Concept explainers
Coin Toss Simulator
Write a class named Coin. The Coin class should have the following member variable:
• A string named sideUp. The sideUp member variable will hold either “heads” or “tails” indicating the side of the coin that is facing up.
The Co1 n class should have the following member functions:
• A default constructor that randomly determines the side of the coin that is facing up (“heads” or “tails”) and initializes the sideUp member variable accordingly.
• A void member function named toss that simulates the tossing of the coin. When the toss member function is called, it randomly determines the side of the coin that is facing up (“heads” or “tails”) and sets the sideUp member variable accordingly.
• A member function named getSideUp that returns the value of the sideUp member variable.
Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
C++ How to Program (10th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
- Circle Class (Easy) Write a Circle class that has the following member variables: radius : a double The class should have the following member functions: Default Constructor: default constructor that sets radius to 0.0. Constructor: accepts the radius of the circle as an argument. setRadius: an mutator function for the radius variable. getRadius: an accessor function for the radius variable. getArea: returns the area of the circle, which is calculated as area = pi * radius * radius getCircumference: returns the circumference of the circle, which is calculated as circumference = 2 * pi * radius Step1: Create a declaration of the class. Step2: Write a program that demonstrates the Circle class by asking the user for the circle’s radius, creating Circle objects, and then reporting the circle’s area, and circumference. You should create at least two circle objects, one sets the radius to 0.0 and one accepts the radius as an…arrow_forwardWe use the _____ operator to create an instance of (object in) a particular class.arrow_forwardTrue or false: A class may have a constructor with no parameter list, and an overloaded constructor whose parameters all take default arguments.arrow_forward
- Problem: Employee and ProductionWorker Classes Write a python class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: • Shift number (an integer, such as 1, 2, or 3)• Hourly pay rateThe workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for this class. Once you have written the class, write a program that creates an object of the ProductionWorker class, and prompts the user to enter data for each of the object’s data attributes. Store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen Note: The program should be written in python. Sample Input/Output: Enter the name: Ahmed Al-AliEnter the ID number: 12345Enter the department:…arrow_forwardThe class implementation: The Account class) Design a class named Account that contains: A private int data field named id for the account (default 0). A private double data field named balance for the account (default 0). A private double data field named annuallnterestRate that stores the urrent interest rate (default 0). Assume all accounts have the same interestarrow_forwardRemaining Time: 35 minutes, 18 seconds, ¥ Question Completion Status: A class named Account is defined as the following. class Account } private: int id; double balance; public: //A constructor without parameter that creates a default account with id 0, balance 0 Account): /A constructor with the parameter that setting the id and balance Account(int, double), int getId); / return the ID double getBalance() //return the balance void withdraw(double amount) // the amount will be withdrawn void setlID(int), //set the ID void setBalance (double) //set the balance }: Assume that we will create a separate file of implementation of the function definition for the questions a) -b) a) Write a construction function definition with two parameters (ID and balance). b) Write a function definition of the function declaration (void withdraw(double amount):). c) Creates an Account object with an ID (1122) and balance ($20000). Then, write source codes to withdraw $2 MacBooarrow_forward
- In order to use the assignment operator on an instance of a class data type, it must be overloaded. Group of answer choices True Falsearrow_forwardThere are two types of data members in a class: static and non-static. Provide an example of when it might be useful to have a static data member in the actual world.arrow_forwardemployee and production worker classes write an employee class that keeps data attributes for the following pieces of information: • employee name • employee number next, write a class named productionworker that is a subclass of the employee class. the productionworker class should keep data attributes for the following information: • shift number (an integer, such as 1, 2, or 3) • hourly pay rate the workday is divided into two shifts: day and night. the shift attribute will hold an integer value representing the shift that the employee works. the day shift is shift 1 and the night shift is shift 2. write the appropriate accessor and mutator methods for each class. once you have written the classes, write a program that creates an object of the productionworker class and prompts the user to enter data for each of the object’s data attributes. store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen. satak overfallowarrow_forward
- Car Class in C++ language whole task is in the picturearrow_forwardngineering Computer ScienceQ&A Libraryin c++ Create a class time24, each object is a value represented the time of day in the form hours, minutes and seconds. Provide a constructor that enables an object of this class to be initialized when it is instantiated. The constructor should contain default values in case no initializes are provided. Provide Public member functions for each of the following operations: set the time, print the time, increment the time by one second, compare two times for equality, increment the time by one hour, determine if one time is “less than” (comes before) another time, and final print the time in format 12 hours. Include any additional operations that you think would be useful for your class. Design, implement, and test your class. in c++ Create a class time24, each object is a value represented the time of day in the form hours, minutes and seconds. Provide a constructor that enables an object of this class to be initialized when it is…arrow_forwardC++arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
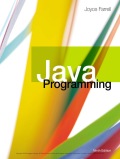
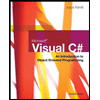
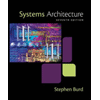