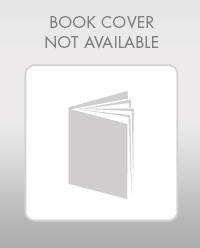
Explanation of Solution
Output of the Program:
The given program has been added with comments and line number.
/* Include required variables */
#include <iostream> //Line 1
using namespace std; //Line 2
/* Function prototype */
void showVar(); //Line 3
/* Main function */
int main()//Line 4
{ //Line 5
/* Loop till "count" variable is less than "10" */
for (int count = 0; count < 10; count++) //Line 6
/*Call the function "showVar()"*/
showVar(); //Line 7
/*Return the value 0*/
return 0; //Line 8
}//Line 9
/*Function definition*/
void showVar() //Line 10
{//Line 11
/* Variable "var" is declared as static "int" and assign "10" to it */
static int var = 10; //Line 12
/*Print the value "var"*/
cout << var << endl; //Line 13
/*Increment the variable "var"*/
var++; //Line 14
} //Line 15
Explanation:
The above program explains the scope of the local variable when it is declared as “static”.
“main()” function:
- In line 6, it uses the “for” loop for iterating the “count” variable equals to less than 10.
- When the value of “count” is “0”, the function “showVar()” has been called, which prints the value 10.
- When the value of “count” is “1”, the function “showVar()” has been called, which prints the value 11.
- When the value of “count” is “2”, the function “showVar()” has been called, which prints the value 12...

Trending nowThis is a popular solution!

Chapter 6 Solutions
Starting Out With C++: Early Objects (10th Edition)
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
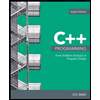
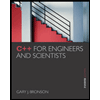
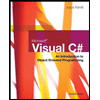
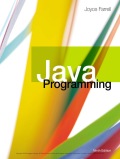