int, int that
represents the operator sign(+,-, *, or /), the minimum, and maximum values for the
number range, and a second constructor that takes only a String that represents the
operator sign. For the second constructor, the minimum should default to zero and the
maximum to ten.
Your Problem object should generate 2 random integers between the minimum and
maximum values (inclusively). Each Problem object should only have one set of
numbers that do not change.
While you may include additional helper methods, two methods need to be available
to the user. The first is the answer method that should return a double that represents
the answer to the problem.
The second is the toString that should return a String that represents the problem. The
format should be:
number…
It is customary that we write this method to return a string representation of objects in a class.
constructor
mutator
toString
accessor
-int x //x coord of the center
-int y // y coord of the center
-int radius
-static int count // static variable to keep count of number of circles created
+ Circle() //default constructor that sets origin to (0,0) and radius to 1
+Circle(int x, int y, int radius) // regular constructor
+getX(): int
+getY(): int
+getRadius(): int
+setX(int newX: void
+setY(int newY): void
+setRadius(int newRadius):void
+getArea(): double // returns the area using formula pi*r^2
+getCircumference // returns the circumference using the formula 2*pi*r
+toString(): String // return the circle as a string in the form (x,y) : radius
+getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle
+moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates
+intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false
+resize(double scale):void// * multiply the…
Chapter 8 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 8.1 - Prob. 1STQCh. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Can a derived class directly access by name a...Ch. 8.1 - Can a derived class directly invoke a private...Ch. 8.1 - Prob. 6STQCh. 8.1 - Suppose s is an object of the class Student. Base...Ch. 8.2 - Give a complete definition of a class called...Ch. 8.2 - Add a constructor to the class Student that sets...Ch. 8.2 - Rewrite the definition of the method writeoutput...
Ch. 8.2 - Rewrite the definition of the method reset for the...Ch. 8.2 - Can an object be referenced by variables of...Ch. 8.2 - What is the type or types of the variable(s) that...Ch. 8.2 - Prob. 14STQCh. 8.2 - Prob. 15STQCh. 8.2 - Consider the code below, which was discussed in...Ch. 8.2 - Prob. 17STQCh. 8.3 - Prob. 18STQCh. 8.3 - Prob. 19STQCh. 8.3 - Is overloading a method name an example of...Ch. 8.3 - In the following code, will the two invocations of...Ch. 8.3 - In the following code, which definition of...Ch. 8.4 - Prob. 23STQCh. 8.4 - Prob. 24STQCh. 8.4 - Prob. 25STQCh. 8.4 - Prob. 26STQCh. 8.4 - Prob. 27STQCh. 8.4 - Prob. 28STQCh. 8.4 - Are the two definitions of the constructors given...Ch. 8.4 - The private method skipSpaces appears in the...Ch. 8.4 - Describe the implementation of the method drawHere...Ch. 8.4 - Is the following valid if ShapeBaSe is defined as...Ch. 8.4 - Prob. 33STQCh. 8.5 - Prob. 34STQCh. 8.5 - What is an advantage of having the main...Ch. 8.5 - What Java construct allows us to define and...Ch. 8 - Consider a program that will keep track of the...Ch. 8 - Implement your base class for the hierarchy from...Ch. 8 - Draw a hierarchy for the components you might find...Ch. 8 - Suppose we want to implement a drawing program...Ch. 8 - Create a class Square derived from DrawableShape,...Ch. 8 - Create a class SchoolKid that is the base class...Ch. 8 - Derive a class ExaggeratingKid from SchoolKid, as...Ch. 8 - Create an abstract class PayCalculator that has an...Ch. 8 - Derive a class RegularPay from PayCalculator, as...Ch. 8 - Create an abstract class DiscountPolicy. It should...Ch. 8 - Derive a class BulkDiscount from DiscountPolicy,...Ch. 8 - Derive a class BuyNItemsGetOneFree from...Ch. 8 - Prob. 13ECh. 8 - Prob. 14ECh. 8 - Create an interface MessageEncoder that has a...Ch. 8 - Create a class SubstitutionCipher that implements...Ch. 8 - Create a class ShuffleCipher that implements the...Ch. 8 - Define a class named Employee whose objects are...Ch. 8 - Define a class named Doctor whose objects are...Ch. 8 - Create a base class called Vehicle that has the...Ch. 8 - Create a new class called Dog that is derived from...Ch. 8 - Define a class called Diamond that is derived from...Ch. 8 - Prob. 2PPCh. 8 - Prob. 3PPCh. 8 - Prob. 4PPCh. 8 - Create an interface MessageDecoder that has a...Ch. 8 - For this Programming Project, start with...Ch. 8 - Modify the Student class in Listing 8.2 so that it...Ch. 8 - Create a JavaFX application that uses a TextField...Ch. 8 - Prob. 10PP
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
On paper, write a program that will display your name on the first line; your street address on the second line...
