STARTING OUT WITH C++ MPL
9th Edition
ISBN: 9780136673989
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 7, Problem 48RQE
Write a remove member function that accepts an argument for a number of units and removes that number of units of an item from inventory. If the operation is completed successfully it should return the number of units remaining in stock for that item. However, if the number of units passed to the function is less than the number of units in stock, it should not make the removal and should return - 1 as an error signal.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Suppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet.
What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?
R language
Using R language
Chapter 7 Solutions
STARTING OUT WITH C++ MPL
Ch. 7.5 - Which of the following shows the correct use of...Ch. 7.5 - An objects private member variables can be...Ch. 7.5 - Assuming that soap is an instance of the Inventory...Ch. 7.5 - Complete the following code skeleton to declare a...Ch. 7.7 - Briefly describe the purpose of a constructor.Ch. 7.7 - Constructor functions have the same name as the A)...Ch. 7.7 - A constructor that requires no arguments is called...Ch. 7.7 - Assume the following is a constructor: ClassAct: :...Ch. 7.7 - Prob. 7.9CPCh. 7.7 - True or false: A class may have a constructor with...
Ch. 7.7 - A destructor function name always starts with A) a...Ch. 7.7 - True or false: Just as a class can have multiple...Ch. 7.7 - What will the following program code display on...Ch. 7.7 - What will the following program code display on...Ch. 7.9 - 7.15 private class member function can be called...Ch. 7.9 - When an object is passed to a function, a copy of...Ch. 7.9 - If a function receives an object as an argument...Ch. 7.9 - Prob. 7.18CPCh. 7.9 - Prob. 7.19CPCh. 7.10 - Prob. 7.20CPCh. 7.10 - Write a class declaration for a class named...Ch. 7.10 - Write a class declaration for a class named Pizza...Ch. 7.10 - Write four lines of code that might appear in a...Ch. 7.11 - Assume the following class components exist in a...Ch. 7.11 - What header files should be included in the client...Ch. 7.12 - Write a structure declaration for a structure...Ch. 7.12 - Prob. 7.27CPCh. 7.12 - Prob. 7.28CPCh. 7.12 - Write a declaration for a structure named...Ch. 7.12 - Write a declaration for a structure named City,...Ch. 7.12 - Write assignment statements that store the...Ch. 7.12 - Prob. 7.32CPCh. 7.12 - Write a function that uses a Rectangle structure...Ch. 7.12 - Prob. 7.34CPCh. 7.15 - Prob. 7.35CPCh. 7.15 - When designing an object -oriented application,...Ch. 7.15 - How do you identify the potential classes in a...Ch. 7.15 - What two questions should you ask to determine a...Ch. 7.15 - Look at the following description of a problem...Ch. 7 - Prob. 1RQECh. 7 - Which of the following must a programmer know...Ch. 7 - Prob. 3RQECh. 7 - ______programming is centered around functions, or...Ch. 7 - An object is a software entity that combines both...Ch. 7 - An object is a(n) ______ of a class.Ch. 7 - Prob. 7RQECh. 7 - Once a class is declared, how many objects can be...Ch. 7 - An objects data items are stored in its...Ch. 7 - The procedures, or functions, an object performs...Ch. 7 - Bundling together an objects data and procedures...Ch. 7 - An objects members can be declared public or...Ch. 7 - Normally a classs _________ are declared to be...Ch. 7 - A class member function that uses, but does not...Ch. 7 - A class member function that changes the value of...Ch. 7 - When a member functions body is written inside a...Ch. 7 - A class constructor is a member function with the...Ch. 7 - A constructor is automatically called when an...Ch. 7 - Constructors cannot have a(n) ______ type.Ch. 7 - A(n) ______ constructor is one that requires no...Ch. 7 - A destructor is a member function that is...Ch. 7 - A destructor has the same name as the class but is...Ch. 7 - A constructor whose parameters all have default...Ch. 7 - A class may have more than one constructor, as...Ch. 7 - Prob. 25RQECh. 7 - In general, it is considered good practice to have...Ch. 7 - When a member (unction forms part of the interface...Ch. 7 - When a member function performs a task internal to...Ch. 7 - True or false: A class object can be passed to a...Ch. 7 - Prob. 30RQECh. 7 - It is considered good programming practice to...Ch. 7 - If you were writing a class declaration for a...Ch. 7 - If you were writing the definitions for the Canine...Ch. 7 - A structure is like a class but normally only...Ch. 7 - By default, are the members of a structure public...Ch. 7 - Prob. 36RQECh. 