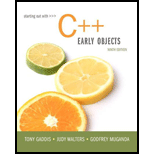
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 7, Problem 1RQE
Program Description Answer
ADT means “Abstract Data Type”.
Expert Solution & Answer

Explanation of Solution
Abstract Data type:
ADT stands for Abstract Data type.
An abstract data type is a data type which holds value and the operations are performed on them without specifying the details of how the data type is implemented. In other words, it is a collection of data and related operations for using the data.
- It supports abstraction, encapsulation, and data hiding.
- The programmer is responsible for defining a set of values that the data type can hold and also to define a set of operations performed on the data type.
- It extracts and combines the common functionalities form various related object into one package.
- Generally in C++ and other object oriented
programming languages, the programmer created ADT’s are implemented as class.- Class – A class is a collection of data members and member functions, which are used to represent the overall structure of the project.
- Object – The objects are created to access the data members and member functions of the class.
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
What exactly are compound operators?
What is const keyword?
What are operators, exactly?
Chapter 7 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 7.5 - Which of the following shows the correct use of...Ch. 7.5 - An objects private member variables can be...Ch. 7.5 - Assuming that soap is an instance of the Inventory...Ch. 7.5 - Complete the following code skeleton to declare a...Ch. 7.7 - Briefly describe the purpose of a constructor.Ch. 7.7 - Constructor functions have the same name as the A)...Ch. 7.7 - A constructor that requires no arguments is called...Ch. 7.7 - Assume the following is a constructor: ClassAct: :...Ch. 7.7 - Prob. 7.9CPCh. 7.7 - True or false: A class may have a constructor with...
Ch. 7.7 - A destructor function name always starts with A) a...Ch. 7.7 - True or false: Just as a class can have multiple...Ch. 7.7 - What will the following program code display on...Ch. 7.7 - What will the following program code display on...Ch. 7.9 - 7.15 private class member function can be called...Ch. 7.9 - When an object is passed to a function, a copy of...Ch. 7.9 - If a function receives an object as an argument...Ch. 7.9 - Prob. 7.18CPCh. 7.9 - Prob. 7.19CPCh. 7.10 - Prob. 7.20CPCh. 7.10 - Write a class declaration for a class named...Ch. 7.10 - Write a class declaration for a class named Pizza...Ch. 7.10 - Write four lines of code that might appear in a...Ch. 7.11 - Assume the following class components exist in a...Ch. 7.11 - What header files should be included in the client...Ch. 7.12 - Write a structure declaration for a structure...Ch. 7.12 - Prob. 7.27CPCh. 7.12 - Prob. 7.28CPCh. 7.12 - Write a declaration for a structure named...Ch. 7.12 - Write a declaration for a structure named City,...Ch. 7.12 - Write assignment statements that store the...Ch. 7.12 - Prob. 7.32CPCh. 7.12 - Write a function that uses a Rectangle structure...Ch. 7.12 - Prob. 7.34CPCh. 7.15 - Prob. 7.35CPCh. 7.15 - When designing an object -oriented application,...Ch. 7.15 - How do you identify the potential classes in a...Ch. 7.15 - What two questions should you ask to determine a...Ch. 7.15 - Look at the following description of a problem...Ch. 7 - Prob. 1RQECh. 7 - Which of the following must a programmer know...Ch. 7 - Prob. 3RQECh. 7 - ______programming is centered around functions, or...Ch. 7 - An object is a software entity that combines both...Ch. 7 - An object is a(n) ______ of a class.Ch. 7 - Prob. 7RQECh. 