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
T F Arguments are passed to the base class constructor by the derived class constructor.
Starting Out with C++ from Control Structures to Objects (8th Edition)
Explain the problems that denormalized tables may have for insert, update, and delete actions.
Database Concepts (7th Edition)
(Tabular Output) Write a program that utilizes looping to produce the following table of values:
C How to Program (8th Edition)
Repair Bill Suppose automobile repair customers are billed at the rate of per hour for labor. Also, suppose co...
Introduction To Programming Using Visual Basic (11th Edition)
Assume the following statement has been executed: number = 1234567.456 Write a Python statement that displays t...
Starting Out with Python (4th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // *** returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // *** move the center of the circle to the new coordinates +intersects(Circle other): bool //*** returns true if the center of the other circle lies inside this circle else returns false +resize(double…arrow_forwardThe Constructors with all the default arguments are similar as default constructors. True or Falsearrow_forwardThe Schedule class contains several courses. Each Course has a name, start time, and end time. The toString method for a Course should return a string containing the name, start time, and end time. For example, if the course is "Networking I" and it is held from 8am to 8:50am, the toString method for that course should return: "Networking I: 8am - 8:50am" The toString method for a Schedule should return "Your Schedule:" followed by all of the courses in the schedule. Below is an example showing what the Schedule's toString method should return if it contained five courses (Networking I, Database, Web Development, Java, and Yoga): "Your ScheduleNetworking I: 8am - 8:50amDatabase: 9am - 9:50amWeb Development: 10am - 10:50amJava I: 12pm - 12:50pmYoga: 1pm - 1:50pm" Include a Main.java to test your codearrow_forward
- True or False Constructors can accept arguments in the same way as other methods.arrow_forwardjavaarrow_forwardYou will create an Essay class that inherits from the GradedActivity class. The Essay class should determine the grade a student receives on an essay. The essay score can be up to 100 points and is determined by the following: Grammar: Spelling: Correct Length: Content: You do not need to do any verification of the number of points. All you will do in the Essay class is to add up the four points and send it to GradedActivity. Start with the program GradedActivity.java. You could modify FinalExam.java Naming: Name the Java project: LastFProjectAssign08 Name the driver program (main): LastFAssign08.java Where Last is your last name and F is the first initial of your first name. Ex: OgleD Call the essay class: Essay.java. Make sure you name, class, and date are comments in this file. Save in a file folder on your storage device. Sample output: What was the grammar score on the essay? 15What was the spelling score on the essay? 10What was the length score on the essay? 10What was…arrow_forward
- The Spider Game Introduction: In this assignment you will be implementing a game that simulates a spider hunting for food using python. The game is played on a varying size grid board. The player controls a spider. The spider, being a fast creature, moves in the pattern that emulates a knight from the game of chess. There is also an ant that slowly moves across the board, taking steps of one square in one of the eight directions. The spider's goal is to eat the ant by entering the square it currently occupies, at which point another ant begins moving across the board from a random starting location. Game Definition: The above Figure illustrates the game. The yellow box shows the location of the spider. The green box is the current location of the ant. The blue boxes are the possible moves the spider could make. The red arrow shows the direction that the ant is moving - which, in this case, is the horizontal X-direction. When the ant is eaten, a new ant is randomly placed on one of the…arrow_forwardA class object can encapsulate more than one [answer].arrow_forwardDrag and drop the options in the correct places. This is not a graded questionarrow_forward
- Java Assignment Outcomes: Student will demonstrate the ability to utilize inheritance in a Java program. Student will demonstrate the ability to apply the IS A and HAS A relationships. Program Specifications: Start by watching Video Segment 16 from Dr. Colin Archibald's video series (found in the module overview). Key in the program shown in the video and make sure it works. Then, add the following: Animals have a Weight. Animals have a Height. Dog is an Animal. Dogs have a Name. Dogs have a Breed. Dogs have a DOB. Cat is an Animal Cats have a Name. Cats have 9 lives, so you need to keep track of the remaining lives once a cat dies. Bird is an Animal Birds have a wing span Birds have a canFly which is true or false (some birds cannot fly) Create a test class that creates one of each type of animal and displays the animal’s toString method. Submission Requirements: You must follow the rules from the prior assignments. UMLs and Design Tools are not required for this one. YOU MAY…arrow_forward2. Car Class Write a class named car that has the following fields: ▪ yearModel. The year Model field is an int that holds the car's year model. ▪ make. The make field is a String object that holds the make of the car, such as "Ford", "Chevrolet", "Honda", etc. ▪ speed. The speed field is an int that holds the car's current speed. In addition, the class should have the following methods. ■ Constructor. The constructor should accept the car's year model and make as arguments. These values should be assigned to the object's year Model and make fields. The constructor should also assign 0 to the speed field.arrow_forwardThis is the tester of two classesarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
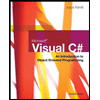
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY