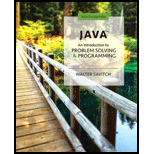
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 8, Problem 6E
Create a class SchoolKid that is the base class for children at a school. It should have attributes for the child’s name and age the name of the child’s teacher, and a greeting. It should have appropriate accessor and mutator methods for each of the attributes.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Could you help me to know features of the following concepts:
- defragmenting.
- dynamic disk.
- hardware RAID
Chapter 8 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 8.1 - Prob. 1STQCh. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Can a derived class directly access by name a...Ch. 8.1 - Can a derived class directly invoke a private...Ch. 8.1 - Prob. 6STQCh. 8.1 - Suppose s is an object of the class Student. Base...Ch. 8.2 - Give a complete definition of a class called...Ch. 8.2 - Add a constructor to the class Student that sets...Ch. 8.2 - Rewrite the definition of the method writeoutput...
Ch. 8.2 - Rewrite the definition of the method reset for the...Ch. 8.2 - Can an object be referenced by variables of...Ch. 8.2 - What is the type or types of the variable(s) that...Ch. 8.2 - Prob. 14STQCh. 8.2 - Prob. 15STQCh. 8.2 - Consider the code below, which was discussed in...Ch. 8.2 - Prob. 17STQCh. 8.3 - Prob. 18STQCh. 8.3 - Prob. 19STQCh. 8.3 - Is overloading a method name an example of...Ch. 8.3 - In the following code, will the two invocations of...Ch. 8.3 - In the following code, which definition of...Ch. 8.4 - Prob. 23STQCh. 8.4 - Prob. 24STQCh. 8.4 - Prob. 25STQCh. 8.4 - Prob. 26STQCh. 8.4 - Prob. 27STQCh. 8.4 - Prob. 28STQCh. 8.4 - Are the two definitions of the constructors given...Ch. 8.4 - The private method skipSpaces appears in the...Ch. 8.4 - Describe the implementation of the method drawHere...Ch. 8.4 - Is the following valid if ShapeBaSe is defined as...Ch. 8.4 - Prob. 33STQCh. 8.5 - Prob. 34STQCh. 8.5 - What is an advantage of having the main...Ch. 8.5 - What Java construct allows us to define and...Ch. 8 - Consider a program that will keep track of the...Ch. 8 - Implement your base class for the hierarchy from...Ch. 8 - Draw a hierarchy for the components you might find...Ch. 8 - Suppose we want to implement a drawing program...Ch. 8 - Create a class Square derived from DrawableShape,...Ch. 8 - Create a class SchoolKid that is the base class...Ch. 8 - Derive a class ExaggeratingKid from SchoolKid, as...Ch. 8 - Create an abstract class PayCalculator that has an...Ch. 8 - Derive a class RegularPay from PayCalculator, as...Ch. 8 - Create an abstract class DiscountPolicy. It should...Ch. 8 - Derive a class BulkDiscount from DiscountPolicy,...Ch. 8 - Derive a class BuyNItemsGetOneFree from...Ch. 8 - Prob. 13ECh. 8 - Prob. 14ECh. 8 - Create an interface MessageEncoder that has a...Ch. 8 - Create a class SubstitutionCipher that implements...Ch. 8 - Create a class ShuffleCipher that implements the...Ch. 8 - Define a class named Employee whose objects are...Ch. 8 - Define a class named Doctor whose objects are...Ch. 8 - Create a base class called Vehicle that has the...Ch. 8 - Create a new class called Dog that is derived from...Ch. 8 - Define a class called Diamond that is derived from...Ch. 8 - Prob. 2PPCh. 8 - Prob. 3PPCh. 8 - Prob. 4PPCh. 8 - Create an interface MessageDecoder that has a...Ch. 8 - For this Programming Project, start with...Ch. 8 - Modify the Student class in Listing 8.2 so that it...Ch. 8 - Create a JavaFX application that uses a TextField...Ch. 8 - Prob. 10PP
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
TestScores Modification for Serialization Modify the TestScores class that you created for Programming Challeng...
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Create variable names that would be appropriate for holding each of the following information items: a. The num...
Starting Out With Visual Basic (8th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
1.2 Explain the difference between geodetic and plane
surveys,
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Define each of the following terms: supertype subtype specialization entity cluster completeness constraint enh...
Modern Database Management
Explain how controlled movements of the work m three mutually perpendicular directions are obtained m column-an...
Degarmo's Materials And Processes In Manufacturing
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
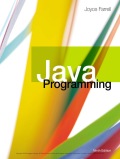
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
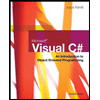
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
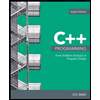
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
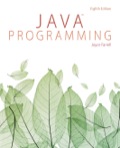
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
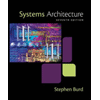
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY