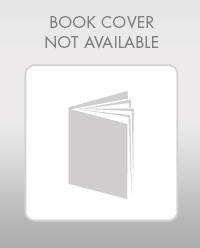
Explanation of Solution
Output of the Program:
The given program has been added with comments and line number.
/*Include required variables*/
#include <iostream> //Line 1
using namespace std; //Line 2
/*Function prototype*/
void myFunc();//Line 3
/*Main function*/
int main()//Line 4
{//Line 5
/*Variable "var" is declared as "int" data type and it is assigned with the value "100"*/
int var = 100; //Line 6
/*Print the value*/
cout << var << endl; //Line 7
/*Call the function "myFunc()"*/
myFunc();//Line 8
/*Display the value of variable "val" after calling the function*/
cout << var << endl; //Line 9
/*Return the value 0*/
return 0; //Line 10
}//Line 11
/* Function definition */
void myFunc()//Line 12
{//Line 13
/*Variable "var" is declared as "int" data type and it is assigned with the value "50"*/
int var = 50; //Line 14
/* Print the value of the variable "var" */
cout << var << endl; //Line 15
}//Line 16
Explanation:
The above program explains the scope of the local variable...

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out With C++: Early Objects (10th Edition)
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- The assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forwardhi I would like to get help to resolve the following casearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
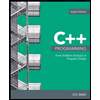
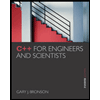
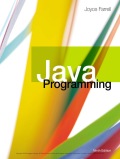