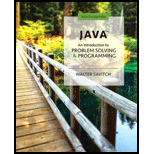
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 2PP
Program Plan Intro
Displaying right arrow and left arrow
Program Plan:
Filename: Test.java
- • Include the required header files.
- • Define the class “Test”.
- • Define main function.
- ○ Create an object for scanner.
- ○ Declare the character variable.
- ○ The “do-while” condition is used to get the user response to do the operation once again.
- ■ Create the objects “a1” and “b1” for “LeftArrow” and “RightArrow” classes.
- ■ Call the method “writeOutput” using both the objects.
- ■ Create the objects “a2” and “b2” for “LeftArrow” and “RightArrow” classes.
- ■ Call the method “writeOutput” using both the objects.
- ■ Create the objects “a3” and “b3” for “LeftArrow” and “RightArrow” classes.
- ■ Call the method “writeOutput” using both the objects.
- ■ Change the value of offset from 0 to 1 and call the method “writeOutput” using both the objects.
- ■ Call the method “setOffset” using both the objects.
- ■ Change the value of offset from 0 to 2 and call the method “writeOutput” using both the objects.
- ■ Call the method “setTail” using both the objects.
- ■ Change the value of offset from 3 to 10 and call the method “writeOutput” using both the objects.
- ■ Call the method “setBase” using both the objects.
- ■ Test the write offset values for both arrows by calling “writeOffset” method.
- ■ Test the return offset for both arrows by calling “getOffset” method.
- ■ Test the write tail for both arrows by calling “writeTail” method.
- ■ Test the return tail for both arrows by calling “getTail” method.
- ■ Test the write base for both arrows by calling “writeBase” method.
- ■ Test the return tail for both arrows by calling “getBase” method.
- ■ Reset the values and call “set” method.
- ■ Call the method “writeOutput” using both the objects.
- ■ Call the “drawHere” method using both the objects.
- ■ Reset the values and call “set” method.
- ■ Call the method “writeOutput” using both the objects.
- ■ Call the “drawHere” method using both the objects.
- ■ Reset the values and call “set” method.
- ■ Call the method “writeOutput” using both the objects.
- ■ Call the “drawHere” method using both the objects.
- ■ Finally display the two arrows on the output screen.
Filename: LeftArrow.java
- • Define the class “LeftArrow” extends from the “ShapeBase” class.
- ○ Declare the required variables.
- ○ Define the default constructor.
- ■ Call the “super” method.
- ■ Set the values.
- ○ Define the constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- • Assign “base” is equal to 3.
- ○ Define another constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super”.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- ■ Define another constructor with the parameters “theTail”, “theBase”.
- • Assign “base” is equal to 3.
- ○ Define the “set” method with the arguments “newOffset”, newTail”, and “newBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ○ Define the “setTail” method with an argument “newTail”.
- ■ Set the value.
- ○ Define the method “setBase” with an argument “newBase”.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ■ Check the “newBase” value is less than 3.
- ○ Define the “writeOutput()” method.
- ■ Call the “writeOffset()”, “writeTail ()”, and “writeBase()” methods.
- ○ Define the “writeOffset()” method.
- ■ Display the offset by calling the “getOffset()” method.
- ○ Define the “writeTail()” method.
- ■ Display the offset by calling the “getTail()” method.
- ○ Define the “writeBase()” method.
- ■ Display the offset by calling the “getBase()” method.
- ○ The method “getTail()” returns the “tail” value.
- ○ The method “getBase()” returns the “base” value.
- ○ Define the method “drawHere ()”.
- ■ Call the “drawTop()”, “drawTail()”, and “drawBottom()” methods.
- ○ Define the “drawTop ()” method.
- ■ Declare the variable “linecount” and assign the value of “getBase()” value divided by 2.
- ■ Declare the variable “numberOfSpaces” and calculate it.
- ■ Call the “skipSpaces()” method with a parameter “numberOfSpaces” value.
- ■ Display the “*” character.
- ■ Declare the “count” variable.
- ■ Declare the “insideWidth” with value 1.
- ■ The “for” condition is used to display the “*” character in left arrow shape.
- • Decrement the “numberOfSpaces” value by 2.
- • Call the “skipSpaces()” method with “numberOfSpaces” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “insideWidth” value.
- • Display the “*” character.
- • Increment the “insideWidth” value by 2.
- ○ Define the “drawTial()” method.
- ■ Call the “skipSpaces()” method with “getOffset()” value.
