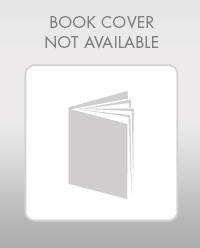
Concept explainers
The following
#include <iostream>
using namespace std;
void func1(int &, int &) ;
void func2(int &, int &, int &);
void func3(int, int, int);
int main()
{
int x = 0, y = 0, z = 0;
cout << x << " " << y << z << endl;
func1(x, y);
cout << x << " " << y << z << endl;
func2(x, y, z);
cout << x << " " << y << z << endl;
func3(x, y, z);
cout << x << " " << y << z << endl;
return 0;
}
void func1(int &a, int &b)
{ cout << "Enter two numbers: ";
cin >> a >> b;
}
void func2(int &a, int &b, int &c)
{ b++;
c - -;
a = b + c;
}
void func3(int a, int b, int c)
{ a = b + c;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Modern Database Management
Management Information Systems: Managing The Digital Firm (16th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Mechanics of Materials (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- C++ Visual 2019 A particular talent competition has five judges, each of whom awards a score between 0 and 10 to each performer. Fractional scores, such as 8.3, are allowed. A performer's final score is determined by dropping the highest and lowest score received, then averaging the three remaining scores. Write a program that uses this method to calculate a contestant's score. It should include the following functions: void getJudgeData() should ask the user for a judge's score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five judges. void calcScore() should calculate and display the average of the three scores that remain after dropping the highest and lowest scores the performer received. This function should be called just once by main and should be passed the five scores. The last two functions, described below, should be called by calcScore, which uses the returned information to determine which of the…arrow_forwardStatic Variable: a variable whose lifetime is the lifetime of the program (static int x;) Dynamic Variable: It is a pointer to a variable (int *x;) Is this comparison true?arrow_forwardLowest Score Drop Write a program that calculates the average of a group of test scores, where the lowest score in the group is dropped. It should use the following functions: void getScore() should ask the user for a test score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five scores to be entered. void calcAverage() should calculate and display the average of the four highest scores. This function should be called just once by main and should be passed the five scores. int findLowest() should find and return the lowest of the five scores passed to it. It should be called by calcAverage, which uses the function to determine which of the five scores to drop. Input Validation: Do not accept test scores lower than 0 or higher than 100.arrow_forward
- // FixedDebugFive04// Program prompts user for any number of values// (up to 20)// and averages them// C# Programusing System;public class DebugFive04{public static void Main(){int[] numbers = new int[20];const int QUIT = 999;int x;int num;double average;double total = 0;Console.Write("Please enter a number or " +QUIT + " to quit...");num = Convert.ToInt32(Console.ReadLine());x = 0;while ((x < numbers.Length) && num != QUIT){numbers[x] = num;total += numbers[x];Console.Write("Please enter a number or " +QUIT + " to quit...");num = Convert.ToInt32(Console.ReadLine());x++;}average = total / x;Console.WriteLine("The average is {0}", average);Console.WriteLine("The numbers are:");for (int y = 0; y < x; ++y)Console.Write("{0,6}", numbers[y]);}}arrow_forwardNeeds to be done in C# language. Problem Write a program that computes and displays the charges for a patient’s hospital stay. First, the program should ask if the patient was admitted as an in-patient or an out-patient. If the patient was an in-patient, the following data should be entered: • The number of days spent in the hospital• The daily rate• Hospital medication charges• Charges for hospital services (lab tests, etc.) The program should ask for the following data if the patient was an out-patient: • Charges for hospital services (lab tests, etc.)• Hospital medication charges The program should use two overloaded functions to calculate the total charges. One of the functions should accept arguments for the in-patient data, while the other function accepts arguments for out-patient information. Both functions should return the total charges. Input Validation: Do not accept negative numbers for any data.arrow_forwardusing namespace std; int main () { int x = 3; if (!x) if (x = 4) cout << "AAA"; else cout << "BBB"; return 0; BBB AAA None of the above Run-time errorarrow_forward
- C++ code not Javaarrow_forwardQuestion #3: Write a C program that repeatedly asks the user to enter real numbers from the keyboard then it calculates and prints the average of the entered numbers. The program continuously asks the user till the user responds by 'N'. #include int main(void) { //Declare required variables //code for reading real numbers continuously till the user responds by 'N' //code for calculating the average of the entered numbers and printing it printf("\n"); printf("\n"); return 0; } Question3.c Output Screenshotarrow_forward#include #include void main(void) {int number; Cout > number; if ( number > 100) cout <<" number is greater then 100" ; else cout <" number is not greater than 100" ;arrow_forward
- C++ beginnerarrow_forwardI assume the following 2 codes express the same meaning? int myvar; int * myptr = &myvar; and int myvar; int * myptr; myptr = &myvar;arrow_forwardQ 6:- Specify the local and Global variables used in the code below and express the reason r'. #include int a=10,b; void main() { printf("a = 8d and b=%d“,a,b) ; #include void main() { int x-23, y-4; printf("x = %d and y=sd“,x,y);arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage