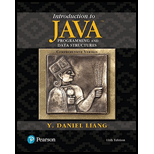
Concept explainers
(Compute e) You can approximate e using the following summation:
Write a
Initialize e and item to be 1, and keep adding a new item to e. The new item is the previous item divided by i, for i >= 2.)

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- (IN C LANGUAGE) Cumulative Addition: Computer selects a number between 7 and 23 at random. User will only add 2, 3 or 5 numbers to reach that number. For example: To reach 14:User will enter 5 5 2 2 (4 input).Also he can enter 2 2 2 2 2 2 2 (7 input) or 3 3 3 3 2 (5 input)arrow_forward(Sum the digits in an integer) Write a program that reads an integer between 0 and 1000 and adds all the digits in the integer. For example, if an integer is 932, the sum of all its digits is 14.arrow_forward(Geometry: distance of two points) Write a program that prompts the user to enter two points (x1, y1) and (x2, y2) and displays their distance between them. The formula for computing the distance is: Square root of ((x2 - x1) squared + (y2 - y1) squared) Note that you can use pow(a, 0.5) to compute square root of a. Sample Run Enter x1 and y1: 1.5 -3.4 Enter x2 and y2: 4 5 The distance between the two points is 8.764131445842194arrow_forward
- (Display Magic Numbers) Display the first N magic numbers, where N is a positive number that the user provides as input. Here, a magic number is a number whose sum of its digits eventually leads to 1. For example, 1234 is a magic number because 1 + 2 + 3 + 4 = 10 and 1 + 0 = 1, while 1235 is not (1 + 2 + 3 + 5 = 11 and 1 + 1 = 2). Write a program that prints out the first N magic numbers, seven on each line. Here is the sample output: You are required to use the following function prototype: bool isMagic(int value); // Returns true if value is a magic number The outline of this function will be as follows: Step 1: Calculate the sum of digits of value Step 2: Repeat Step 1 until we get a single digit Step 3: If the resulting sum is equal to 1 then it is a magic number, otherwise notarrow_forward(Display Magic Numbers) Display the first N magic numbers, where N is a positive number that the user provides as input. Here, a magic number is a number whose sum of its digits eventually leads to 1. For example, 1234 is a magic number because 1+2+3+4 = 10 and 1 +0 = 1, while 1235 is not (1 +2+ 3 +5 = 11 and 1 +1 = 2). Write a program that prints out the first N magic numbers, seven on each line. Here is the sample output: Enter a positive integer number: 30 1 10 19 28 37 46 55 64 73 82 91 100 109 118 127 136 145 154 163 172 181 190 199 208 217 226 235 244 253 262 You are required to use the following function prototype: bool isMagic(int value); // Returns true if value is a magic number The outline of this function will be as follows: Step 1: Calculate the sum of digits of value Step 2: Repeat Step 1 until we get a single digit Step 3: If the resulting sum is equal to 1 then it is a magic number, otherwise notarrow_forward(Demonstrate cancellation errors) A cancellation error occurs when you are manipulating a very large number with a very small number. The large number may cancel out the smaller number. For example, the result of 100000000.0 + 0.000000001 is equal to 100000000.0. To avoid cancellation errors and obtain more accurate results, carefully select the order of computation. For example, in computing the following series, you will obtain more accurate results by comput- ing from right to left rather than from left to right: 1 1 1 1+ + 3 + ... 2 п Write a program that compares the results of the summation of the preceding series, computing from left to right and from right to left with n = 50000.arrow_forward
- (Bar-Chart Printing Program) One interesting application of computers is drawing graphsand bar charts. Write a program that reads five numbers (each between 1 and 30). For each numberread, your program should print a line containing that number of adjacent asterisks. For example,if your program reads the number seven, it should print *******.arrow_forward(IN C LANGUAGE) Binary-Decimal / Decimal Binary . Between 0 and 255 a number will be decided randomly by computer. Then asks to user 3 times a random digit of binary value of the that number. If user enters wrong number for a digit program will select another random number and ask random times random digit. For example: Computer selected number as 163 ( Which is 10100011) What is the digit 2 (question 1/3) :User enter 1 CorrectWhat is the digit 4 (question 2/3) : User enter 0 CorrectWhat is the digit 7 (question 3/3) : User enter 0 CorrectUser finished the quest with 3 input .arrow_forward(Geometry: area of a regular polygon) A regular polygon is an n-sided polygon in which all sides are of the same length and all angles have the same degree (i.e., the polygon is both equilateral and equiangular). The formula for computing the area of a regular polygon is n x s? Area 4 X tan Here, s is the length of a side. Write a program that prompts the user to enter the number of sides and their length of a regular polygon and displays its area. Here is a sample run:arrow_forward
- (Count positive and negative numbers and compute the average of numbers) Write a program that reads an unspecified number of integers, determines how many positive and negative values have been read, and computes the total and average of the input values (not counting zeros). Your program ends with the input 0. Display the maximum, minimum, and average as a floating-point number. Here is a sample run:Enter an integer, the input ends if it is 0: 1 2 -1 3 0The number of positives is 3The number of negatives is 1The maximum is 3.0The minimum is -1.0The average is 1.25arrow_forward(Algebra: solve 2 * 2 linear equations) A linear equation can be solved using Cramer’s rule given in Programming Exercise 1.13. Write a program that prompts the user to enter a, b, c, d, e, and f and displays the result. If ad - bc is 0, report that “The equation has no solution.”arrow_forward( MindTap - Cenage )Example 5-6 implements the Number Guessing Game program. If the guessed number is not correct, the program outputs a message indicating whether the guess is low or high. Modify the program as follows: Suppose that the variables num and guess are as declared in Example 5-6 and diff is an int variable. Let diff = the absolute value of (num - guess). If diff is 0, then guess is correct and the program outputs a message indicating that the user guessed the correct number. Suppose diff is not 0. Then the program outputs the message as follows: If diff is greater than or equal to 50, the program outputs the message indicating that the guess is very high (if guess is greater than num) or very low (if guess is less than num). If diff is greater than or equal to 30 and less than 50, the program outputs the message indicating that the guess is high (if guess is greater than num) or low (if guess is less than num). If diff is greater than or equal to 15 and less than 30, the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
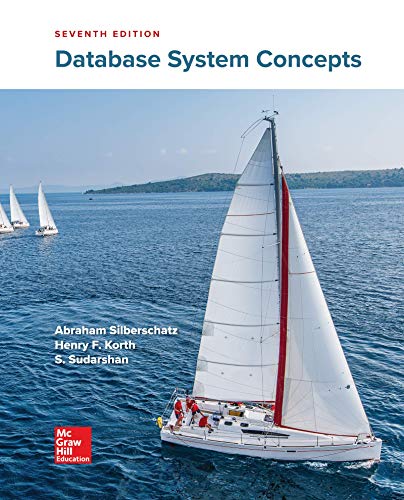
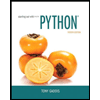
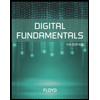
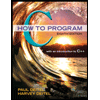
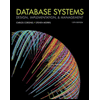
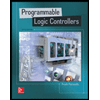