- ■ Display the “*” character.
- ■ Declare and calculate the “insideWidth” value.
- ■ Call the “skipSpaces()” method with “insideWidth” value.
- ■ Declare the “count” variable.
- ■ The “for” condition is used to display the “*” character in left arrow shape.
- • Display the “*” character.
- ○ Define the “drawBottom()” method.
- ■ Declare the required variables and calculate them respectively.
- ■ The “for” condition is used to display the “*” character in left arrow shape.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “inideWidth” value.
- • Display the “*” character.
- • Increment the “startOfLine” value by 2.
- • Decrement the “insideWidth” value by 2.
- ○ Define “skipSpaces()” method.
- ■ Display the space.
Filename: RightArrow.java
- • Define the class “RightArrow” extends from the “ShapeBase” class.
- ○ Declare the required variables.
- ○ Define the default constructor.
- ■ Call the “super” method.
- ■ Set the values.
- ○ Define the constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- • Assign “base” is equal to 3.
- ○ Define another constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super”.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- ■ Define another constructor with the parameters “theTail”, “theBase”.
- • Assign “base” is equal to 3.
- ○ Define the “set” method with the arguments “newOffset”, newTail”, and “newBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ○ Define the “setTail” method with an argument “newTail”.
- ■ Set the value.
- ○ Define the method “setBase” with an argument “newBase”.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ■ Check the “newBase” value is less than 3.
- ○ Define the “writeOutput()” method.
- ■ Call the “writeOffset()”, “writeTail ()”, and “writeBase()” methods.
- ○ Define the “writeOffset()” method.
- ■ Display the offset by calling the “getOffset()” method.
- ○ Define the “writeTail()” method.
- ■ Display the offset by calling the “getTail()” method.
- ○ Define the “writeBase()” method.
- ■ Display the offset by calling the “getBase()” method.
- ○ The method “getTail()” returns the “tail” value.
- ○ The method “getBase()” returns the “base” value.
- ○ Define the method “drawHere ()”.
- ■ Call the “drawTop()”, “drawTail()”, and “drawBottom()” methods.
- ○ Define the “drawTop ()” method.
- ■ Declare the variable “startOfLine” and assign the value of “getOffset()” plus “getTail()”.
- ■ Call the “skipSpaces()” method with a parameter “startOfLine” value.
- ■ Display the “*” character.
- ■ Declare the “linecount” and calculate it.
- ■ Declare the “count” variable.
- ■ Declare the “insideWidth” with value 1.
- ■ The “for” condition is used to display the “*” character in right arrow shape.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “insideWidth” value.
- • Display the “*” character.
- • Increment the “insideWidth” value by 2.
- ○ Define the “drawTial()” method.
- ■ Call the “skipSpaces()” method with “getOffset()” value.
- ■ Declare the “count” variable.
- ■ The “for” condition is used to display the “*” character in right arrow shape.
- • Display the “*” character.
- • Calculate the “insideWidth”.
- • Call the “skipSpaces()” method with “insideWidth” value.
- • Display the “*” character.
- ○ Define the “drawBottom()” method.
- ■ Declare the required variables and calculate them respectively.
- ■ The “for” condition is used to display the “*” character in right arrow shape.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “inideWidth” value.
- • Display the “*” character.
- • Decrement the “insideWidth” value by 2.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- ○ Define “skipSpaces()” method.
- ■ Display the space.
Filename: ShapeInterface.java
Define the interface “SpaceInterface”.
- • Declare the “setOffset()” method.
- • Declare the “getOffset()” method.
- • Declare the “drawAt()” method.
- • Declare the “drawHere()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Lab 07: Java Graphics
(Bonus lab)
In this lab, we'll be practicing what we learned about GUIs, and Mouse events. You will need to
implement the following:
➤ A GUI with a drawing panel. We can click in this panel, and you will capture those clicks as a
Point (see java.awt.Point) in a PointCollection class (you need to build this).
о The points need to be represented by circles.
Below the drawing panel, you will need 5 buttons:
о
An input button to register your mouse to the drawing panel.
○
о
о
A show button to paint the points in your collection on the drawing panel.
A button to shift all the points to the left by 50 pixels.
The x position of the points is not allowed to go below zero.
Another button to shift all the points to the right 50 pixels.
The x position of the points cannot go further than the
You can implement this GUI in any way you choose. I suggest using the BorderLayout for a panel
containing the buttons, and a GridLayout to hold the drawing panel and button panels.…
If a UDP datagram is sent from host A, port P to host B, port Q, but at host B there is no process listening to port Q, then B is to send back an ICMP Port Unreachable message to A. Like all ICMP messages, this is addressed to A as a whole, not to port P on A.
(a) Give an example of when an application might want to receive such ICMP messages.
(b) Find out what an application has to do, on the operating system of your choice, to receive such messages.
(c) Why might it not be a good idea to send such messages directly back to the originating port P on A?
Discuss how business intelligence and data visualization work together to help decision-makers and data users. Provide 2 specific use cases.
Chapter 8 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 8.1 - Prob. 1STQCh. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Can a derived class directly access by name a...Ch. 8.1 - Can a derived class directly invoke a private...Ch. 8.1 - Prob. 6STQCh. 8.1 - Suppose s is an object of the class Student. Base...Ch. 8.2 - Give a complete definition of a class called...Ch. 8.2 - Add a constructor to the class Student that sets...Ch. 8.2 - Rewrite the definition of the method writeoutput...
Ch. 8.2 - Rewrite the definition of the method reset for the...Ch. 8.2 - Can an object be referenced by variables of...Ch. 8.2 - What is the type or types of the variable(s) that...Ch. 8.2 - Prob. 14STQCh. 8.2 - Prob. 15STQCh. 8.2 - Consider the code below, which was discussed in...Ch. 8.2 - Prob. 17STQCh. 8.3 - Prob. 18STQCh. 8.3 - Prob. 19STQCh. 8.3 - Is overloading a method name an example of...Ch. 8.3 - In the following code, will the two invocations of...Ch. 8.3 - In the following code, which definition of...Ch. 8.4 - Prob. 23STQCh. 8.4 - Prob. 24STQCh. 8.4 - Prob. 25STQCh. 8.4 - Prob. 26STQCh. 8.4 - Prob. 27STQCh. 8.4 - Prob. 28STQCh. 8.4 - Are the two definitions of the constructors given...Ch. 8.4 - The private method skipSpaces appears in the...Ch. 8.4 - Describe the implementation of the method drawHere...Ch. 8.4 - Is the following valid if ShapeBaSe is defined as...Ch. 8.4 - Prob. 33STQCh. 8.5 - Prob. 34STQCh. 8.5 - What is an advantage of having the main...Ch. 8.5 - What Java construct allows us to define and...Ch. 8 - Consider a program that will keep track of the...Ch. 8 - Implement your base class for the hierarchy from...Ch. 8 - Draw a hierarchy for the components you might find...Ch. 8 - Suppose we want to implement a drawing program...Ch. 8 - Create a class Square derived from DrawableShape,...Ch. 8 - Create a class SchoolKid that is the base class...Ch. 8 - Derive a class ExaggeratingKid from SchoolKid, as...Ch. 8 - Create an abstract class PayCalculator that has an...Ch. 8 - Derive a class RegularPay from PayCalculator, as...Ch. 8 - Create an abstract class DiscountPolicy. It should...Ch. 8 - Derive a class BulkDiscount from DiscountPolicy,...Ch. 8 - Derive a class BuyNItemsGetOneFree from...Ch. 8 - Prob. 13ECh. 8 - Prob. 14ECh. 8 - Create an interface MessageEncoder that has a...Ch. 8 - Create a class SubstitutionCipher that implements...Ch. 8 - Create a class ShuffleCipher that implements the...Ch. 8 - Define a class named Employee whose objects are...Ch. 8 - Define a class named Doctor whose objects are...Ch. 8 - Create a base class called Vehicle that has the...Ch. 8 - Create a new class called Dog that is derived from...Ch. 8 - Define a class called Diamond that is derived from...Ch. 8 - Prob. 2PPCh. 8 - Prob. 3PPCh. 8 - Prob. 4PPCh. 8 - Create an interface MessageDecoder that has a...Ch. 8 - For this Programming Project, start with...Ch. 8 - Modify the Student class in Listing 8.2 so that it...Ch. 8 - Create a JavaFX application that uses a TextField...Ch. 8 - Prob. 10PP
Knowledge Booster
Similar questions
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
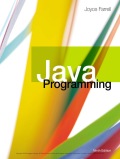
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
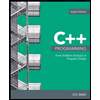
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
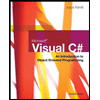
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
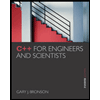
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
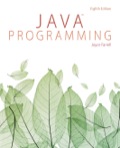
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT