import java.util.Scanner; import java.io.FileInputStream; import java.io.FileOutputStream; import java.text.DecimalFormat; import java.io.IOException; public class LabProgram { public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); // Initialize variables for calculating exam averages int count = 0; double sumMid1 = 0, sumMid2 = 0, sumFinal = 0; // Initialize FileOutputStream for output FileOutputStream fos = new FileOutputStream("report.txt"); // Prompt for the filename System.out.print("Enter the name of the TSV file: "); String fileName = scnr.nextLine(); FileInputStream fis = null; try { fis = new FileInputStream(fileName); // Read the file and build the content string StringBuilder sb = new StringBuilder(); int ch; while ((ch = fis.read()) != -1) { sb.append((char) ch); } // Split the content by lines String[] lines = sb.toString().split("\n"); // Process each line for (String line : lines) { // Make sure the line contains sufficient data if (line.isEmpty()) { continue; } String[] tokens = line.trim().split("\t"); // Check the number of tokens if (tokens.length < 5) { System.out.println("Skipping invalid line: " + line); continue; } try { // Read student information String lastName = tokens[0]; String firstName = tokens[1]; int mid1 = Integer.parseInt(tokens[2].trim()); int mid2 = Integer.parseInt(tokens[3].trim()); int finalExam = Integer.parseInt(tokens[4].trim()); // Calculate the average and grade double average = (mid1 + mid2 + finalExam) / 3.0; String grade; if (average >= 90) grade = "A"; else if (average >= 80) grade = "B"; else if (average >= 70) grade = "C"; else if (average >= 60) grade = "D"; else grade = "F"; // Create report string String reportString = lastName + "\t" + firstName + "\t" + mid1 + "\t" + mid2 + "\t" + finalExam + "\t" + grade + "\n"; // Write to report.txt using FileOutputStream fos.write(reportString.getBytes()); // Update exam sum and count for average calculation sumMid1 += mid1; sumMid2 += mid2; sumFinal += finalExam; count++; } catch (NumberFormatException e) { System.out.println("Skipping invalid line with incorrect number format: " + line); } } // Calculate and write the averages to the file DecimalFormat df = new DecimalFormat("##.00"); String averageString = "Averages:\tMidterm1 " + df.format(sumMid1 / count) + ",\tMidterm2 " + df.format(sumMid2 / count) + ",\tFinal " + df.format(sumFinal / count); fos.write(averageString.getBytes()); } finally { // Close the FileInputStream and FileOutputStream if (fis != null) { fis.close(); } if (fos != null) { fos.close(); } } }
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.text.DecimalFormat;
import java.io.IOException;
public class LabProgram {
public static void main(String[] args) throws IOException {
Scanner scnr = new Scanner(System.in);
// Initialize variables for calculating exam averages
int count = 0;
double sumMid1 = 0, sumMid2 = 0, sumFinal = 0;
// Initialize FileOutputStream for output
FileOutputStream fos = new FileOutputStream("report.txt");
// Prompt for the filename
System.out.print("Enter the name of the TSV file: ");
String fileName = scnr.nextLine();
FileInputStream fis = null;
try {
fis = new FileInputStream(fileName);
// Read the file and build the content string
StringBuilder sb = new StringBuilder();
int ch;
while ((ch = fis.read()) != -1) {
sb.append((char) ch);
}
// Split the content by lines
String[] lines = sb.toString().split("\n");
// Process each line
for (String line : lines) {
// Make sure the line contains sufficient data
if (line.isEmpty()) {
continue;
}
String[] tokens = line.trim().split("\t");
// Check the number of tokens
if (tokens.length < 5) {
System.out.println("Skipping invalid line: " + line);
continue;
}
try {
// Read student information
String lastName = tokens[0];
String firstName = tokens[1];
int mid1 = Integer.parseInt(tokens[2].trim());
int mid2 = Integer.parseInt(tokens[3].trim());
int finalExam = Integer.parseInt(tokens[4].trim());
// Calculate the average and grade
double average = (mid1 + mid2 + finalExam) / 3.0;
String grade;
if (average >= 90) grade = "A";
else if (average >= 80) grade = "B";
else if (average >= 70) grade = "C";
else if (average >= 60) grade = "D";
else grade = "F";
// Create report string
String reportString = lastName + "\t" + firstName + "\t" + mid1 + "\t" + mid2 + "\t" + finalExam + "\t" + grade + "\n";
// Write to report.txt using FileOutputStream
fos.write(reportString.getBytes());
// Update exam sum and count for average calculation
sumMid1 += mid1;
sumMid2 += mid2;
sumFinal += finalExam;
count++;
} catch (NumberFormatException e) {
System.out.println("Skipping invalid line with incorrect number format: " + line);
}
}
// Calculate and write the averages to the file
DecimalFormat df = new DecimalFormat("##.00");
String averageString = "Averages:\tMidterm1 " + df.format(sumMid1 / count) + ",\tMidterm2 " + df.format(sumMid2 / count) + ",\tFinal " + df.format(sumFinal / count);
fos.write(averageString.getBytes());
} finally {
// Close the FileInputStream and FileOutputStream
if (fis != null) {
fis.close();
}
if (fos != null) {
fos.close();
}
}
}
}


Step by step
Solved in 4 steps with 1 images

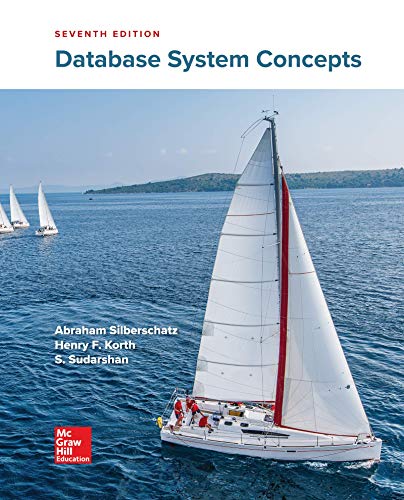
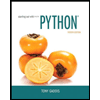
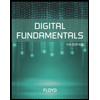
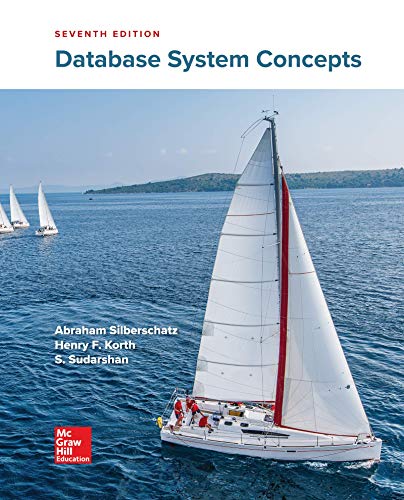
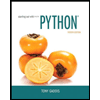
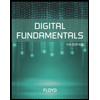
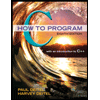
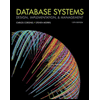
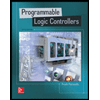