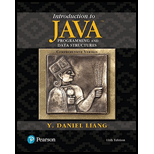
Concept explainers
(Count positive and negative numbers and compute the average of numbers) Write a
Enter an integer, the input ends if it is 0: 1 2 -1 3 0
The number of positives is 3
The number of negatives is 1
The total is 5.0
The average is 1.25
Enter an integer, the input ends if it is 0: 0
No numbers are entered except 0

Count positive and negative numbers and compute the average of numbers
Program Plan:
- Include the required import statement.
- Define the class
- Define the main() method using public static main.
- Declare and initialize the required variables.
- Declare the input scanner.
- Read an input from the user.
- Using while loop, check whether the integer is “0” or not
- Check whether the integer is greater than “0”.
- If so, increment the positive counter.
- Check whether the integer is less than “0”.
- If so, increment the negative counter.
- Calculate the sum of integers.
- Read the next input.
- Check whether the integer is greater than “0”.
- Display the sum and average of integers.
- Define the main() method using public static main.
The below program is used to count number of positives and number of negatives which are presented as inputs and finally calculate its sum and average as follows:
Explanation of Solution
Program:
//import statement
import java.util.Scanner;
//class Excersise_1
public class Excersise_1 {
// main function
public static void main(String[] args) {
// declare and initialize the required variables
int count_Positive = 0, count_Negative = 0;
int counter = 0, sum = 0, integer;
// declare the input scanner
Scanner in = new Scanner(System.in);
// print the instruction
System.out.print("Enter an integer, the input ends if it is 0: ");
// read the integer value from user
integer = in.nextInt();
// using while loop, check the integer
while (integer != 0) {
// check if it is positive
if (integer > 0)
/* if so, increment the positive counter */
count_Positive++;
// check if it is negative
else if (integer < 0)
/* if so, increment the negative counter */
count_Negative++;
// calculate the total of integer
sum += integer;
// increment the counter
counter++;
// Read the next integer
integer = in.nextInt();
}
// check the counter is 0
if (counter == 0)
// if so, no inputs are read
System.out.println("No numbers are entered except 0");
else {
// print the number of positive integers
System.out.println("The number of positives is " + count_Positive);
// print the number of negative integers
System.out.println("The number of negatives is " + count_Negative);
// print the sum
System.out.println("The total is " + sum);
// print the overall average
System.out.println("The average is " + sum * 1.0 / counter);
}
}
}
Enter an integer, the input ends if it is 0: 1
2
-1
3
0
The number of positives is 3
The number of negatives is 1
The total is 5
The average is 1.25
Want to see more full solutions like this?
Chapter 5 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Electric Circuits. (11th Edition)
Concepts Of Programming Languages
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
SURVEY OF OPERATING SYSTEMS
Problem Solving with C++ (10th Edition)
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardBased on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward
- 23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forwardYou are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forward
- Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
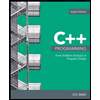
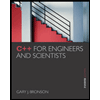
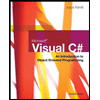
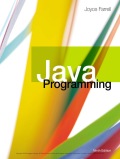
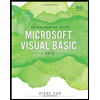