7 - When a structure variable is created its members...Ch. 7 - Prob. 38RQECh. 7 - Prob. 39RQECh. 7 - Prob. 40RQECh. 7 - Prob. 41RQECh. 7 - Write a function called showReading. It should...Ch. 7 - Write a function called input Reading that has a...Ch. 7 - Write a function called getReading, which returns...Ch. 7 - Indicate whether each of the following enumerated...Ch. 7 - Prob. 46RQECh. 7 - Assume a class named Inventory keeps track of...Ch. 7 - Write a remove member function that accepts an...Ch. 7 - Prob. 49RQECh. 7 - A) struct TwoVals { int a, b; } ; int main() { }...Ch. 7 - A) struct Names { string first; string last; } ;...Ch. 7 - A) class Circle: { private double centerX; double...Ch. 7 - A) class DumbBell; { int weight; public: void set...Ch. 7 - If the items on the following list appeared in a...Ch. 7 - Look at the following description of a problem...Ch. 7 - Soft Skills Working in a team can often help...Ch. 7 - Date Design a class called Date that has integer...Ch. 7 - Report Heading Design a class called Heading that...Ch. 7 - Widget Factory Design a class for a widget...Ch. 7 - Car Class Write a class named Car that has the...Ch. 7 - Population In a population, the birth rate and...Ch. 7 - Gratuity Calculator Design a Tips class that...Ch. 7 - Inventory Class Design an Inventory class that can...Ch. 7 - Movie Data Write a program that uses a structure...Ch. 7 - Movie Profit Modify the Movie Data program written...Ch. 7 - Prob. 10PCCh. 7 - Prob. 11PCCh. 7 - Ups and Downs Write a program that displays the...Ch. 7 - Wrapping Ups and Downs Modify the program you...Ch. 7 - Left and Right Modify the program you wrote for...Ch. 7 - Moving Inchworm Write a program that displays an...Ch. 7 - Coin Toss Simulator Write a class named Coin. The...Ch. 7 - Tossing Coins for a Dollar Create a game program...Ch. 7 - Fishing Came Simulation Write a program that...Ch. 7 - Group Project 19. Patient Fees This program should...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What is the difference between the names defined in an ML let construct from the variables declared in a C bloc...
Concepts Of Programming Languages
In the following exercises, write a program to carry out the task. The program should use variables for each of...
Introduction To Programming Using Visual Basic (11th Edition)
ICA 17-24
The decay of a radioactive isotope can be theoretically modeled with the following equation, where C0...
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Give an example of each of the following, other than those described in this chapter, and clearly explain why y...
Modern Database Management
The job of the _____ is to fetch instructions, carry out the operations commanded by the instructions, and prod...
Starting Out With Visual Basic (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Compare the security services provided by a digital signature (DS) with those of a message authentication code (MAC). Assume that Oscar can observe all messages sent between Rina and Naseem. Oscar has no knowledge of any keys but the public one, in the case of DS. State whether DS and MAC protect against each attack and, if they do, how. The value auth(x) is computed with a DS or a MAC algorithm. In each scenario, assume the message M = x#####auth(x). (Message integrity) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message M, and then sends M in cleartext to Naseem. Oscar intercepts the message and replaces “Mark” with “Oscar.” Will Naseem detect this in the case of either DS or MAC? If yes, how will Naseem detect it? If not, why? (Replay) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message…arrow_forwardI need to resolve the following....You are trying to convince your boss that your company needs to invest in a license for MS-Project (project management software from Microsoft) before beginning a systems project. What arguments would you give her?arrow_forwardWhat are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forward
- I would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forwardWhat is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward
- 3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forward
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
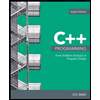
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
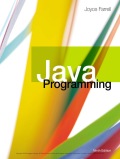
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
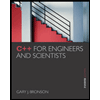
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
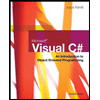
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
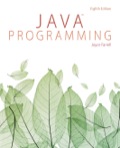
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY