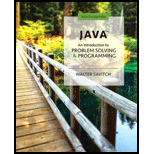
Create a new class called Dog that is derived from the Pet class given in Listing 6.1 of Chapter 6. The new class has the additional attributes of breed (type string) and boosterShot (type boolean), which is true if the pet has had its booster shot and false if not. Give your classes a reasonable complement of constructors and accessor methods. Write a driver

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Modern Database Management
Problem Solving with C++ (10th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Digital Fundamentals (11th Edition)
- Give source code and output alsoarrow_forwardCreate a base class called Insect. All insects belong to an Order [i.e. “Hemiptera” (ants), “Siphonaptera” (fleas), “Termitoidae” (termites), “Gryllidae” (crickets), etc.] and have a size that is measured in millimeters (use a double data type). Provide a default constructor that initializes the size to zero and outputs the message “Invoking the default Insect constructor” and another constructor that allows the Order and size to be set by the client. This other constructor should also output the message “Invoking the 2-argument Insect constructor.” Also create a destructor for this class that outputs the message “Invoking the default Insect destructor.” Your Insect class should have a function called Eat that cannot be implemented. That is, it should be declared as a purely virtual function. Your class should also have Get and Set methods to allow the order and size to be accessed.arrow_forwardYou are asked to write a discount system for a beauty saloon, which provides services and sells beauty products. It offers 3 types of memberships: Premium, Gold, and Silver. Premium, gold and silver members receive a discount of 20%, 15%, and 10%, respectively, for all services provided. Customers without membership receive no discount. All members receives a flat 10% discount on products purchased (this might change in the future). Your system shall consist of three classes: Customer, DiscountRate and Visit, as shown in the class diagram. It shall compute the total bill if a customer purchases $x of products and $y of services, for a visit. Also, write a test program VisitDriver (are not to be graded and optional) to exercise all the classes. Important: "Visit Class diagram - ERROR" Constructor method of Visit class must recieve Customer object instead of String name. Visit(Customer customer, Date date) The class DiscountRate contains only static variables and methods (underlined in…arrow_forward
- You are asked to write a discount system for a beauty saloon, which provides services and sells beauty products. It offers 3 types of memberships: Premium, Gold, and Silver. Premium, gold and silver members receive a discount of 20%, 15%, and 10%, respectively, for all services provided. Customers without membership receive no discount. All members receives a flat 10% discount on products purchased (this might change in the future). Your system shall consist of three classes: Customer, DiscountRate and Visit, as shown in the class diagram. It shall compute the total bill if a customer purchases $x of products and $y of services, for a visit. Also, write a test program VisitDriver (are not to be graded and optional) to exercise all the classes. Important: "Visit Class diagram - ERROR" Constructor method of Visit class must recieve Customer object instead of String name. Visit(Customer customer, Date date) The class DiscountRate contains only static variables and methods (underlined in…arrow_forwardIn python how do you: Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value. The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items. Ex: If the input is: M&M's 10.0 34.0 2.0 1.0 where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is: Nutritional information per serving of None:…arrow_forwardIN C++ Implement a class Car with the following properties. A car has a certain fuel efficiency (measured in miles/gallon or liters/km—pick one) and a certain amount of fuel in the gas tank. The efficiency is specified in the constructor, and the initial fuel level is 0. Provide another parameterized constructor which takes both efficiency and fuel as parameters. Also supply methods getGasInTank, returning the current amount of gasoline in the fuel tank. getEfficiency() return the efficiency of car. setGasInTank(double fuel), to add gasoline to the fuel tank. setEfficiency(double efficiency) Supply a method Drive () that simulates driving the car for a certain distance, reducing the amount of gasoline in the fuel tank according to its efficiency. Sample usage: Car myHybrid (50); // 50 miles per gallon myHybrid.addGas(20); // Tank 20 gallons myHybrid.Drive(100); // Drive 100 miles double gasLeft = myHybrid.getGasInTank(); // Get gas remaining in tank Supply a main method that tests…arrow_forward
- Help, I making a elevator simulator, I need help in writing code in the Elevator class and it's polymorphism class. I am not sure what to do next. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system:StandardElevator: This is the most common type of elevator and has a request…arrow_forwardCreate a Right Triangle class that has two sides. Name your class rightTraingle. Code getter and setters for the base and the height. (Remember class variables are private.) The class should include a two-argument constructor that allows the program to set the base and height. The constructor should verify that all the dimensions are greater than 0. before assigning the values to the private data members. If a side is not greater than zero, set the value to -1. The class also should include two value-returning methods. One value-returning method should calculate the area of a triangle, and the other should calculate the perimeter of a triangle. If either side is -1, these functions return a -1. The formula for calculating the area of a triangle is 1/2 * b*h, where b is the base and h is the height. The formula for calculating the perimeter of a triangle is b+h+sqrt (b*b+h*h). Be sure to include a default constructor that initializes the variables of the base, height to -1. To test…arrow_forwardNote: Write a Java program given below Question # 1: Define a class for Students with field name, age, marks. Define getter and setter for all the fields. In the setter method, if age is less than 0, print that Age is less than zero. Similarly, if marks is less than zero or greater than 100, print in correct marks and don’t assign marks to member variable. In the Test class, create student’s object and assign the marks and age to appropriate values. Try assigning incorrect values to check if getter and setter methods work as expected.arrow_forward
- Write a program that accepts two numbers. Use Scanner class to get the user input. Create 4 classes, the first one is the Main Class that has the main method, the other three classes are Su m, Subtract and Multiply. These three classes should have their constructors which has a parameter of type int. Create a method for each class that returns the result of the computation of two numbers. In the main method, after accepting the user input, it should call the three methods from the three classes and display its result.arrow_forwardDesign a class called "Person" with attributes for name, age, and gender. Implement methods to get and set these attributes, and also a method to calculate the person's age in dog years (assuming 1 dog year is equivalent to 7 human years).arrow_forwardGive Solutionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
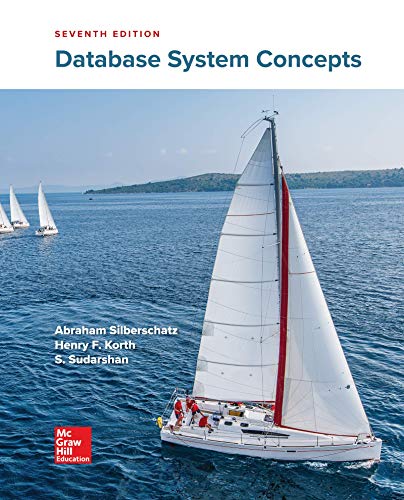
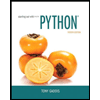
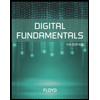
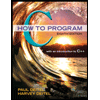
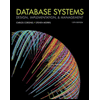
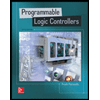