7 - Once a class is declared, how many objects can be...Ch. 7 - An objects data items are stored in its...Ch. 7 - The procedures, or functions, an object performs...Ch. 7 - Bundling together an objects data and procedures...Ch. 7 - An objects members can be declared public or...Ch. 7 - Normally a classs _________ are declared to be...Ch. 7 - A class member function that uses, but does not...Ch. 7 - A class member function that changes the value of...Ch. 7 - When a member functions body is written inside a...Ch. 7 - A class constructor is a member function with the...Ch. 7 - A constructor is automatically called when an...Ch. 7 - Constructors cannot have a(n) ______ type.Ch. 7 - A(n) ______ constructor is one that requires no...Ch. 7 - A destructor is a member function that is...Ch. 7 - A destructor has the same name as the class but is...Ch. 7 - A constructor whose parameters all have default...Ch. 7 - A class may have more than one constructor, as...Ch. 7 - Prob. 25RQECh. 7 - In general, it is considered good practice to have...Ch. 7 - When a member (unction forms part of the interface...Ch. 7 - When a member function performs a task internal to...Ch. 7 - True or false: A class object can be passed to a...Ch. 7 - Prob. 30RQECh. 7 - It is considered good programming practice to...Ch. 7 - If you were writing a class declaration for a...Ch. 7 - If you were writing the definitions for the Canine...Ch. 7 - A structure is like a class but normally only...Ch. 7 - By default, are the members of a structure public...Ch. 7 - Prob. 36RQECh. 7 - When a structure variable is created its members...Ch. 7 - Prob. 38RQECh. 7 - Prob. 39RQECh. 7 - Prob. 40RQECh. 7 - Prob. 41RQECh. 7 - Write a function called showReading. It should...Ch. 7 - Write a function called input Reading that has a...Ch. 7 - Write a function called getReading, which returns...Ch. 7 - Indicate whether each of the following enumerated...Ch. 7 - Prob. 46RQECh. 7 - Assume a class named Inventory keeps track of...Ch. 7 - Write a remove member function that accepts an...Ch. 7 - Prob. 49RQECh. 7 - A) struct TwoVals { int a, b; } ; int main() { }...Ch. 7 - A) struct Names { string first; string last; } ;...Ch. 7 - A) class Circle: { private double centerX; double...Ch. 7 - A) class DumbBell; { int weight; public: void set...Ch. 7 - If the items on the following list appeared in a...Ch. 7 - Look at the following description of a problem...Ch. 7 - Soft Skills Working in a team can often help...Ch. 7 - Date Design a class called Date that has integer...Ch. 7 - Report Heading Design a class called Heading that...Ch. 7 - Widget Factory Design a class for a widget...Ch. 7 - Car Class Write a class named Car that has the...Ch. 7 - Population In a population, the birth rate and...Ch. 7 - Gratuity Calculator Design a Tips class that...Ch. 7 - Inventory Class Design an Inventory class that can...Ch. 7 - Movie Data Write a program that uses a structure...Ch. 7 - Movie Profit Modify the Movie Data program written...Ch. 7 - Prob. 10PCCh. 7 - Prob. 11PCCh. 7 - Ups and Downs Write a program that displays the...Ch. 7 - Wrapping Ups and Downs Modify the program you...Ch. 7 - Left and Right Modify the program you wrote for...Ch. 7 - Moving Inchworm Write a program that displays an...Ch. 7 - Coin Toss Simulator Write a class named Coin. The...Ch. 7 - Tossing Coins for a Dollar Create a game program...Ch. 7 - Fishing Came Simulation Write a program that...Ch. 7 - Group Project 19. Patient Fees This program should...
Knowledge Booster
Similar questions
- Whats the simplified expression?arrow_forwardWhat exactly are logical operators?arrow_forwardLanguage : C Jojo remembered going to a cave with his best friend Lili before the coronavirus pandemic.There, he saw several Stalactites hanging from the /cave’s ceiling. Stalactite is a type offormation that hangs from the ceiling of caves.Jojo noticed an interesting fact about the stalactites. The stalactite formation in thiscave forms a pattern. He wonders how will the formation look like if the cave is of adifferent size. He has asked your help to visualize this formation. Format Input :A single line with an integer N denoting the size of the cave Format Output :A size N formation of stalactites. Do not print extra spaces behind the last ‘*’ character in each line. Constraints : • 1 ≤ N ≤ 12 Sample Input 1 :2 Sample Output 1*** *Sample Input 2 :3 Sample Output 2 :******* * * * *arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
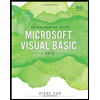
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning