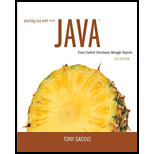
Concept explainers
Payroll Class
Design a Payroll class that has fields for an employee’s name, ID number, hourly pay rate, and number of hours worked. Write the appropriate accessor and mutator methods and a constructor that accepts the employee’s name and ID number as arguments. The class should also have a method that returns the employee’s gross pay, which is calculated as the number of hours worked multiplied by the hourly pay rate. Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Starting Out With Visual Basic (7th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with C++: Early Objects
- Lab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardjava Assignment Description: Write a class named Car that has the following fields: The yearModel field is an int that holds the car’s year model. The make field references a String object that holds the make of the car. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods. The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Constructor: The constructor should accept no arguments. Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed. Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and…arrow_forwardRemaining Time: 35 minutes, 18 seconds, ¥ Question Completion Status: A class named Account is defined as the following. class Account } private: int id; double balance; public: //A constructor without parameter that creates a default account with id 0, balance 0 Account): /A constructor with the parameter that setting the id and balance Account(int, double), int getId); / return the ID double getBalance() //return the balance void withdraw(double amount) // the amount will be withdrawn void setlID(int), //set the ID void setBalance (double) //set the balance }: Assume that we will create a separate file of implementation of the function definition for the questions a) -b) a) Write a construction function definition with two parameters (ID and balance). b) Write a function definition of the function declaration (void withdraw(double amount):). c) Creates an Account object with an ID (1122) and balance ($20000). Then, write source codes to withdraw $2 MacBooarrow_forward
- Question - Create a class named Pizza with the following data fields: description - of type String price - of type double The description stores the type of pizza (such as sausage and onion). Include a constructor that requires arguments for both fields and a method named display to display the data. For example, if the description is 'sausage and onion' and the price is '14.99', the display method should output: sausage and onion pizza Price: $14.99 Create a subclass named DeliveryPizza that inherits from Pizza but adds the following data fields: deliveryFee - of type double address - of type String The description, price, and delivery address are required as arguments to the constructor. The delivery fee is $3 if the pizza ordered costs more than $15; otherwise it is $5. Code that is not accepted - class DeliveryPizza extends Pizza { private double deliveryFee; private String address; public DeliveryPizza(String desc, double price, double deliveryFee, String address){…arrow_forwardbasic java onlyarrow_forwardAssignment 10: Chapter 10 Complete Programming Challenge 10 at the end of chapter 10 10. Ship, CruiseShip, and CargoShip Classes Design a Ship class that the following members: A field for the name of the ship (a string) - A field for the year that the ship was built (a string). A constructor and appropriate accessors and mutators. A toString method that displays the ship's name and the year it was built. Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members: A field for the maximum number of passengers (an int). A constructor and appropriate accessors and mutators. A toString method that overrides the toString method in the base class. The CruiseShip class's toString method should display only the ship's name and the maximum number of passengers. Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members: A field for the cargo capacity in tonnage (an int). A constructor and…arrow_forward
- Design a class that holds the following personal data: name address age phone numberWrite appropriate accessor and mutator methods. Also, create a program that creates three instances of the class. One instance should hold yourinformation, and the other two should hold your friends’ or family members’information.arrow_forwardTrue or False It is legal to write a class without any constructors.arrow_forwardCar ClassWrite a class named Car that has the following fields:• yearModel. The yearModel field is an int that holds the car’s year model.• make. The make field references a String object that holds the make of the car.• speed. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods.• Constructor. The constructor should accept the car’s year model and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field.• Accessors. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and speed fields.• accelerate. The accelerate method should add 5 to the speed field each time it is called.• brake. The brake method should subtract 5 from the speed field each time it is called.Demonstrate the class in a program that creates a Car object, and then calls the accelerate method five…arrow_forward
- T/F: Instance variables are shared by all the instances of the class. T/F: The scope of instance and static variables is the entire class. They can be declared anywhere inside a class. T/F: To declare static variables, constants, and methods, use the static modifier.arrow_forwardParent Class: Food Write a parent class called Food. A food is described by a name, the number of grams of sugar (as a whole number), and the number of grams of sodium (as a whole number). Core Class Components For the Food class, write: the complete class header the instance data variables a constructor that sets the instance data variables based on parameters getters and setters; use validity checking on the parameters where appropriate a toString method that returns a text representation of a Food object that includes all three characteristics of the food Class-Specific Method Write a method that calculates what percent of the daily recommended amount of sugar is contained in a food. The daily recommended amount might change, so the method takes in the daily allowance and then calculates the percentage. For example, let's say a food had 6 grams of sugar. If the daily allowance was 24 grams, the percent would be 0.25. For that same food, if the daily allowance was 36 grams, the…arrow_forward7)Circle Class Write a Circle class that has the following fields: radius: a double PI:a final double initialized with the value 3.14159 The class should have the following methods: Constructor. Accepts the radius of the circles as an argument. Constructor. A no-arg constructor that sets the radius field to 0.0. setRadius. A mutator method for the radius field. getRadius.An accessor method for the radius field. area. Returns the area of the circle, which is calculated as area. Returns the area of the circles, which is calculated as area= PI * radius * radius diameter. Returns the diameter of the cirlce, which is calculated as diameter = radius * 2 circumference. Returns the circumference of the circle, which is calculated as circumference = 2 * PI * radius Write a program that demonstrates the Circle class by asking the user for the circles's radius, creating a Circle object, and then reporting the cirlce's area, diameter, and circumference.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
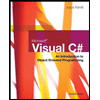
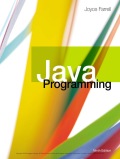
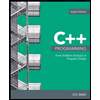
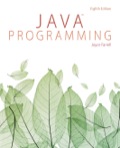