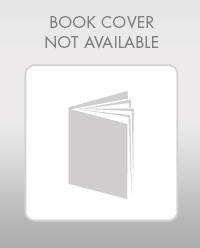
Star Search
A particular talent competition has five judges, each of whom awards a score between 0 and 10 to each performer. Fractional scores, such as 8.3, are allowed. A performer’s final score is determined by dropping the highest and lowest score received, then averaging the three remaining scores. Write a
• void getJudgeData() should ask the user for a judge’s score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five judges.
• double calcScore() should calculate and return the average of the three scores that remain after dropping the highest and lowest scores the performer received. This function should be called just once by main and should be passed the five scores.
Two additional functions, described below, should be called by calcScore, which uses the returned information to determine which of the scores to drop.
• int findLowest() should find and return the lowest of the five scores passed to it.
• int findHighest() should find and return the highest of the five scores passed to it.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Problem Solving with C++ (9th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
C Programming Language
Web Development and Design Foundations with HTML5 (8th Edition)
- (Numerical) Write and test a function that returns the position of the largest and smallest values in an array of double-precision numbers.arrow_forwardDomino's Time Function Name: dominosTime() Parameters: N/A Returns: None Description: During the summer, you ordered a lot of food from Domino's. Pizzas are $12, an order of pasta is $6, and chicken wings are $8. Write a function that asks the user how many of each food item they would like, and then print a response telling them what their order to- tal will be. The order total should be an integer. >>> dominosTime() How many pizzas do you want? 3 How many orders of pasta do you want? 2 How many orders of chicken wings do you want? 2 By ordering 3 pizzas, 2 orders of pasta, and 2 orders of chicken wings, your order total comes to $64.arrow_forwardUsernames An online company needs your help to implement a program that verifies the username chosen by a new user. Their rules is described below: Username MUST contain at least 6 characters; Username cannot start with a number; Username can only contain letters or numbers. If valid, the username may be resgistered if it doesn't already exist in the system. You should not use built-in functions to determine the character type such as isnumeric() or islower(). Use the strings given alphabet and numeric to determine if each character is valid. Use the list registered to help you determine if the username is already registered. Don't forget to execute the cell below to use these strings # run this cell to create these variablesalphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'numeric = "0123456789"registered = ["john87", "topmage", "light4ever", "username2"] Write a function nameValidation which receives a String argument name. This function: has name String…arrow_forward
- Usernames An online company needs your help to implement a program that verifies the username chosen by a new user. Their rules is described below: Username MUST contain at least 6 characters; Username cannot start with a number; Username can only contain letters or numbers. If valid, the username may be resgistered if it doesn't already exist in the system. You should not use built-in functions to determine the character type such as isnumeric() or islower(). Use the strings given alphabet and numeric to determine if each character is valid. Use the list registered to help you determine if the username is already registered. Don't forget to execute the cell below to use these strings # run this cell to create these variablesalphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'numeric = "0123456789"registered = ["john87", "topmage", "light4ever", "username2"]arrow_forwardUsernames An online company needs your help to implement a program that verifies the username chosen by a new user. Their rules is described below: Username MUST contain at least 6 characters; Username cannot start with a number; Username can only contain letters or numbers. If valid, the username may be resgistered if it doesn't already exist in the system. You should not use built-in functions to determine the character type such as isnumeric() or islower(). Use the strings given alphabet and numeric to determine if each character is valid. Use the list registered to help you determine if the username is already registered. Don't forget to execute the cell below to use these strings # run this cell to create these variablesalphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'numeric = "0123456789"registered = ["john87", "topmage", "light4ever", "username2"] Write a function registerName which receives a String username and it returns a string message. This…arrow_forwardC codearrow_forward
- FizzBuzz Interview Question Create a function that takes a number as an argument and returns "Fizz", "Buzz" or "FizzBuzz". If the number is a multiple of 3 the output should be "Fizz". If the number given is a multiple of 5, the output should be "Buzz". If the number given is a multiple of both 3 and 5, the output should be "FizzBuzz". If the number is not a multiple of either 3 or 5, the number should be output on its own as shown in the examples below. The output should always be a string even if it is not a multiple of 3 or 5. Examples fizz_buzz (3) "Fizz" fizz_buzz (5) → "Buzz fizz_buzz (15) "FizzBuzz" fizz_buzz (4) "4" 11arrow_forwardTracking laps Learning Objectives In this lab, you will practice writing functions, passing arguments and returning results from the function printing the result of a function call writing your code as a module Instructions Main Idea An Olympic-size swimming pool is used in the Olympic Games, where the racecourse is 50 meters (164.0 ft) in length. "In swimming, a lap is the same as a length. By definition, a lap means a complete trip around a race track, in swimming, the pool is the race track. Therefore if you swim from one end to the other, you’ve completed the track and thus you’ve completed one lap or one length." (Source: What Is A Lap In Swimming? Lap Vs Length) Write the function meters_to_laps() that takes a number of meters as an argument and returns the real number of laps. Complete the program to output the number of laps with two digits after the period. Examples Input: 150 Output : 3.00 Input: 80 Output: 1.60 Your program must define and call the following…arrow_forwardLISP Function help please LISP Programming only A function that generates a random day of the week, then displays a message saying that "Today is ... and tomorrow will be ...". Then use the built-in function random first to generate a number between 0 and 6 (including). The expression (random) by itself generates a random integer. You can call it with one parameter to return a value within the range from 0 to the value of the parameter-1. For example, (random 10) will return a value between 0 and 9. Next, use the number generated at the previous step to retrieve the symbol for the day of the week from the list. Use the built-in elt. Extract the symbol-name of the day first, then apply the built-in function capitalize to it. Use the result in the princ function call, and do the same thing for the next day. Make the function return true (t) instead of the last thing it evaluates, to avoid seeing the message printed more than once.arrow_forward
- Void functions do not return any value when they are called.True or falsearrow_forwardPlants Growth Cycle Learning Objectives In this lab, you will practice: Defining a function to match the given specifications Calling the function in your program Using if statements (can combine them with dictionaries) Instructions For every plant, there is a growth cycle. The number of days that it takes starting from being a seed and ending in being a fruit is what is called the growth cycle. Write a function that takes a plant's name as an argument and returns its growth cycle (in days). In your program: Input from the user the name of a plant Check if the input is either "strawberry", "cucumber" or "potato", if Yes: 2.1 Call calculate_growth_cycle 2.2. In function calculate_growth_cycle, check over the plant's name: 2.2.1 If strawberry, print "### The life cycle of a strawberry ###" and return 110 2.2.2 If cucumber, print "### The life cycle of a cucumber ###" and return 76 2.2.3 If potato, print "### The life cycle a potato ###" and return 120 2.3 With the growth cycle…arrow_forwardJAVA:arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
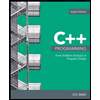
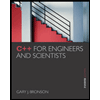