import java.util.Scanner; public class CircleAndSphereWhileLoop { public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and sphere volume on separate // lines with 6 decimal digits // Sample output // The radius : 499.990000. // The circle circumference: 3141.529822. // The circle area : 785366.747785. // The sphere surface area : 3141466.991100. // The sphere volume : 523567360.300077. } }
import java.util.Scanner;
public class CircleAndSphereWhileLoop
{
public static final double MAX_RADIUS = 500.0;
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
// Step 2: Read a double value as radius using prompt
// "Enter the radius (between 0.0 and 500.0, exclusive): "
// Step 3: While the input radius is not in the ragne (0.0, 500.0)
// Display a message on one line (ssuming input value -1)
// "The input number -1.00 is out of range."
// Read a double value as radius using the same promt
double circumference = 2 * Math.PI * radius;
double area = Math.PI * radius * radius;
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3);
// Step 4: Display the radius, circle circumference, circle area,
// sphere surface area, and sphere volume on separate
// lines with 6 decimal digits
// Sample output
// The radius : 499.990000.
// The circle circumference: 3141.529822.
// The circle area : 785366.747785.
// The sphere surface area : 3141466.991100.
// The sphere volume : 523567360.300077.
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

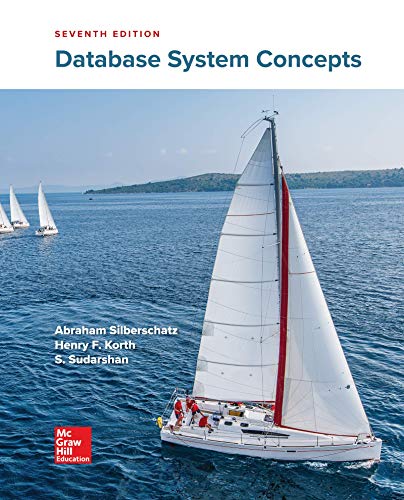
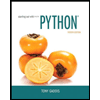
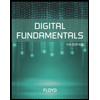
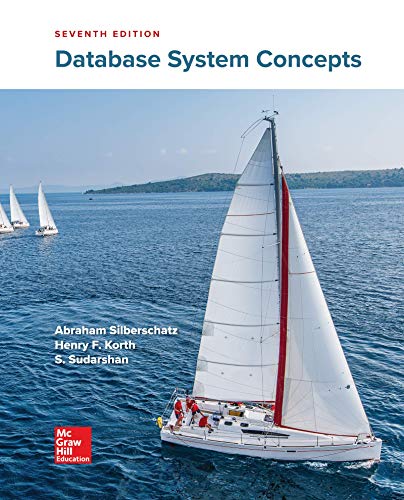
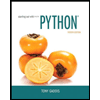
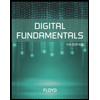
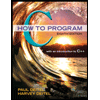
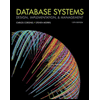
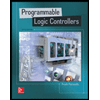