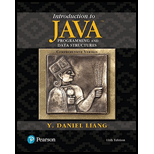
Suppose the input is 2 3 4 5 0. What is the output of the following code?
import java.util.Scanner;
public class Test {
public static void main(String[] args) {
Scanner input= new Scanner(System.in);
int number, sum= 0, count;
for (count = 0; count < 5; count++){
number= input.nextInt();
sum += number;
}
System.out.println("sum is "+ sum);
System.out.println("count is " + count);
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Degarmo's Materials And Processes In Manufacturing
Starting Out with Python (4th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forward
- You are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forwardConsider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forward
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
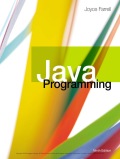
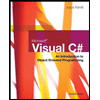
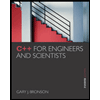
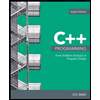
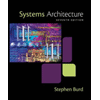